How to Process Finished With Exit Code 0 in Python
- Exit Codes in Python
- Process Finished With Exit Code 0 in Python
- Nonzero Exit Codes in Python
- Conclusion
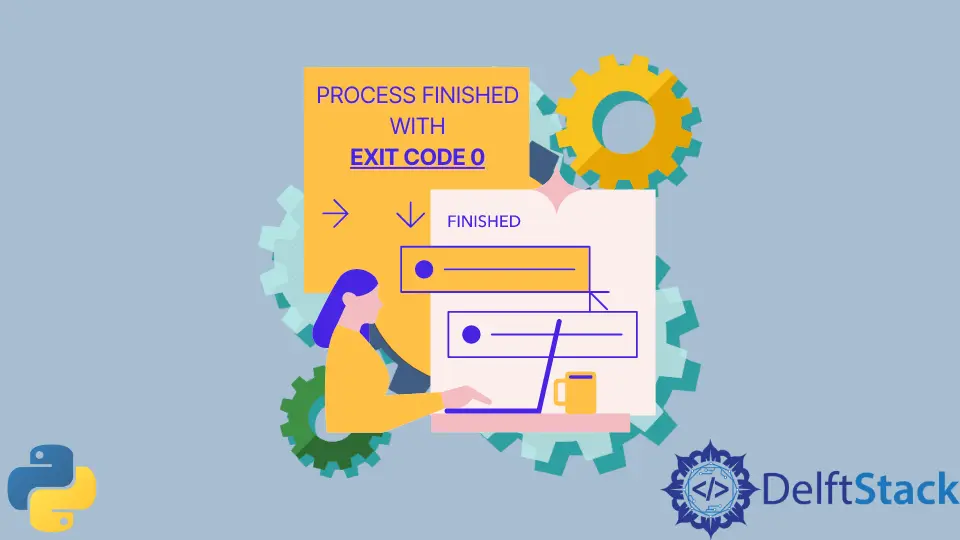
Exit code 0 is a fundamental concept in programming, especially when it comes to running scripts and applications.
In Python, as in many other programming languages, the exit code provides crucial information about the outcome of a program’s execution. An exit code of 0 is significant, indicating that the program terminated successfully without any errors.
Exit Codes in Python
An exit code is a small integer returned by a process to the operating system upon its termination. The range of exit codes varies across operating systems, but the convention is to use 0 to indicate successful termination and any nonzero value (often 1) to denote an error or abnormal termination.
In Python, the sys
module provides the exit()
function, allowing you to set the exit code for your script. When you exit a Python script without specifying an exit code, the default value is 0.
Process Finished With Exit Code 0 in Python
When a Python script or program terminates and returns an exit code of 0, it signifies successful execution. This implies that the script completed its intended tasks without encountering any major issues or errors.
Here are some scenarios where you might expect an exit code of 0:
The most common case is when your Python script runs without any errors, completes its tasks, and reaches the end of its execution.
For instance:
print("Hello World!")
Output:
After running this script, it will print "Hello World!"
and exit with a code of 0, indicating that the program ran successfully.
You can explicitly set the exit code to 0 using the sys
module’s exit()
function. This is often used to indicate success, even when there are no visible indicators within the program itself:
import sys
sys.exit(0)
In this case, you’re terminating the program and specifying an exit code of 0, signaling a successful execution.
Nonzero Exit Codes in Python
On the other hand, if a program encounters an error or experiences a problem during its execution, it can exit with a nonzero exit code. These codes typically provide more information about the type of error encountered, allowing for more granular handling of program terminations.
For example, if a script encounters an error or an exception, it might exit with an exit code other than 0 to indicate that something went wrong.
import sys
try:
result = 10 / 0
except ZeroDivisionError:
sys.exit(1)
Here, the try
block is where we place the code that we anticipate might raise an exception. Inside this block, there’s a division operation 10 / 0
, which will raise a ZeroDivisionError
since you cannot divide a number by zero in Python.
Following this, the except ZeroDivisionError
block is used to handle the ZeroDivisionError
exception. If this exception is raised during the execution of the code inside the try
block, the interpreter will execute the code inside this except
block.
Inside the except
block, sys.exit(1)
is called, which will tell the program to exit with an exit code of 1. An exit code of 1 typically indicates that an error occurred during the program’s execution.
The sys.exit()
function terminates the program and returns the specified exit code to the operating system.
Conclusion
In Python, an exit code of 0 is a positive sign, denoting that the program completed successfully without encountering any major issues. Understanding exit codes and using them effectively can help you build more robust and error-handling code, improving the reliability and stability of your applications.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn