How to Change Dictionary Values in Python
- Accessing Dictionary Values
-
Using the
update()
Method - Changing Values with a Loop
- Using Dictionary Comprehensions
- Conclusion
- FAQ
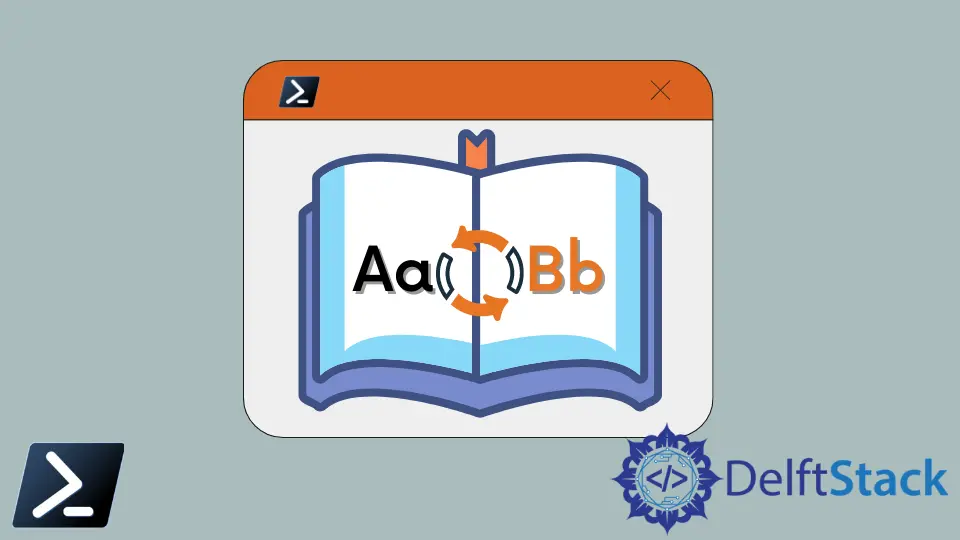
Dictionaries are one of the most versatile data structures in Python, allowing you to store and manipulate data in key-value pairs. Whether you’re working on a small script or a large application, knowing how to change dictionary values is essential.
In this tutorial, we’ll explore various methods to modify dictionary values in Python, providing clear examples and explanations along the way. By the end, you’ll be equipped with the knowledge to efficiently update your dictionaries, making your coding experience smoother and more effective. Let’s dive into the world of Python dictionaries and unlock their potential.
Accessing Dictionary Values
Before we can change dictionary values, it’s important to understand how to access them. In Python, you can access a dictionary’s value by referencing its key. For example, consider the following dictionary:
my_dict = {'name': 'Alice', 'age': 25, 'city': 'New York'}
print(my_dict['name'])
Output:
Alice
In this code snippet, we’re accessing the value associated with the key ’name’. The output shows ‘Alice’, which is the value stored under that key. This straightforward access method is the foundation for modifying values.
To change a value, simply assign a new value to the existing key:
my_dict['age'] = 26
print(my_dict['age'])
Output:
26
Here, we’ve updated the age from 25 to 26. This method is direct and efficient, making it a go-to approach for modifying dictionary values.
Using the update()
Method
Another effective way to change dictionary values is by using the update()
method. This method allows you to update multiple key-value pairs at once, making it particularly useful when you need to make several changes simultaneously. Here’s how it works:
my_dict = {'name': 'Alice', 'age': 25, 'city': 'New York'}
my_dict.update({'age': 26, 'city': 'Los Angeles'})
print(my_dict)
Output:
{'name': 'Alice', 'age': 26, 'city': 'Los Angeles'}
In this example, we called the update()
method on my_dict
and passed a new dictionary containing the keys and their updated values. As a result, both the ‘age’ and ‘city’ keys were modified. This method is particularly beneficial for bulk updates, making your code cleaner and more efficient.
Changing Values with a Loop
If you need to change values based on certain conditions, using a loop can be a powerful approach. For instance, if you want to increment the age of all individuals in a dictionary by one, you can use a loop to iterate through the dictionary and update the values conditionally. Here’s an example:
my_dict = {'Alice': 25, 'Bob': 30, 'Charlie': 35}
for key in my_dict:
my_dict[key] += 1
print(my_dict)
Output:
{'Alice': 26, 'Bob': 31, 'Charlie': 36}
In this code, we loop through each key in my_dict
and increment the corresponding value by one. This method is not only effective for updating values but also allows for more complex logic, such as applying conditions to decide whether to change a value or not. This flexibility is one of the many reasons Python is favored for data manipulation tasks.
Using Dictionary Comprehensions
Dictionary comprehensions provide a concise way to create new dictionaries by modifying existing ones. This method is particularly useful when you want to apply a transformation to all values in a dictionary. Here’s an example that demonstrates how to use dictionary comprehensions to change values:
my_dict = {'Alice': 25, 'Bob': 30, 'Charlie': 35}
new_dict = {key: value + 5 for key, value in my_dict.items()}
print(new_dict)
Output:
{'Alice': 30, 'Bob': 35, 'Charlie': 40}
In this example, we created a new dictionary called new_dict
where each value from my_dict
is incremented by 5. Dictionary comprehensions are not only elegant but also enhance code readability, allowing you to express complex transformations in a single line. This method is particularly effective when you want to create a modified version of an existing dictionary without altering the original.
Conclusion
In this tutorial, we explored several methods to change dictionary values in Python, including direct access, the update()
method, loops, and dictionary comprehensions. Each technique has its own advantages, and the best choice often depends on the specific use case and the complexity of the task at hand. By mastering these methods, you’ll be well-equipped to manipulate dictionaries effectively in your Python projects. Remember, dictionaries are powerful tools in your programming arsenal, and knowing how to modify them can significantly enhance your coding experience.
FAQ
-
How do I change a single dictionary value in Python?
You can change a single dictionary value by accessing the key directly and assigning a new value to it. -
What is the difference between using update() and direct assignment?
Theupdate()
method can modify multiple key-value pairs at once, while direct assignment is used for changing individual values. -
Can I change dictionary values based on conditions?
Yes, you can use loops to iterate through the dictionary and apply conditions to change values accordingly. -
What are dictionary comprehensions?
Dictionary comprehensions are a concise way to create new dictionaries by applying transformations to existing ones. -
Are there any performance considerations when changing dictionary values?
Generally, dictionary operations are efficient, but using the right method for your specific needs can optimize performance.