How to Start a for Loop at 1 in Python
-
Using the
range()
Function - Looping Through a List with Indexing
- Using Enumerate for Index Control
- Conclusion
- FAQ
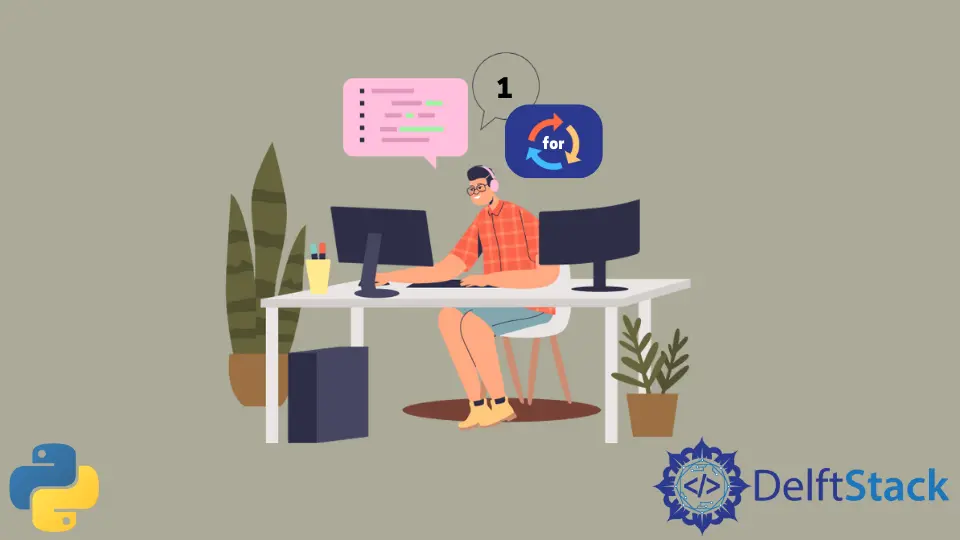
When working with loops in Python, particularly the for loop, you may find yourself needing to start the iteration from an index of 1 instead of the default 0. This is a common requirement, especially when dealing with user-facing data or specific algorithms where a 1-based index makes more sense.
In this tutorial, we’ll explore various methods to initiate a for loop at 1 in Python. By the end, you’ll have a solid understanding of how to manipulate loop indices to fit your needs, ensuring your code is both efficient and effective. Let’s dive in!
Using the range()
Function
One of the most straightforward methods to start a for loop at 1 in Python is by utilizing the range()
function. The range()
function generates a sequence of numbers, and you can specify the starting point, which is particularly useful when you want to begin at an index of 1.
Here’s how you can do it:
for i in range(1, 6):
print(i)
Output:
1
2
3
4
5
In this example, range(1, 6)
generates numbers starting from 1 up to, but not including, 6. This means the loop will iterate through the numbers 1, 2, 3, 4, and 5. Using range()
is an efficient way to control the starting point of your loop, allowing you to customize your iterations easily. It’s also worth noting that the second parameter in the range()
function defines the endpoint, so you can adjust it according to your needs. This method is particularly useful when you want to loop through a specific range of numbers, making it a versatile tool in your Python toolkit.
Looping Through a List with Indexing
If you’re working with a list and want to start your for loop at index 1, you can achieve this by slicing the list. By creating a new list that excludes the first element, you can effectively start your loop from the second item onward.
Here’s an example:
my_list = ['a', 'b', 'c', 'd', 'e']
for item in my_list[1:]:
print(item)
Output:
b
c
d
e
In this example, my_list[1:]
creates a new list that starts from the second element of my_list
. The slicing operation is a powerful feature in Python, allowing you to easily manipulate lists. By using this method, you can maintain the integrity of the original list while controlling which elements are processed in your loop. This approach is particularly useful when you need to skip the first element for any reason, such as when the first item is a header or not relevant to the current operation.
Using Enumerate for Index Control
Another effective way to start a for loop at index 1 is by using the enumerate()
function. This built-in function allows you to loop through an iterable while also keeping track of the index. By adjusting the starting index, you can easily control where your loop begins.
Here’s how it works:
my_list = ['a', 'b', 'c', 'd', 'e']
for index, item in enumerate(my_list, start=1):
print(index, item)
Output:
1 a
2 b
3 c
4 d
5 e
In this code snippet, enumerate(my_list, start=1)
starts the index at 1 instead of the default 0. This means that as you iterate through my_list
, the index will begin counting from 1, which can be particularly useful for displaying results in a user-friendly format. The combination of enumerate()
with a specified starting index not only provides the current index but also the corresponding item, making it a powerful tool for iterating through lists when you need both the index and the value.
Conclusion
Starting a for loop at index 1 in Python is a simple yet essential skill for many programming scenarios. Whether you’re using the range()
function, slicing lists, or leveraging the enumerate()
function, Python offers several methods to achieve this with ease. By mastering these techniques, you can write more intuitive and user-friendly code that meets your specific requirements. Remember, the choice of method often depends on the context of your problem, so it’s helpful to be familiar with multiple approaches. Happy coding!
FAQ
-
How do I start a for loop from a specific number other than 1?
You can use therange()
function to specify any starting number, likerange(start, end)
. -
Can I use a for loop to iterate through a dictionary starting at index 1?
Yes, you can convert the dictionary keys or values to a list and then slice it or useenumerate()
. -
Is there a performance difference between these methods?
Generally, the performance difference is negligible for small lists, butenumerate()
may be more efficient for larger datasets.
-
Can I start a for loop at an index greater than 1?
Absolutely! You can specify any starting index in therange()
or by slicing the list accordingly. -
What if I want to start at index 0 but only print from index 1?
You can loop fromrange(0, len(my_list))
and use an if statement to skip index 0.
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedIn