Use a condição if not em Python
Najwa Riyaz
10 outubro 2023
Python
Python Condition
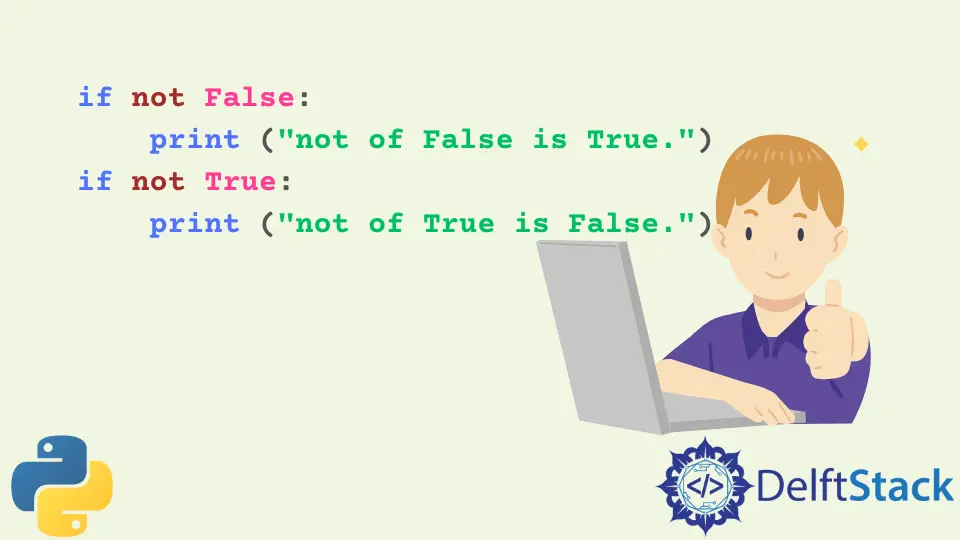
A instrução if
é combinada com o operador lógico not
para avaliar se uma condição não aconteceu. Este artigo explica como usar a condição if not
em Python.
Aqui está um bloco de código que demonstra essa condição.
if not a_condition:
block_of_code_to_execute_if_condition_is_false
No caso acima, o código block_of_code_to_execute_if_condition_is_false
será executado com sucesso se o resultado de a_condition
for False
.
Valores verdadeiros e falsos em Python
Antes de começar, vamos entender que o valor equivalente é False
em Python nos seguintes casos:
- Valores numéricos de zero, como
0
,0L
,0.0
- Sequências vazias, como:
- lista vazia []
- dicionário vazio {}
- string vazia ''
- tupla vazia
- conjunto vazio
- um objeto
None
Exemplos da condição if not
em Python
Aqui estão vários exemplos que ajudam a entender como if not
é utilizado em Python.
Uso de valores booleanos
if not False:
print("not of False is True.")
if not True:
print("not of True is False.")
Resultado:
not of False is True.
Uso de um valor numérico
Por exemplo, valores como 0
, 0L
, 0.0
estão associados ao valor False
.
if not 0:
print("not of 0 is True.")
if not 1:
print("not of 1 is False.")
Resultado:
not of 0 is True.
Uso de Lista
de Valores
if not []:
print("An empty list is false. Not of false =true")
if not [1, 2, 3]:
print("A non-empty list is true. Not of true =false")
Resultado:
An empty list is false. Not of false =true
Uso de valores de Dicionário
if not {}:
print("An empty dictionary dict is false. Not of false =true")
if not {"vehicle": "Car", "wheels": "4", "year": 1998}:
print("A non-empty dictionary dict is true. Not of true =false")
Resultado:
An empty dictionary dict is false. Not of false =true
Uso de String
de Valores
if not "":
print("An empty string is false. Not of false =true")
if not "a string here":
print("A non-empty string is true. Not of true =false")
Resultado:
An empty string is false. Not of false =true
Uso de um valor None
:
if not None:
print("None is false. Not of false =true")
Resultado:
None is false. Not of false =true
Uso de set
de Valores:
dictvar = {}
print("The empty dict is of type", type(dictvar))
setvar = set(dictvar)
print("The empty set is of type", type(setvar))
if not setvar:
print("An empty set is false. Not of false =true")
Resultado:
The empty dict is of type <class 'dict'>
The empty set is of type <class 'set'>
An empty dictionary dict is false. Not of false =true
Uso de uma tuple
de Valores
Uma tupla vazia está associada ao valor False
.
if not ():
print("1-An empty tuple is false. Not of false =true")
if not tuple():
print("2-An empty tuple is false. Not of false =true")
Resultado:
1-An empty tuple is false. Not of false =true
2-An empty tuple is false. Not of false =true
Está gostando dos nossos tutoriais? Inscreva-se no DelftStack no YouTube para nos apoiar na criação de mais vídeos tutoriais de alta qualidade. Inscrever-se