How to Copy String in Python
- Use an Empty String to Get a Copy String in Python
- Use Slicing to Copy a String in Python
-
Use the
str()
Function to Copy a String in Python - Use String Formatting to Copy a String in Python
-
Use the
format()
Method to Copy a String in Python -
Use
f-string
to Copy a String in Python - Conclusion
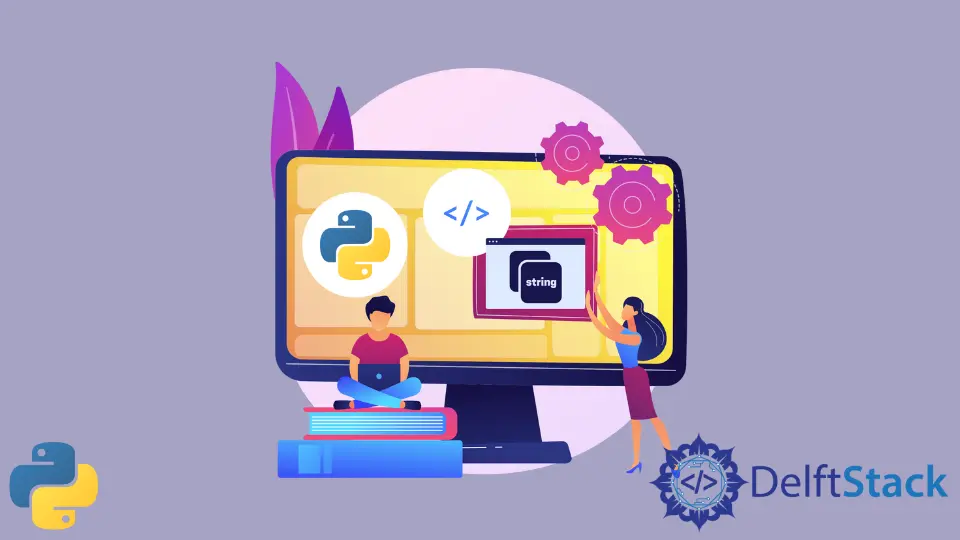
This tutorial will introduce different methods to copy a string in Python. Before we move on to the different methods to copy a string in Python, we should keep in mind that a string cannot be directly copied.
In Python, strings are immutable, meaning that their value cannot change for the program. Being immutable also means that a string cannot directly have a copy.
If a new variable is declared and is directly assigned the value of a given string variable, this would not create a copy of the original string. Instead, both of the created variables would point to the same string.
However, there are a few loopholes that can be utilized to copy a string in Python. We will discuss the methods below in this article.
Use an Empty String to Get a Copy String in Python
We start with the simplest method, which is very easy to implement. We need to add an empty string to the original string using the concatenation
operator while declaring the new string.
Example Code:
ostr = "Web"
nstr = "" + ostr
print(nstr)
Output:
Web
In this code, we initialize a string variable ostr
with the value "Web"
. Subsequently, we create a new string nstr
by concatenating an empty string ""
with the original string ostr
, and the +
operator is used for concatenation in this case.
Finally, we print the resulting string nstr
to the console. The output of this code is Web
, as the concatenation with an empty string essentially duplicates the content of the original string.
Use Slicing to Copy a String in Python
The slice or the :
operator can be utilized to slice the original and generate a new one. The slicing
operator works by taking a start
and an index
value in its syntax.
If both these values are not passed, the original string will be copied intact to the new variable.
Example Code:
ostr = "Web"
nstr = ostr[:]
print(nstr)
Output:
Web
In this code, we define ostr
as a string variable with the value "Web"
. We then create a new string nstr
using string slicing, denoted by ostr[:]
, and this slicing notation extracts a copy of the entire original string, essentially duplicating its content.
Finally, we print the resulting string nstr
to the console. The output will be Web
, representing the duplicated content of the initial string.
Use the str()
Function to Copy a String in Python
The str()
function in Python is a built-in function that converts an object into a string representation. Its primary purpose is to provide a human-readable version of an object.
