How to Use a for Loop for Multiple Variables in Python
-
Use the
for
Loop With theitems()
Method for Multiple Assignments in a Dictionary in Python -
Use the
for
Loop With theenumerate()
Function for Multiple Assignments in a List in Python -
Use the
for
Loop With thezip()
Function for Multiple Assignments in a Tuple or List in Python -
Use the
for
Loop With therange()
Function for Multiple Assignments in a List in Python -
Use Nested
for
Loops for Multiple Assignments in a List in Python - Conclusion
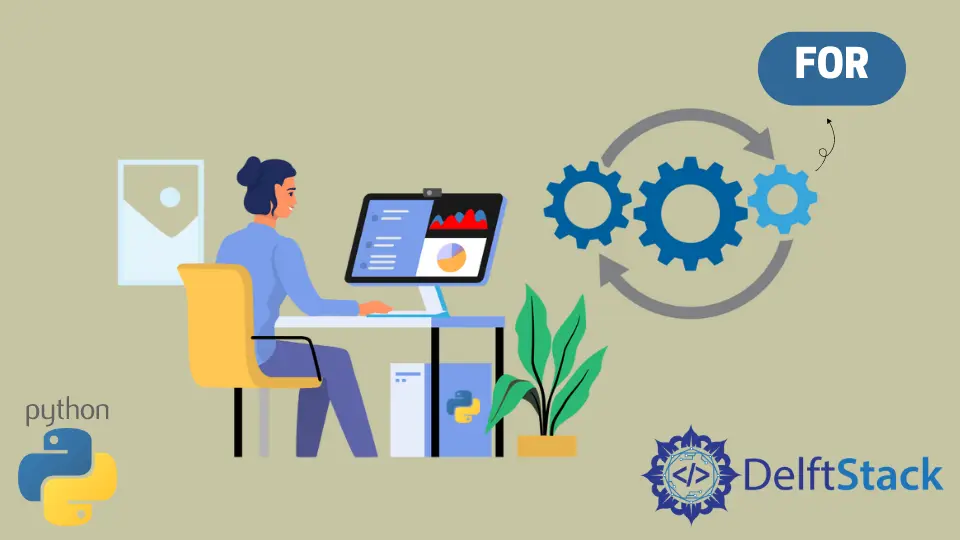
[A for
loop]({{relref “/HowTo/Python/one line for loop python.en.md”}}) is a fundamental control flow structure in Python, essential for iterating over various sequences such as lists, tuples, dictionaries, and even strings.
This article focuses on the use of the for
loop to handle multiple variables in Python.
Use the for
Loop With the items()
Method for Multiple Assignments in a Dictionary in Python
Dictionaries serve as a versatile data structure in Python, enabling the storage of data values in key-value pairs. In simple terms, a dictionary maps one value to another, analogous to how an English dictionary maps a word to its definition.
To achieve multiple assignments within a dictionary using a for
loop, the items()
method is employed on the given Python dictionary. This provides the output as a list containing all the dictionary keys along with their corresponding values.
The general syntax for the items()
method is:
dictionary.items()
Here, dictionary
is the dictionary for which you want to retrieve key-value pairs. The items()
method returns a view object that can be used to iterate over the dictionary’s key-value pairs as tuples.
Let’s take a closer look at the following code:
dict1 = {1: "Bitcoin", 2: "Ethereum"}
for key, value in dict1.items():
print(f"Key {key} has value {value}")
Output:
Key 1 has value Bitcoin
Key 2 has value Ethereum
In this example, the items()
method is used to retrieve the key-value pairs from the dict1
dictionary. The for
loop iterates over these pairs, allowing you to access and print each key and its corresponding value.
Use the for
Loop With the enumerate()
Function for Multiple Assignments in a List in Python
The enumerate()
function in Python is used to add a counter to an iterable and return it as an enumerate object. This proves especially useful when dealing with multiple lists, allowing for concurrent processing using indexes to access corresponding elements in the other list.
The general syntax for the enumerate()
function is as follows:
enumerate(iterable, start=0)
Parameters:
-
iterable
: This is the iterable (list, tuple, string, etc.) for which you want to generate an enumerate object. It can be any iterable object where you want to access both the elements and their indices. -
start
(optional): This parameter specifies the starting value of the index. By default, it starts from 0, but you can provide any integer value to start the index from a different number.
The enumerate()
function returns an enumerate object, which is an iterator that yields tuples containing an index and an element from the given iterable.
The following code snippet illustrates the use of the enumerate()
function for multiple assignments within a list:
coins = ["Bitcoin", "Ethereum", "Cardano"]
prices = [48000, 2585, 2]
for i, coin in enumerate(coins):
price = prices[i]
print(f"${price} for 1 {coin}")
Output:
$48000 for 1 Bitcoin
$2585 for 1 Ethereum
$2 for 1 Cardano
In this code, two lists, namely coins
and prices
, are simultaneously manipulated through assignment operations.
The enumerate()
object facilitates this process by providing the necessary indexes, enabling the efficient traversal of both lists concurrently.
