How to Exit Commands in Python
-
Exit Programs With the
quit()
Function in Python -
Exit Programs With the
exit()
Function in Python -
Exit Programs With the
sys.exit()
Function in Python -
Exit Programs With the
os._exit()
Function in Python
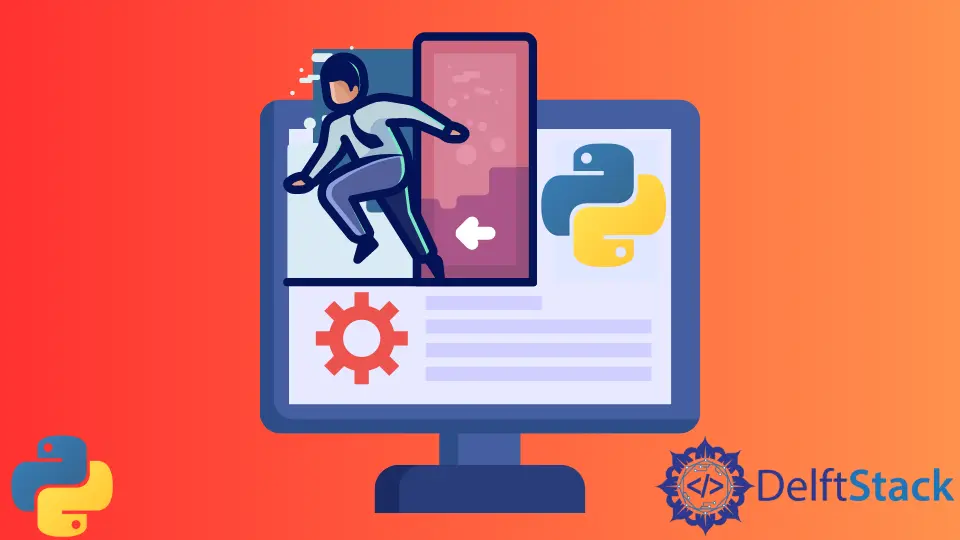
This tutorial will discuss the methods to exit a program in Python.
Exit Programs With the quit()
Function in Python
Whenever we run a program in Python, the site
module is automatically loaded into the memory. This site
module contains the quit()
function,, which can be used to exit the program within an interpreter. The quit()
function raises a SystemExit
exception when executed; thus, this process exits our program.
The following code shows us how to use the quit()
function to exit a program.
print("exiting the program")
print(quit())
Output:
exiting the program
We exited the program with the quit()
function in the code above. The quit()
function is designed to work with the interactive interpreter and should not be used in any production code. Take note that the quit()
function depends upon the site
module.
Exit Programs With the exit()
Function in Python
The exit()
function is also included inside the site
module of Python. This function does the same thing as the quit()
function. Both these functions were added to make Python more user-friendly. The exit()
function also raises a SystemExit
exception when executed.
The following program shows us how to use the exit()
function to exit a program.
print("exiting the program")
print(exit())
Output:
exiting the program
We exited the program with the exit()
function in the code above. However, the exit()
function is also designed to work with the interactive interpreter and should not be used in any production code as well. The reason is that the exit()
function also depends upon the site
module.
Exit Programs With the sys.exit()
Function in Python
The sys.exit()
function also does the same job as the previous functions because it’s included in the sys
module of Python. The sys.exit()
also raises a SystemExit
exception when executed. But unlike the previous two methods, this method is intended to be used in the production code.
This method does not depend on the site
module, and the sys
module is always available in production code. The program below shows us how to exit a program using the sys.exit()
function.
import sys
print("exiting the program")
print(sys.exit())
Output:
exiting the program
We exited the program with the sys.exit()
function in the code above. For this approach to work, you have to import the sys
module into our program.
Exit Programs With the os._exit()
Function in Python
This function is contained inside the os
module of Python. The os._exit()
function exits a process without calling any cleanup handlers or flushing stdio
buffers. This process does not provide you with a very graceful way to exit a program, but it works.
Ideally, this method should be reserved for special scenarios like the killing-a-child process. You can also use this function inside the production code because it does not depend upon the site
module, and we can always use the os
module in our production code.
The following code snippet shows us how to exit a program with the os._exit()
function.
import os
print("exiting the program")
print(os._exit(0))
Output:
exiting the program
We exited the program with the os._exit()
function in the code above. We have to import the os
module inside our code for this method to work and specify an exit code inside the os._exit()
function.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn