How to Use the if not Condition in Python
Najwa Riyaz
Feb 02, 2024
Python
Python Condition
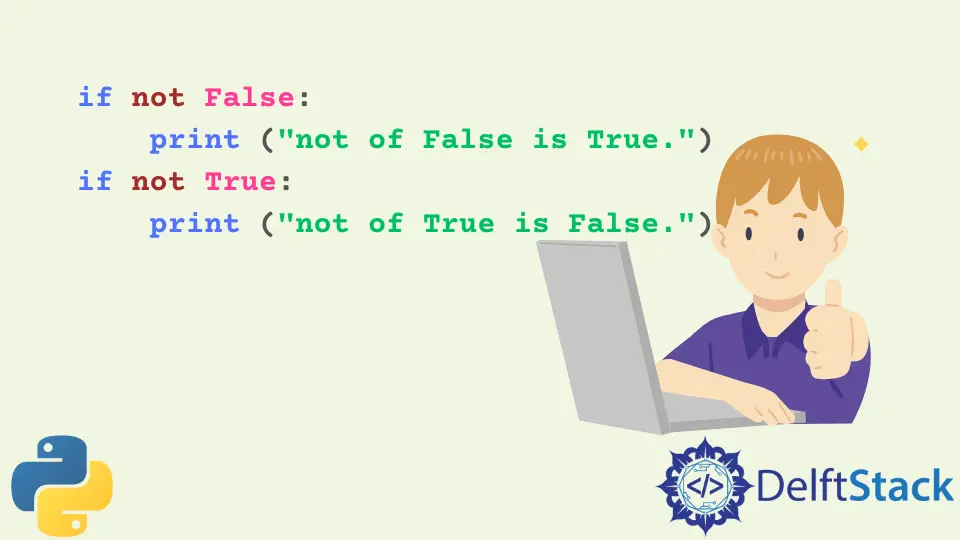
The if
statement is combined with the not
logical operator to evaluate if a condition did not happen.
This article explains how to use the if not
condition in Python.
Here’s a code block that demonstrates this condition.
if not a_condition:
block_of_code_to_execute_if_condition_is_false
In the case above, the code block_of_code_to_execute_if_condition_is_false
will be executed successfully if the result of a_condition
is False
.
True and False Values in Python
Before we begin, let us understand that the equivalent value is False
in Python in the following cases:
- Numeric zero values, such as
0
,0L
,0.0
- Empty sequences such as:
- empty list []
- empty dictionary {}
- empty string ''
- empty tuple
- empty set
- a
None
object
Examples of the if not
Condition in Python
Here are several examples that help you understand how if not
is utilized in Python.
Usage of Boolean
Values
if not False:
print("not of False is True.")
if not True:
print("not of True is False.")
Output:
not of False is True.
Usage of a numeric
Value
For example, values like 0
, 0L
,0.0
are associated with the value False
.
if not 0:
print("not of 0 is True.")
if not 1:
print("not of 1 is False.")
Output:
not of 0 is True.
Usage of List
of Values
if not []:
print("An empty list is false. Not of false =true")
if not [1, 2, 3]:
print("A non-empty list is true. Not of true =false")
Output:
An empty list is false. Not of false =true
Usage of Dictionary
Values
if not {}:
print("An empty dictionary dict is false. Not of false =true")
if not {"vehicle": "Car", "wheels": "4", "year": 1998}:
print("A non-empty dictionary dict is true. Not of true =false")
Output:
An empty dictionary dict is false. Not of false =true
Usage of String
of Values
if not "":
print("An empty string is false. Not of false =true")
if not "a string here":
print("A non-empty string is true. Not of true =false")
Output:
An empty string is false. Not of false =true
Usage of a None
Value:
if not None:
print("None is false. Not of false =true")
Output:
None is false. Not of false =true
Usage of set
of Values:
dictvar = {}
print("The empty dict is of type", type(dictvar))
setvar = set(dictvar)
print("The empty set is of type", type(setvar))
if not setvar:
print("An empty set is false. Not of false =true")
Output:
The empty dict is of type <class 'dict'>
The empty set is of type <class 'set'>
An empty dictionary dict is false. Not of false =true
Usage of a tuple
of Values
An Empty Tuple Is Associated With the Value False
.
if not ():
print("1-An empty tuple is false. Not of false =true")
if not tuple():
print("2-An empty tuple is false. Not of false =true")
Output:
1-An empty tuple is false. Not of false =true
2-An empty tuple is false. Not of false =true
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe