How to Increment by 2 in Python for Loop
-
Increment by 2 in a Python
for
Loop With therange()
Function -
Increment by 2 in Python
for
Loop Using the Slicing Method -
Increment by 2 in Python
for
Loop Using the+=
Operator - Conclusion
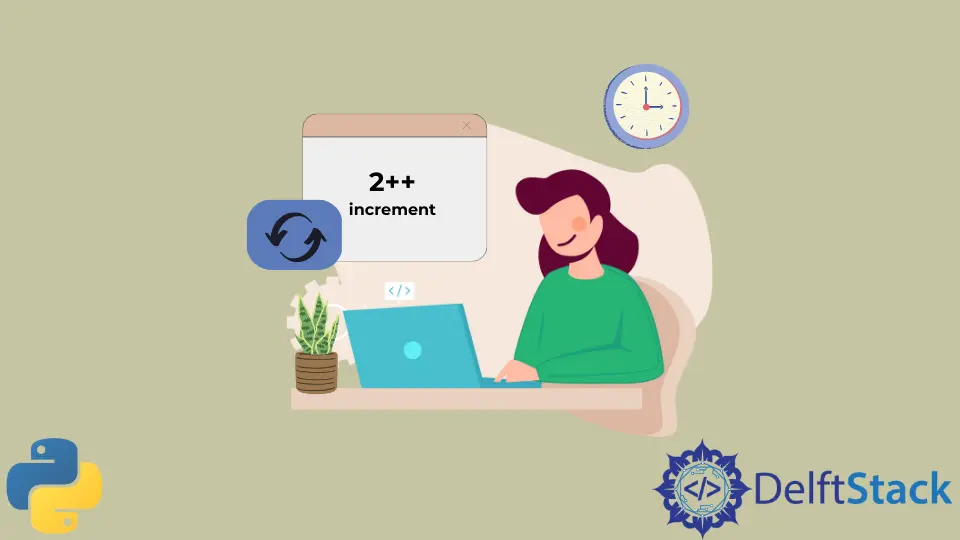
In Python programming, the ability to increment a variable by a specific value during each iteration is a common necessity. One frequent requirement is the need to increase the variable by 2.
In this article, we explore three distinct methods for achieving this within a Python for
loop. Whether you’re a beginner or an experienced developer, these methods offer valuable insights into optimizing your code for precise iteration control.
Increment by 2 in a Python for
Loop With the range()
Function
The range()
function is a built-in Python function that generates a sequence of numbers within a specified range. It has the following syntax:
range(start, stop, step)
start
: The starting value of the sequence.stop
: The stopping value of the sequence (exclusive).step
: The step or increment value between the numbers.
By leveraging the range()
function, we can easily create a for
loop that increments a variable by a specific value in each iteration.
To increment by 2 in a Python for
loop, you can set the step
parameter of the range()
function to 2
.
Here’s how to do it:
for i in range(start, stop, 2):
start
: The initial value of your counter variable.stop
: The value at which you want the loop to stop (exclusive).2
: This sets the step value to2
, causing the counter variable to increment by 2 in each iteration.
Let’s illustrate this with a practical example. Suppose you want to print all even numbers between 0
and 10
using a for
loop with a 2-unit increment.
You can achieve it like this:
for i in range(0, 11, 2):
print(i)
Output:
0
2
4
6
8
10
In this example, the for
loop starts from 0
, stops just before 11
, and increments the counter variable by 2
in each iteration, effectively printing even numbers. As seen in the output, the program successfully prints all the even numbers between 0
to 10
by having the value of i
increment by 2
in each iteration.
Increment by 2 in Python for
Loop Using the Slicing Method
The slicing method in Python is a powerful way to extract a portion of a sequence, such as a list, string, or tuple, by specifying a start
, end
, and step
. The syntax for slicing is as follows:
sequence[start:end:step]
start
: The index where the slice begins.end
: The index where the slice ends (exclusive).step
: The step or increment value to move through the sequence.
By utilizing the slicing method, we can efficiently increment a variable by a specific value in each iteration of a for
loop.
To increment a variable by 2 in a Python for
loop using the slicing method, follow these steps:
-
Create a sequence, such as a list, that contains the values you want to iterate through.
-
Create a
for
loop with the sequence and use slicing to select elements with a step of2
in each iteration.
Here’s a guide on how to do it:
sequence = [value1, value2, value3, ...]
for element in sequence[::2]:
# Your code here
Let’s illustrate this with a practical example. Suppose you want to print all even numbers between 0
and 10
using a for
loop with a 2-unit increment.
You can do it like this:
numbers = list(range(11))
for number in numbers[::2]:
print(number)
Output:
0
2
4
6
8
10
In this example, we create a list of even numbers, and within the for
loop, we directly iterate through the list, effectively printing the desired values.
Be aware that this method copies the original list to a new memory space. It will have a bad speed performance if the list is huge.
Increment by 2 in Python for
Loop Using the +=
Operator
The +=
operator is used for in-place addition in Python. It allows you to update the value of a variable by adding another value to it.
The syntax is as follows:
variable += value
This operator is particularly useful when you want to modify a variable’s value and store the result back in the same variable.
To increment a variable by 2 in a Python for
loop using the +=
operator, follow these steps:
-
Initialize a variable to the starting value.
-
Create a
for
loop with the desired range of iterations. -
Inside the loop, use the
+=
operator to increment the variable by2
.
Here’s how to do it:
counter = start_value
for i in range(number_of_iterations):
# Your code here
counter += 2
start_value
: The initial value of your counter variable.number_of_iterations
: The total number of iterations you want to perform.
Let’s illustrate this with a practical example. Suppose you want to print all even numbers between 0
and 10
using a for
loop with a 2-unit increment.
You can achieve it like this:
counter = 0
for i in range(6):
print(counter)
counter += 2
Output:
0
2
4
6
8
10
In this example, we initialize the counter
variable to 0
, and within the for
loop, we use the +=
operator to increment it by 2
in each iteration, effectively printing even numbers.
Conclusion
In Python, incrementing variables within for
loops by 2, or any desired value, is a task made simple by the language’s versatility. The methods we’ve explored, including utilizing the range()
function, the slicing method, and the +=
operator, provide you with options for achieving this common programming requirement.
With these tools at your disposal, you can efficiently manipulate variables during iterations and unlock new possibilities for your Python programming projects.