The str()
function, when made to pass a given string as its argument, returns the original string itself. This can be utilized when we have to create a copy string.
Basic Syntax:
str(object)
In the syntax, the str()
function in Python doesn’t require any parameters, but it can take an object
as an argument that you want to convert to a string.
Example Code:
ostr = "Web"
nstr = str(ostr)
print(nstr)
Output:
Web
In this code, we initialize a string variable ostr
with the value "Web"
. To create a copy of this string, we use the str()
function, applying it to the original string ostr
, and this function converts the input to its string representation.
Subsequently, we assign the result to a new variable, nstr
. Finally, we print the copied string nstr
to the console, and the output will be Web
, representing the copied content of the initial string.
Use String Formatting to Copy a String in Python
String formatting supplies a wide variety of customizations for the user to select from in the Python code. The %
sign is commonly implied as the interpolation operator and is utilized to implement string formatting.
Although there is more than one way to implement string formatting in Python, the interpolation operator or %
sign is very versatile. It works on all available versions of Python and is the oldest of the lot.
The %
sign, along with a letter representing the conversion type, is marked as a placeholder for the variable.
Example Code:
ostr = "Web"
nstr = "%s" % ostr
print(nstr)
Output:
Web
In this code, we begin by initializing a string variable ostr
with the value "Web"
. To create a copy of this string, we use the %
operator within a string, followed by % ostr
, and this syntax is an older style of string formatting in Python.
Next, we assign the resulting formatted string to a new variable, nstr
. Finally, we print the copied string nstr
to the console, and the output will be Web
, representing the duplicated content of the initial string.
Use the format()
Method to Copy a String in Python
The format()
method in Python is a built-in string method that allows you to format strings by inserting values into placeholders within the string. It provides a versatile way to create strings by replacing placeholders with the values of variables or expressions.
We can use the format()
method to copy a string in Python.
Basic Syntax:
formatted_string = "Template {}".format(value)
In the syntax, the format()
method in Python takes values
as parameters to replace placeholders ({}
) in a string. The basic syntax includes the template string with placeholders and the values passed as arguments.
Example Code:
ostr = "Web"
nstr = "{}".format(ostr)
print(nstr)
Output:
Web
In this code, we initialize a string variable ostr
with the value "Web"
. To duplicate this string, we use the format()
method applied to an empty string containing a placeholder {}
, and inside the format()
method, we specify the original string ostr
as the value to replace the placeholder.
Subsequently, we assign the resulting formatted string to a new variable, nstr
. Finally, we print the copied string nstr
to the console, and the output will be Web
, representing the copied content of the initial string.
Use f-string
to Copy a String in Python
The f-strings
were introduced in Python 3.6 and provide a concise way to create formatted strings. They are generally preferred for string formatting due to their simplicity and readability.
We can use f-strings
(formatted string literals) to copy a string in Python.
Basic Syntax:
copied_string = f"{original_string}"
When using an f-string
in Python, there isn’t a distinct concept of “parameters” in the same way as when using a function that takes parameters. Instead, values are directly embedded within the string.
Example Code:
ostr = "Web"
nstr = f"{ostr}"
print(nstr)
Output:
Web
In this code, we initialize a string variable ostr
with the value "Web"
. To create a copy of this string, we use an f-string
, denoted by the f"{ostr}"
syntax, and within the curly braces, we insert the value of the original string ostr
.
We then assign the resulting formatted string to a new variable, nstr
. Finally, we print the copied string nstr
to the console, and the output will be Web
, representing the copied content of the initial string.
Conclusion
In summary, this tutorial explored different methods to copy a string in Python, considering the immutability of strings. We discussed techniques such as concatenation, string slicing, the str()
function, and string formatting using %
and format()
.
Each method was illustrated with concise examples, showcasing how to duplicate string content. Whether through traditional approaches or modern features like f-strings
, this tutorial provides Python developers with a versatile toolkit for copying strings, ensuring flexibility and readability in their code.
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedIn