How to Capitalize the First Letter of a String in Python
-
Capitalize First Letter of String in Python Using the
capitalize()
Method -
Capitalize First Letter of String in Python Using the
title()
Method -
Capitalize First Letter of String in Python Using the
capwords()
Function -
Capitalize First Letter of String in Python Using the
regex
Method - Capitalize First Letter of String in Python Using the User-Defined Method
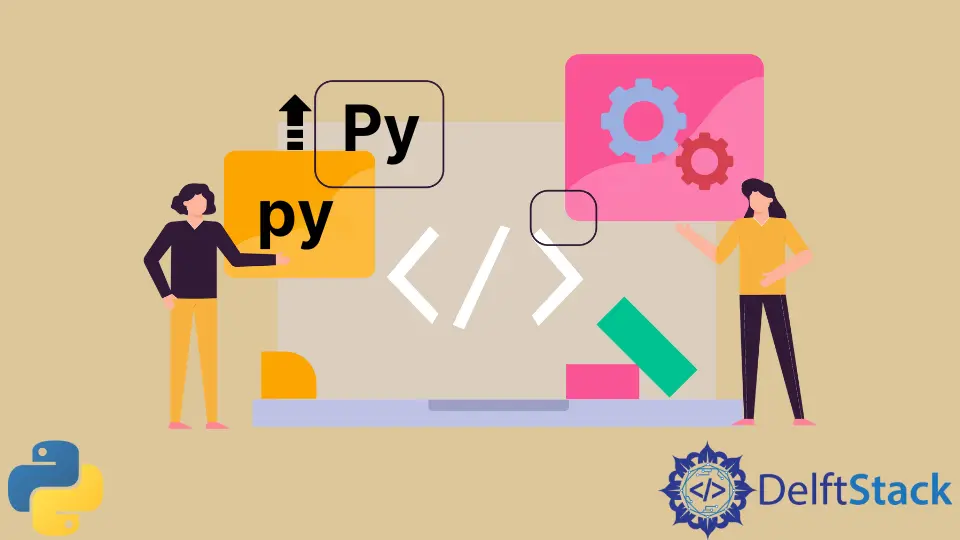
This article will discuss the methods to capitalize the first letter of the string in Python. We will also discuss the scenario when a digit is at the start of the string instead of a letter.
Capitalize First Letter of String in Python Using the capitalize()
Method
The string class’s capitalize()
method capitalizes the first character while the remaining characters are lower-case. It does nothing if the first character is already in the upper-case.
The complete example code is given below.
string = "learn Python"
cap_string = string.capitalize()
print("The capitalized string is:", cap_string)
Output:
The capitalized string is: Learn python
If the first character in the string is a digit, it will not capitalize the first letter. To solve this problem, we can use the isdigit()
function.
The complete example code to use the isdigit()
function is given below.
string = "5learn python"
for i, c in enumerate(string):
if not c.isdigit():
break
cap_string = string[:i] + string[i:].capitalize()
print("The capitalized string is:", cap_string)
Output:
The capitalized string is: 5Learn python
The enumerate()
function provides an iterable counter of the string and returns its enumerated object. The isdigit()
checks if the character is a digit or not. It breaks the for
loop when it encounters the first non-digit character.
The string[:i]
is the substring of the leading digits, and string[i:].capitalize()
converts the first letter of the remaining string to the upper case.
Capitalize First Letter of String in Python Using the title()
Method
The title()
method enables each word title string-cased. It means that each word’s first character is converted to upper-case, and the remaining word characters are converted to lower-case.
The complete example code is given below:
string = "learn python"
cap_string = string.title()
print("The capitalized string is:", cap_string)
Output:
The capitalized string is: Learn Python
This function will capitalize the first letter of each word in the string no matter the digit is present at the start of the word.
Capitalize First Letter of String in Python Using the capwords()
Function
It is the function of the string
module. It breaks the string into words and rejoins them using a specified separator after capitalizing each word. The default separator is the white space.
The complete example code is given below:
import string
strng = "learn python"
cap_strng = string.capwords(strng)
print("The capitalized string is:", cap_strng)
Output:
The capitalized string is: Learn Python
Capitalize First Letter of String in Python Using the regex
Method
This method will also capitalize the first letter of every word in the string while all remaining characters are lower-case.
The complete example code is given below:
import re
string = "learn python"
string = re.sub("([a-zA-Z])", lambda x: x.groups()[0].upper(), string, 1)
print("The capitalized string is:", string)
Output:
The capitalized string is: Learn python
The sub()
function of Python’s regular expression module replaces the string pattern [a-zA-Z]
, a lower case or upper case alphabet, in the given string with the return value of the lambda function.
lambda x: x.groups()[0].upper()
converts the first matched group in the regular expression, the first alphabet in this example, to the upper-case. x
is the matched object here.
We need to capitalize the first letter; therefore, the count
in the re.sub()
function is set to be 1 to make the replacement only once.
Capitalize First Letter of String in Python Using the User-Defined Method
We can also make a user-defined function to capitalize the first letter of the string. We will find the index, i
, of the first alphabet and apply the upper()
method to the substring string[:i]
. It capitalizes the first alphabet of the string even if the leading digits exist.
The complete example code is given below.
def check_alphabets(strng):
i = 0
for c in strng:
if c.isalpha():
i = i + 1
return i
i = i + 1
def unchanged_characters(strng):
i = check_alphabets(strng)
return strng[:i].upper() + strng[i:]
strng = "0learn1python"
cap_string = unchanged_characters(strng)
print("The capitalized string is:", cap_string)
Output:
The capitalized string is: 0Learn1python