How to Empty a Dictionary in Python
-
Assign
{}
to Empty a Dictionary in Python -
Use the
clear()
Function to Remove All Elements Inside a Dictionary in Python
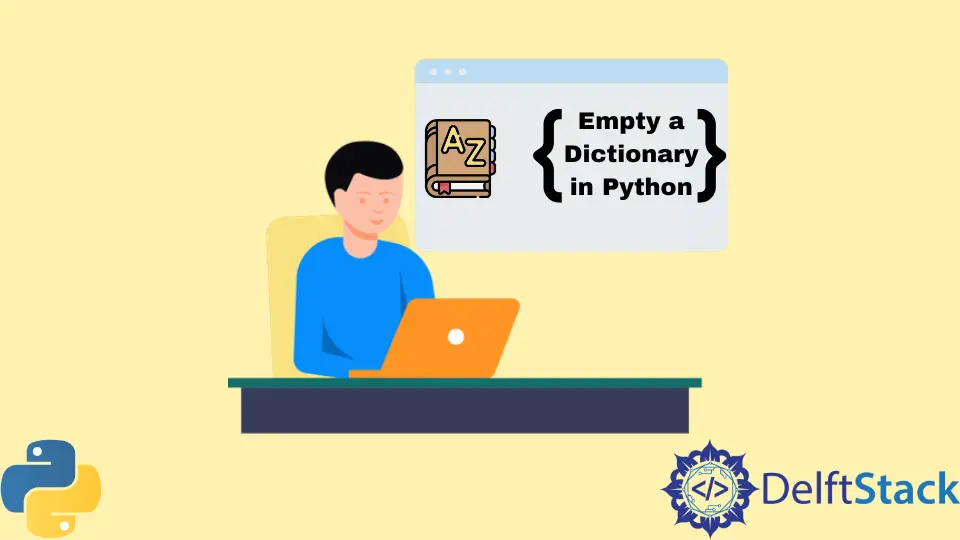
This tutorial will demonstrate how to empty a dictionary in Python.
Assign {}
to Empty a Dictionary in Python
A simple solution to empty a dictionary in Python is assigning {}
to a dictionary.
Assigning {}
instantiates a new object and replaces the existing dictionary. This means that a new reference in memory is assigned for a new dictionary, and the allocated space in memory for the old dictionary that was replaced is tagged for garbage collection.
dct = {"name": "John", "age": 23}
dct = {}
print("Dictionary contains:", dct)
Output:
Dictionary contains : {}
Use the clear()
Function to Remove All Elements Inside a Dictionary in Python
Another approach is to use the built-in function in Python, clear()
, which removes all contents existing in the dictionary. The function doesn’t have any parameters and has no return value. Its sole purpose is only to clear the contents of a dictionary.
dict = {"name": "John", "age": 23}
dict.clear()
print("Dictionary contains :", dict)
Output:
Dictionary contains : {}
The difference between assigning {}
and clear()
function is that the latter does not create a new instance but removes all the content of the existing dictionary as well as the references of the dictionary because the content is cleared.
Let’s compare these two approaches in the same use case.
Using the built in function, clear()
:
dict1 = {"name": "John", "age": 23}
dict2 = dict1
dict1.clear()
print("After using clear()")
print("Dictionary 1 contains :", dict1)
print("Dictionary 2 contains :", dict2)
Output:
After using clear()
Dictionary 1 contains : {}
Dictionary 2 contains : {}
As you can see, dict1
and dict2
are now empty.
Assigning the dictionary to {}
:
dict1 = {"name": "John", "age": 23}
dict2 = dict1
# Assign {} removes only dict1 contents
dict1 = {}
print("After assigning {}")
print("Dictionary 1 contains :", dict1)
print("Dictionary 2 contains :", dict2)
Output:
After assigning {}
Dictionary 1 contains : {}
Dictionary 2 contains : {'name': 'John', 'age': 23}
In the example above, dict1 = {}
created a new empty dictionary, whereas dict2
still points to the old value of dict1
, which leaves the values in dict2
values unchanged. In this case, garbage collection is not triggered because there is still another variable referencing the dictionary.
In summary, using the {}
to clear all the contents in a dictionary creates a new instance instead of updating the existing dictionary reference. If you have another name referring to the same dictionary, it is best to use the clear()
function so that all references to the same instance are also cleared.