How to Check if Two Strings Are Anagrams Using Python
- Check if Two Strings Are Anagrams Using Sorting in Python
- Check if Two Strings Are Anagrams Using Frequency Dictionaries in Python
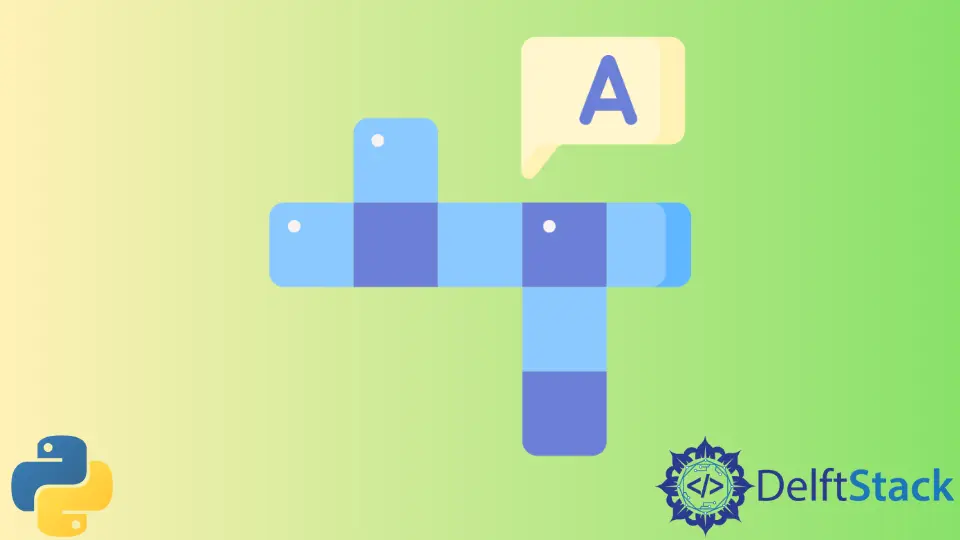
An anagram is a word formed via rearranging the letters of some other word.
For example, race
and care
, listen
, and silent
, fried
and fired
, knee
and keen
are some pairs of anagrams. dad
and bad
, apple
and mango
, hello
and world
are not anagrams.
Using programming languages, we can quickly check if two strings are anagrams of each other or not.
This article will show how to check if two strings are anagrams or not using Python. We will talk about a few approaches for the same.
Check if Two Strings Are Anagrams Using Sorting in Python
To check if two strings are anagrams or not, we can sort the two strings and check if they are equal or not. Refer to the following code for the same.
The time complexity of the code is O(nlogn)
because of sorting, and space complexity is O(1)
, because we are not storing any value.
def check_anagram(a, b):
if a is None or b is None or len(a) != len(b):
return "Not Anagram"
return "Anagram" if sorted(a) == sorted(b) else "Not Anagram"
print(check_anagram("keen", "knee"))
print(check_anagram("race", "care"))
print(check_anagram("fried", "fired"))
print(check_anagram("apple", "paddle"))
print(check_anagram("first", "second"))
print(check_anagram(None, "second"))
print(check_anagram("first", None))
print(check_anagram(None, None))
Output:
Anagram
Anagram
Anagram
Not Anagram
Not Anagram
Not Anagram
Not Anagram
Not Anagram
Check if Two Strings Are Anagrams Using Frequency Dictionaries in Python
To check if two strings are anagrams, we can keep the count of characters present in both the strings and compare the counts. If they would be the same, this means that the two strings are anagrams. Otherwise, they are not.
Refer to the following code for the same.
The time complexity of the following solution is O(n)
since we are iterating over the two strings. The average time complexity of adding an element to the dictionary and retrieving an element is O(1)
.
Moreover, comparing two dictionaries with the same number of keys is O(n)
. And, the space complexity is O(n)
because we are maintaining two dictionaries, and behind the scenes, dictionaries use arrays to store keys and values.
def check_anagram(a, b):
if a is None or b is None or len(a) != len(b):
return "Not Anagram"
counts_a = {}
counts_b = {}
for x in a:
if x not in counts_a.keys():
counts_a[x] = 1
else:
counts_a[x] += 1
for x in b:
if x not in counts_b.keys():
counts_b[x] = 1
else:
counts_b[x] += 1
return "Anagram" if counts_a == counts_b else "Not Anagram"
print(check_anagram("keen", "knee"))
print(check_anagram("race", "care"))
print(check_anagram("fried", "fired"))
print(check_anagram("apple", "paddle"))
print(check_anagram("first", "second"))
print(check_anagram(None, "second"))
print(check_anagram("first", None))
print(check_anagram(None, None))
Output:
Anagram
Anagram
Anagram
Not Anagram
Not Anagram
Not Anagram
Not Anagram
Not Anagram