How to Find Key by Value in Python Dictionary
-
Use
dict.items()
to Find Key by Value in Python Dictionary -
Use
.keys()
and.values()
to Find Key by Value in Python Dictionary
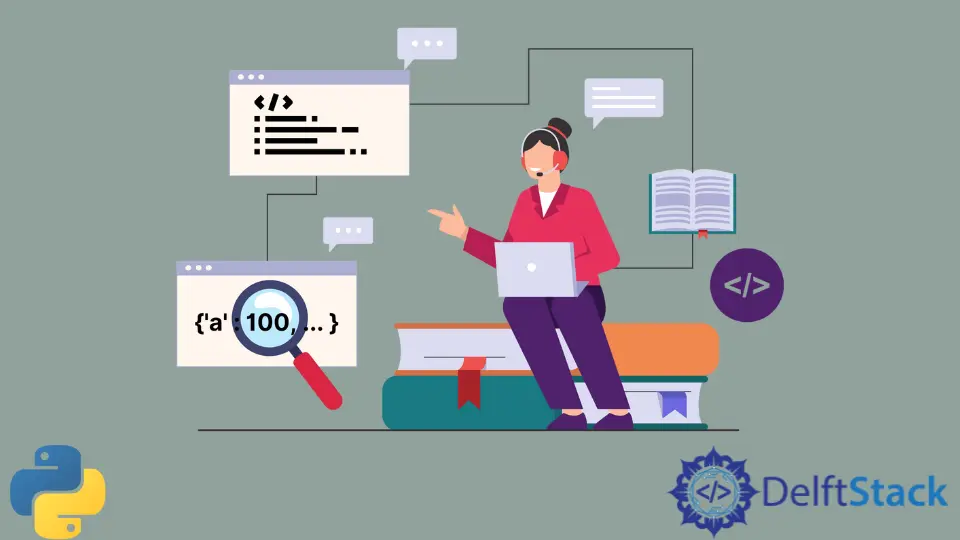
Dictionary is a collection of items in a key-value pair. Items stored in a dictionary are unordered. In this article, we will introduce different ways to find key by value in a Python dictionary. Usually, a value is accessed using a key, but here we will access a key using a value. Here the key is an identity associated with the value.
Use dict.items()
to Find Key by Value in Python Dictionary
dict.items()
method returns a list whose individual element is a tuple consisting of the key of the value of the dictionary. We can get the key by iterating the result of dict.items()
and comparing the value with the second element of the tuple.
Example Code:
my_dict = {"John": 1, "Michael": 2, "Shawn": 3}
def get_key(val):
for key, value in my_dict.items():
if val == value:
return key
return "There is no such Key"
print(get_key(1))
print(get_key(2))
Output:
John
Michael
Use .keys()
and .values()
to Find Key by Value in Python Dictionary
dict.keys()
returns a list consisting keys of the dictionary; dict.values()
returns a list consisting of values of the dictionary. The order of the items generated in .keys()
and .values()
are the same.
The index()
method of the Python list gives the index of the given argument. We can pass the value
to the index()
method of the generated key list to get the index of this value. Then the key could be obtained by accessing the generated value list with the returned index.
my_dict = {"John": 1, "Michael": 2, "Shawn": 3}
list_of_key = list(my_dict.keys())
list_of_value = list(my_dict.values())
position = list_of_value.index(1)
print(list_of_key[position])
position = list_of_value.index(2)
print(list_of_key[position])
Output:
John
Michael
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook