2D Dictionary in Python
- Create a 2D Dictionary in Python by Nesting Dictionaries
- Create a 2D Dictionary in Python by Adding Key-Value Pairs
-
Create a 2D Dictionary in Python Using
defaultdict()
- Create a 2D Dictionary in Python Using a Loop
- Create a 2D Dictionary in Python Using Nested Braces
- Conclusion
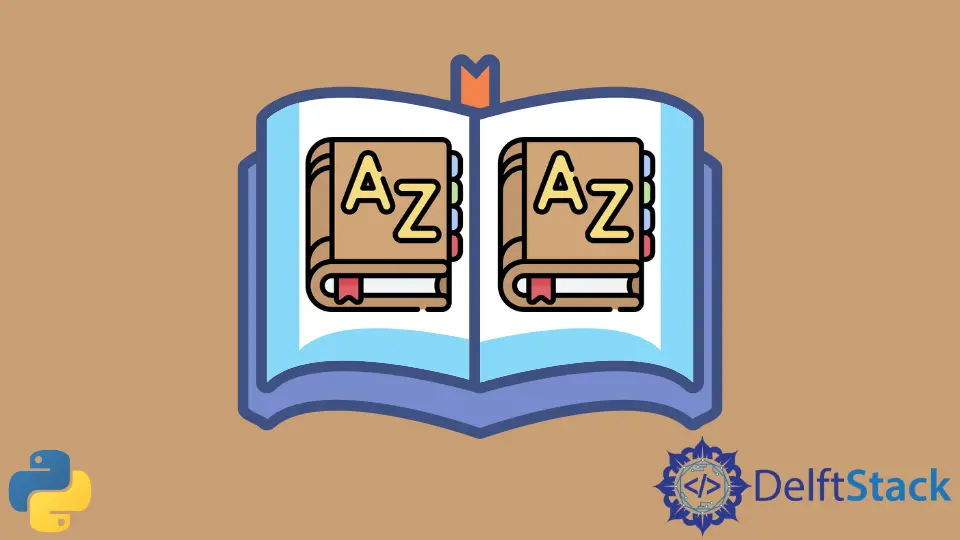
A two-dimensional dictionary, often likened to a grid or table, is a valuable tool for organizing data in a structured manner. Python offers multiple techniques to create such dictionaries, allowing developers to choose the method that aligns with their coding style and project requirements.
This article will walk you through the process of creating a two-dimensional dictionary using nested braces, defaultdict
, a loop, and a dict comprehension. Each method will be explained in detail, providing a holistic understanding of how to efficiently structure and manage data in a two-dimensional format.
Create a 2D Dictionary in Python by Nesting Dictionaries
One approach to creating a two-dimensional dictionary is by nesting dictionaries within a parent dictionary. This technique provides a clear and structured way to represent information, especially when dealing with rows and columns of data.
The idea behind nesting dictionaries is to have a primary dictionary where each key corresponds to a row or a category, and the associated values are dictionaries representing the columns or attributes. Each column dictionary contains key-value pairs specific to that row, forming a comprehensive two-dimensional structure.
Let’s take a look at the example code below:
import json
twoD_dict = dict()
twoD_dict["row1"] = {"name": "Alice", "age": 25, "city": "New York"}
twoD_dict["row2"] = {"name": "Bob", "age": 30, "city": "San Francisco"}
twoD_dict["row3"] = {"name": "Charlie", "age": 22, "city": "Los Angeles"}
print(json.dumps(twoD_dict, sort_keys=True, indent=2))
In this example, we start by initializing an empty dictionary, twoD_dict
, which will serve as the primary container for our two-dimensional structure.
twoD_dict = dict()
Next, we add nested dictionaries to the primary dictionary, with each key-value pair representing a row. In this case, the rows are labeled as row1
, row2
, and row3
, and each row has attributes such as name
, age
, and city
.
twoD_dict["row1"] = {"name": "Alice", "age": 25, "city": "New York"}
twoD_dict["row2"] = {"name": "Bob", "age": 30, "city": "San Francisco"}
twoD_dict["row3"] = {"name": "Charlie", "age": 22, "city": "Los Angeles"}
To visualize the two-dimensional dictionary’s structure, we use json.dumps()
to print it in a readable JSON format.
print(json.dumps(twoD_dict, sort_keys=True, indent=2))
Output:
{
"row1": {
"age": 25,
"city": "New York",
"name": "Alice"
},
"row2": {
"age": 30,
"city": "San Francisco",
"name": "Bob"
},
"row3": {
"age": 22,
"city": "Los Angeles",
"name": "Charlie"
}
}
As we can see, the output shows the organized two-dimensional structure, where a key represents each row, and the associated values are dictionaries containing the attributes of that row. This approach provides a convenient and intuitive way to handle tabular data in Python.
Create a 2D Dictionary in Python by Adding Key-Value Pairs
Another approach to constructing a two-dimensional dictionary in Python involves adding key-value pairs incrementally. This method allows us to build the dictionary dynamically, creating rows and columns as needed.
Creating a two-dimensional dictionary by adding key-value pairs involves starting with an empty main dictionary and progressively adding nested dictionaries along with their corresponding keys. Each nested dictionary represents a row or category, and individual key-value pairs within those dictionaries denote specific columns and their associated values.
This method is particularly useful when the structure of the dictionary is not predefined and needs to be built based on dynamic requirements.
Let’s see the code example demonstrating how to create a two-dimensional dictionary in Python by adding key-value pairs:
import json
twoD_dict = {}
twoD_dict["row1"] = {}
twoD_dict["row1"]["col1"] = 10
twoD_dict["row1"]["col2"] = 20
twoD_dict["row1"]["col3"] = 30
twoD_dict["row2"] = {}
twoD_dict["row2"]["col1"] = 40
twoD_dict["row2"]["col2"] = 50
twoD_dict["row2"]["col3"] = 60
twoD_dict["row3"] = {}
twoD_dict["row3"]["col1"] = 70
twoD_dict["row3"]["col2"] = 80
twoD_dict["row3"]["col3"] = 90
print(json.dumps(twoD_dict, sort_keys=True, indent=2))
In this example, we start by importing the json
module for neat printing. We then initialize an empty dictionary, twoD_dict
. Subsequently, we add key-value pairs to this dictionary incrementally.
Each row
key is associated with an empty dictionary, and within each of these empty dictionaries, we add key-value pairs representing the columns (col1
, col2
, and col3
) along with their corresponding values.
The use of json.dumps
is optional but enhances the clarity of the output by presenting the two-dimensional dictionary in a well-formatted structure.
Output:
{
"row1": {
"col1": 10,
"col2": 20,
"col3": 30
},
"row2": {
"col1": 40,
"col2": 50,
"col3": 60
},
"row3": {
"col1": 70,
"col2": 80,
"col3": 90
}
}
This output showcases the successful creation of a two-dimensional dictionary using the incremental addition of key-value pairs. Rows and columns are clearly defined, providing a flexible and dynamic approach to constructing the dictionary structure.
Create a 2D Dictionary in Python Using defaultdict()
In Python, the defaultdict
from the collections
module provides a convenient way to create a two-dimensional dictionary by automatically initializing nested dictionaries for each key. This approach simplifies the code and ensures that nested dictionaries are present, reducing the need for explicit initialization.
The defaultdict
takes a factory function as an argument, and in our case, we’ll use dict
as the factory function. This means that each key in the main dictionary will automatically be associated with an empty dictionary as its default value.
twoD_dict = defaultdict(dict)
When we access a non-existent key, defaultdict
creates a new entry with the specified default value.
Below is a code example demonstrating how to create a two-dimensional dictionary in Python using defaultdict()
:
from collections import defaultdict
import json
twoD_dict = defaultdict(dict)
twoD_dict["row1"]["col1"] = 10
twoD_dict["row1"]["col2"] = 20
twoD_dict["row1"]["col3"] = 30
twoD_dict["row2"]["col1"] = 40
twoD_dict["row2"]["col2"] = 50
twoD_dict["row2"]["col3"] = 60
twoD_dict["row3"]["col1"] = 70
twoD_dict["row3"]["col2"] = 80
twoD_dict["row3"]["col3"] = 90
print(json.dumps(twoD_dict, sort_keys=True, indent=2))
Here, we begin by importing defaultdict
from the collections
module and json
for neat printing. We then create a defaultdict
named twoD_dict
with the default factory function set to dict
. This ensures that any new key automatically gets associated with an empty dictionary.
We proceed to add key-value pairs to the two-dimensional dictionary, and because of the use of defaultdict
, we don’t need to explicitly initialize nested dictionaries for each key. The structure is dynamically created as we add key-value pairs.
The json.dumps
method is utilized for clear and formatted printing, though it is optional.
Output:
{
"row1": {
"col1": 10,
"col2": 20,
"col3": 30
},
"row2": {
"col1": 40,
"col2": 50,
"col3": 60
},
"row3": {
"col1": 70,
"col2": 80,
"col3": 90
}
}
This output illustrates the successful creation of a two-dimensional dictionary using defaultdict()
, showcasing its simplicity and efficiency in managing nested dictionaries.
Create a 2D Dictionary in Python Using a Loop
Creating a two-dimensional dictionary in Python using a loop offers a flexible and efficient way to build the structure dynamically. This approach is particularly useful when dealing with larger datasets or when the size of the dictionary is not known beforehand.
The idea behind using a loop is to iterate over rows and columns, populating the two-dimensional dictionary incrementally. We define lists for rows and columns and then use nested loops to iterate over these lists, adding key-value pairs to the dictionary.
This method provides a systematic and organized way to construct the two-dimensional structure.
Let’s take a look at a code example that demonstrates how to create a two-dimensional dictionary in Python using a loop:
import json
rows = ["row1", "row2", "row3"]
cols = ["col1", "col2", "col3"]
twoD_dict = {row: {col: None for col in cols} for row in rows}
twoD_dict["row1"]["col1"] = 10
twoD_dict["row1"]["col2"] = 20
twoD_dict["row1"]["col3"] = 30
twoD_dict["row2"]["col1"] = 40
twoD_dict["row2"]["col2"] = 50
twoD_dict["row2"]["col3"] = 60
twoD_dict["row3"]["col1"] = 70
twoD_dict["row3"]["col2"] = 80
twoD_dict["row3"]["col3"] = 90
print(json.dumps(twoD_dict, sort_keys=True, indent=2))
In this example, we start by defining lists for rows (rows
) and columns (cols
). We then use a dictionary comprehension inside another dictionary comprehension to initialize the two-dimensional dictionary (twoD_dict
). The outer loop iterates over rows, and the inner loop iterates over columns, creating a nested structure of dictionaries.
We proceed to populate the dictionary with values, assigning specific values to each key within the nested dictionaries. This process allows for a clear and organized creation of the two-dimensional structure using a loop. The json.dumps
method is applied for clear and formatted printing.
Output:
{
"row1": {
"col1": 10,
"col2": 20,
"col3": 30
},
"row2": {
"col1": 40,
"col2": 50,
"col3": 60
},
"row3": {
"col1": 70,
"col2": 80,
"col3": 90
}
}
This output illustrates the successful creation of a two-dimensional dictionary using a loop, emphasizing its flexibility and suitability for scenarios where the dictionary structure needs to be built dynamically.
Create a 2D Dictionary in Python Using Nested Braces
Nesting dictionaries is a straightforward and intuitive approach to creating a two-dimensional dictionary in Python. This method involves directly embedding dictionaries within the main dictionary, providing a clear and concise structure.
The concept behind using nested braces is to declare a dictionary within another dictionary. Each outer key represents a row or category, and its corresponding value is another dictionary containing key-value pairs for columns and their associated values.
This nesting of dictionaries allows for a clean and hierarchical representation of the two-dimensional structure.
Let’s have an example showcasing how to create a two-dimensional dictionary in Python using nested braces:
import json
twoD_dict = {
"row1": {"col1": 10, "col2": 20, "col3": 30},
"row2": {"col1": 40, "col2": 50, "col3": 60},
"row3": {"col1": 70, "col2": 80, "col3": 90},
}
print(json.dumps(twoD_dict, sort_keys=True, indent=2))
Here, we start by initializing the two-dimensional dictionary (twoD_dict
) directly with nested braces. Each outer key, such as row1
, row2
, and row3
, is associated with a nested dictionary containing key-value pairs for columns (col1
, col2
, and col3
) and their respective values.
Output:
{
"row1": {
"col1": 10,
"col2": 20,
"col3": 30
},
"row2": {
"col1": 40,
"col2": 50,
"col3": 60
},
"row3": {
"col1": 70,
"col2": 80,
"col3": 90
}
}
This output demonstrates the successful creation of a two-dimensional dictionary using nested braces, emphasizing the simplicity and clarity of this approach for structuring data.
Conclusion
In conclusion, the creation of a two-dimensional dictionary in Python is a versatile task, and the choice of method depends on the specific needs of your project. Whether you prioritize simplicity, automatic initialization, dynamic creation, or concise syntax, the methods discussed in this article allow you to organize and manage data efficiently.
Select the approach that best aligns with your coding preferences and project requirements, and leverage the flexibility of Python to enhance the clarity and functionality of your code.