How to Peek Heapq in Python
- Heap in Python
-
Peek Into Heap With the
heap[0]
Notation in Python -
Peek Into Heap With the
heappop()
Function in Python -
Peek Into Heap With the
nsmallest()
Function in Python
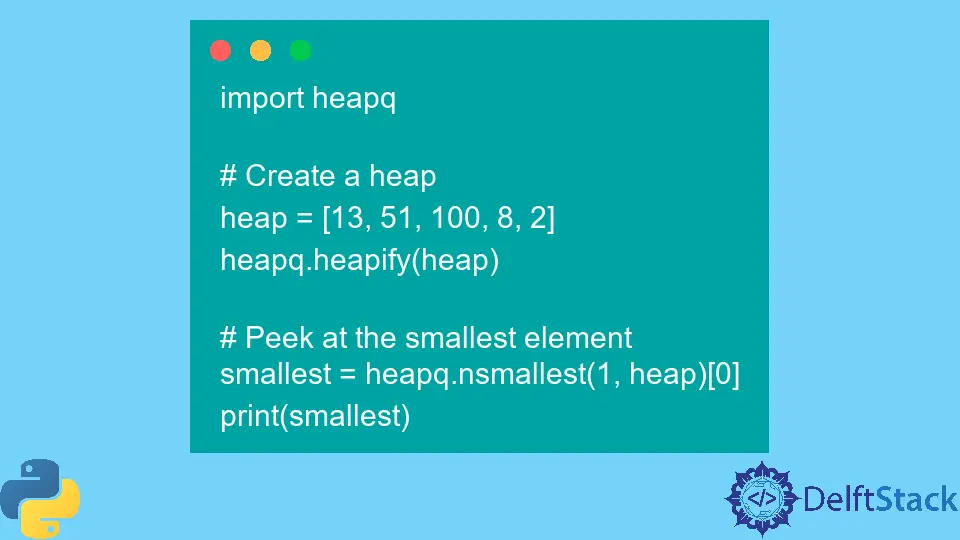
In this tutorial, we will explore different ways of peeking at the smallest element in a heap created using Python’s heapq
library.
Heap in Python
A heap is a special data structure similar to a binary tree.
It has two main properties: the first is a complete binary tree, meaning that all levels of the tree are filled, except possibly for the last level, which is filled from left to right.
The second property is that it is a minimum heap, meaning that the value of each parent node is less than or equal to the values of its children.
The smallest element in a heap is always the root of the tree. A heap is a data structure allowing efficient access to the smallest element.
In Python, the heapq
library provides a way to create and manipulate heaps. One important operation on a heap is the ability to peek at the smallest element without removing it.
Peek Into Heap With the heap[0]
Notation in Python
The most straightforward way to peek at the smallest element in a heap is by using the heap[0]
notation. This will return the smallest element of the heap without removing it.
The following code snippet shows how we can use the heap[0]
notation to peek at the smallest element inside a heap in Python.
import heapq
# Create a heap
heap = [13, 51, 100, 8, 2]
heapq.heapify(heap)
# Peek at the smallest element
smallest = heap[0]
print(smallest)
Output:
2
In the above code example, we first import the heapq
library and create a list of integers. We then use the heapify()
function to convert this list into a heap.
Finally, we use the heap[0]
notation to peek at the smallest element of the heap, which is the first element of the list.
Peek Into Heap With the heappop()
Function in Python
Another way to peek at the smallest element in a heap is by using the heappop()
function. This function removes the smallest element from the heap and returns it.
The following code snippet shows how we can use the heapq.heappop()
function to peek at the smallest element inside a heap in Python.
import heapq
# Create a heap
heap = [13, 51, 100, 8, 2]
heapq.heapify(heap)
# Peek at the smallest element
smallest = heapq.heappop(heap)
print(smallest)
Output:
2
In the above code example, we first import the heapq
library and create a list of integers. We then use the heapify()
function to convert this list into a heap.
Finally, we use the heappop()
function to peek at the smallest element of the heap, which is removed from the heap after this operation. This method is useful when we need to maintain the heap property but also want to see the smallest element.
Peek Into Heap With the nsmallest()
Function in Python
Another way to peek at the smallest element in a heap is by using the nsmallest()
function. This function takes in a number n
and returns the n
smallest elements from the heap without removing them.
The following code snippet shows how we can use the nsmallest()
function to peek at the smallest element inside a heap in Python.
import heapq
# Create a heap
heap = [13, 51, 100, 8, 2]
heapq.heapify(heap)
# Peek at the smallest element
smallest = heapq.nsmallest(1, heap)[0]
print(smallest)
Output:
2
In the above code example, we first import the heapq
library and create a list of integers. We then use the heapify()
function to convert this list into a heap.
Finally, we use the nsmallest()
function to peek at the smallest element of the heap by passing 1 as the first argument, this returns a list of the smallest element, and we access the element by indexing it.
In conclusion, there are several ways to peek at the smallest element in a heap created using the heapq
library in Python. The choice of which method to use will depend on the problem’s specific requirements and the heap’s desired behavior.
The heap[0]
notation, heappop()
, and nsmallest()
are all useful methods to peek at the smallest element. Always be mindful of the trade-off between memory efficiency and performance, and choose the right method accordingly.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn