If a goto Statement Exists in Python
-
Understanding the Absence of
goto
in Python - Using Loops for Control Flow
- Leveraging Functions for Reusability
- Utilizing Exceptions for Control Flow
- Conclusion
- FAQ
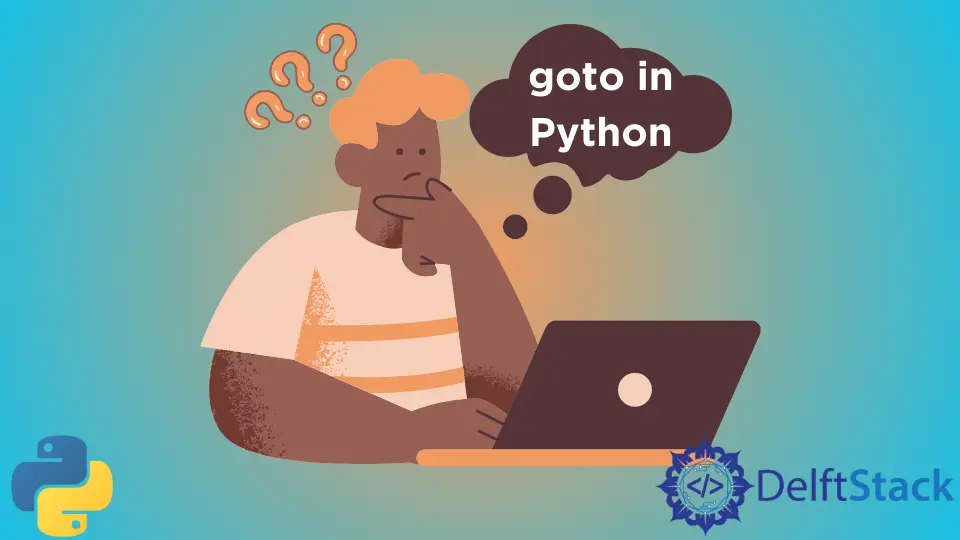
In the world of programming, control flow is a fundamental concept that dictates how a program executes its instructions. Many languages have a goto
statement that allows developers to jump to different parts of the code. However, Python takes a different approach.
This article explores whether a goto
statement exists in Python and discusses alternative methods to manage control flow effectively. If you’re curious about how Python handles jumps in code execution, you’ve come to the right place. We’ll delve into the reasons behind Python’s design choices and offer practical solutions that align with its philosophy.
Understanding the Absence of goto
in Python
Python does not include a goto
statement, and this is a deliberate design choice made by its creator, Guido van Rossum. The rationale behind this decision is rooted in Python’s philosophy, which emphasizes readability and maintainability. goto
can lead to what is known as “spaghetti code,” where the flow of execution becomes tangled and difficult to follow. Instead, Python encourages the use of structured programming techniques like loops and functions to manage control flow.
The absence of goto
in Python doesn’t mean you’re left without options. You can achieve similar outcomes using constructs like loops, functions, and exceptions. In the next sections, we will explore these alternatives.
Using Loops for Control Flow
One of the primary ways to manage control flow in Python is through loops. Loops allow you to repeat a block of code multiple times, which can mimic the behavior of goto
in certain scenarios. Here’s a simple example that demonstrates how to use a loop to control execution flow.
count = 0
while count < 5:
print("Count is:", count)
count += 1
Output:
Count is: 0
Count is: 1
Count is: 2
Count is: 3
Count is: 4
In this example, we have a while
loop that continues to execute as long as the count
variable is less than 5. Each iteration prints the current value of count
and increments it by 1. This structured approach is clear and easy to follow, contrasting with the potential chaos of goto
.
Using loops not only enhances readability but also makes your code easier to debug and maintain. When you structure your code with loops, you can clearly see the flow of execution and understand how data changes over time.
Leveraging Functions for Reusability
Another powerful feature of Python is the ability to define functions. Functions allow you to encapsulate a block of code and execute it whenever needed, which can also serve as an alternative to goto
. Here’s an example that illustrates how functions can help manage control flow.
def greet_user(name):
print("Hello,", name)
def main():
users = ["Alice", "Bob", "Charlie"]
for user in users:
greet_user(user)
main()
Output:
Hello, Alice
Hello, Bob
Hello, Charlie
In this code, we define a function called greet_user
that takes a name as an argument and prints a greeting. The main
function creates a list of users and uses a for
loop to call greet_user
for each user. This structure allows for clear separation of concerns and makes the code modular.
Using functions not only avoids the pitfalls of goto
but also promotes code reusability. You can call the same function from different parts of your program, reducing redundancy and enhancing maintainability.
Utilizing Exceptions for Control Flow
Exceptions are another mechanism in Python that can help control the flow of execution. They allow you to handle errors gracefully and can also be used to jump out of deeply nested structures. Here’s an example of how exceptions can be utilized for control flow.
def divide_numbers(a, b):
try:
result = a / b
except ZeroDivisionError:
return "Cannot divide by zero"
return result
print(divide_numbers(10, 2))
print(divide_numbers(10, 0))
Output:
5.0
Cannot divide by zero
In this example, the function divide_numbers
attempts to divide two numbers. If the second number is zero, it raises a ZeroDivisionError
, which is caught by the except
block. This allows the function to return a user-friendly message instead of crashing.
Using exceptions in this way provides a controlled means of handling errors and can help you manage complex control flows without resorting to goto
. It’s an elegant solution that aligns with Python’s design principles, promoting clarity and robustness in your code.
Conclusion
In summary, Python does not have a goto
statement, reflecting its commitment to readability and structured programming. Instead, Python offers a variety of control flow mechanisms, including loops, functions, and exceptions, that allow developers to manage execution flow effectively. By embracing these features, you can write cleaner, more maintainable code that adheres to Python’s philosophy. Whether you’re a beginner or an experienced developer, understanding these alternatives will enhance your programming skills and help you create more robust applications.
FAQ
-
Is there a
goto
statement in Python?
No, Python does not include agoto
statement as part of its design philosophy. -
What are the alternatives to
goto
in Python?
You can use loops, functions, and exceptions to control the flow of execution in Python. -
Why is
goto
considered bad practice?
goto
can lead to unstructured and difficult-to-maintain code, often referred to as “spaghetti code.” -
Can I use loops to replace
goto
functionality?
Yes, loops can effectively manage control flow and repeat code execution without the need forgoto
. -
How do functions improve code readability?
Functions encapsulate code, making it reusable and easier to understand, which enhances overall code clarity.