How to Capitalize First Letter of Each Word in Python
-
Capitalize First Letter of Each Word in Python Using the
string.title()
Method -
Capitalize Each Word in Python Using the
string.capwords()
Method -
Capitalize Each Word in Python Using
string.split()
andstring.join()
Methods
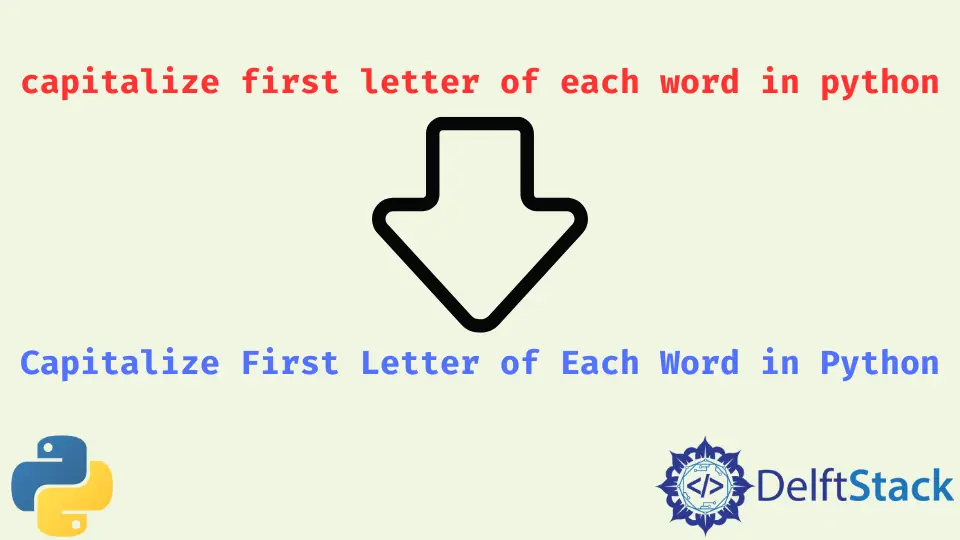
This tutorial will explain various methods to capitalize the first letter of each word in the string in Python. If we have a string like hey! what's up?
and we want to convert it to Hey! What's Up?
. We can do so by either using some available method or separating each word of the string, capitalizing the first letter of each word, and then joining the string back together.
We may also have strings like hey... what's up?
and we want to keep the original spacing of the string. This tutorial will look into the various methods to solve these issues.
Capitalize First Letter of Each Word in Python Using the string.title()
Method
The string.title()
is a built-in method that takes a string as input and returns the string with each word’s capitalized the first character. The string.title()
method does not change the original spacing of the string. The code example below demonstrates how to use the string.title()
method to capitalize each letter of a word in Python.
string = "hey! what's up?"
print(string)
print(string.title())
Output:
Hey! What's up?
Hey! What'S Up?
As can be noticed in the above example, the string.title()
method does not work well with the punctuation marks, as it capitalizes the letter after the punctuation marks.
Capitalize Each Word in Python Using the string.capwords()
Method
The string.capwords(string, sep)
of the string
module takes the string as the first parameter and the sep
as the second parameter and returns the string with the capitalized first character of each word, separated on the base of the sep
parameter. If no value is passed to the sep
parameter or set to None
, the whitespaces will be used as the separator, and a single whitespace replaces one or more whitespaces.
The example code below shows how to use the string.capwords()
method to capitalize each word of the string:
import string
mystring = "hey! what's up?"
print(mystring)
print(string.capwords(mystring))
Output:
hey! what's up?
Hey! What's Up?
The problem with this method is that it will either miss words like 'hello'
or if we pass the '
as the sep
parameter, it will capitalize what's
to what'S
, as shown in the example code below:
import string
mystring = "'hello' what's up?"
print(mystring)
print(string.capwords(mystring))
print(string.capwords(mystring, sep="'"))
Output:
'hello' what's up?
'hello' What's Up?
'Hello' what'S up?
Capitalize Each Word in Python Using string.split()
and string.join()
Methods
The string.split(separator,..)
method converts the string into a list by using the provided value of the separator
parameter as the separator. The string.join(iterable)
method takes the iterable object as input and converts it into a string using the provided string argument as the word separator.
The below example code demonstrates how to use the string.split()
and string.join()
methods to capitalize the first letter of each word in Python.
import re
s = "'hello' what's up?"
print(s)
slist = []
for word in s.split():
if word[0] in ("'", '"', "("):
word = word[0] + word[1].upper() + word[2:]
slist.append(word)
else:
word = word[0].upper() + word[1:]
slist.append(word)
new_string = " ".join(slist)
print(new_string)
Output:
'hello' what's up?
'Hello' What's Up?
As can be noticed in the above example, this method can handle the quotations, possessive nouns, and the words between the parenthesis. But the original spacing of the string will be lost in this method.