Use the for
Loop With the zip()
Function for Multiple Assignments in a Tuple or List in Python
[The zip()
function]({{relref “/HowTo/Python/zip python 3.en.md”}}), a built-in feature in Python’s toolkit, is used to aggregate elements from multiple iterables (lists, tuples, etc.) into an iterator of tuples.
The general syntax for the zip()
function is as follows:
zip(*iterables)
Parameter:
-
*iterables
: This is the variable-length argument list. You can pass multiple iterables (lists, tuples, etc.) as separate arguments to thezip()
function.It accepts any number of iterables, and elements from each iterable will be paired together.
The zip()
function returns an iterator of tuples where each tuple contains the corresponding elements from the input iterables.
This function not only enables parallel interaction but also supports the simultaneous unpacking of multiple variables.
The code snippet below demonstrates the use of the zip()
function for multiple assignments within a tuple or list.
coins = ["Bitcoin", "Ethereum", "Cardano"]
prices = [48000, 2585, 2]
for coin, price in zip(coins, prices):
print(f"${price} for 1 {coin}")
Output:
$48000 for 1 Bitcoin
$2585 for 1 Ethereum
$2 for 1 Cardano
In this example, the zip()
function accepts two lists as parameters and generates an iterable. This iterable yields tuples that comprise corresponding elements from the given lists, presenting them as the loop iterates over it.
This significantly streamlines the handling of multiple variables within a loop. The output obtained shows the merging of elements from both lists.
One of the distinct advantages of zip()
is its ability to work with iterables of unequal lengths. When the iterables provided to zip()
differ in length, the resulting iterable will have the length of the shortest iterable.
This ensures that you can process and handle data from multiple sources even when their lengths aren’t identical.
Use the for
Loop With the range()
Function for Multiple Assignments in a List in Python
Handling multiple variables in Python can also be done using the range()
function with the len()
function. This allows for a structured traversal of multiple iterables, especially when accessing elements through their indices is necessary.
The general syntax for the range()
function is as follows:
range(start, stop[, step])
Parameters:
-
start
(optional): This parameter specifies the starting value of the range (inclusive). If omitted, the default value is 0. -
stop
: This parameter specifies the ending value of the range (exclusive). Therange()
function will generate numbers up to, but not including, this value. -
step
(optional): This parameter specifies the increment between each number in the range. If omitted, the default value is 1.
The range()
function, paired with len()
, can generate a sequence of indices that correspond to the position of elements within the iterables.
In the code snippet below, we have two lists: list1
containing numeric values [1, 2, 3]
and list2
containing corresponding alphabetic characters ['a', 'b', 'c']
. The objective here is to pair each element from list1
with its corresponding element from list2
using their respective indices.
Take a look at the example:
list1 = [1, 2, 3]
list2 = ["a", "b", "c"]
for i in range(len(list1)):
item1 = list1[i]
item2 = list2[i]
print(item1, item2)
Output:
1 a
2 b
3 c
In this specific example, the for
loop iterates through the indices generated by range(len(list1))
. This approach enables access to elements in both list1
and list2
by using the corresponding index.
For each iteration, the i
variable holds the current index, and item1
and item2
are assigned the elements from list1
and list2
at that index, respectively. Consequently, this method facilitates a pairing of elements, assuming the iterables are of the same length.
The code output shows the successful pairing of elements from both list1
and list2
.
Use Nested for
Loops for Multiple Assignments in a List in Python
Nested for
loops in Python provide a versatile approach to dealing with multiple variables in a structured and organized way. This allows you to navigate through several iterables, performing operations based on their positions or interrelationships.
In the provided code example, two lists, list1
and list2
, are used to demonstrate the usage of nested for
loops. list1
contains numerical values [1, 2, 3]
, while list2
consists of corresponding alphabetic characters ['a', 'b', 'c']
.
The goal is to systematically pair each element from list1
with every element from list2
.
See the code example:
list1 = [1, 2, 3]
list2 = ["a", "b", "c"]
for item1 in list1:
for item2 in list2:
print(item1, item2)
Output:
1 a
1 b
1 c
2 a
2 b
2 c
3 a
3 b
3 c
The outer loop, represented by for item1 in list1:
, takes each element from list1
and initiates the inner loop. The inner loop, represented by for item2 in list2:
, then traverses through list2
, systematically pairing each element from list1
with all elements from list2
.
We can see that the output of this code illustrates the pairing of the elements from both lists.
Conclusion
Using multiple variables within a for
loop in Python is a valuable technique that can be applied effectively to lists or dictionaries. However, it’s important to note that it’s not a general-purpose approach and requires caution.
This technique, known as iterable unpacking, involves assigning multiple variables simultaneously in a single line of code.
Using the methods discussed above, you can effectively utilize for
loops to handle multiple variables in Python. Whether you need to access elements based on indices or traverse iterables in a nested fashion, these techniques provide the flexibility and control needed to work with multiple variables seamlessly.
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedIn