How to Print Unicode Characters in Python
- Method 1: Using Unicode Escape Sequences
-
Method 2: Using the
chr()
Function - Method 3: Using Unicode Names
- Additional Tips
- Conclusion
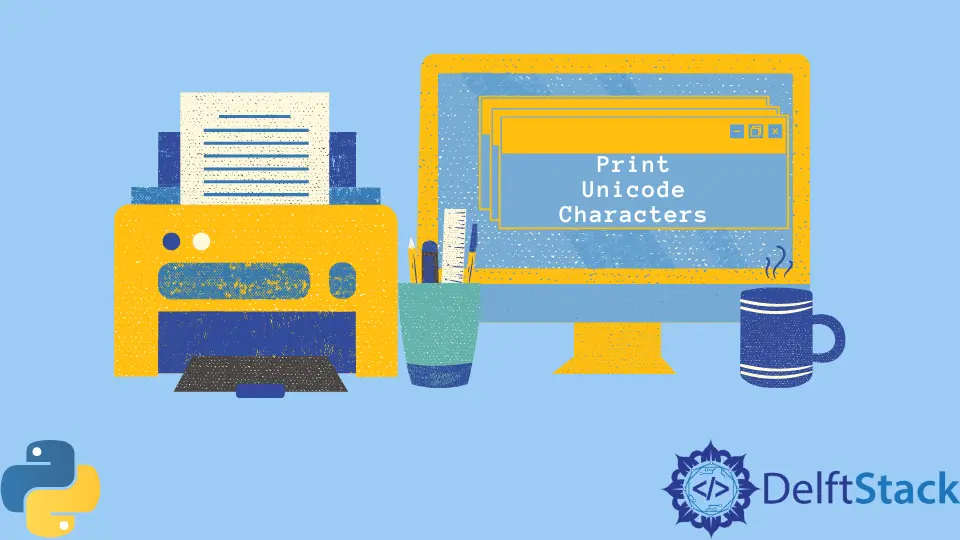
Unicode Character is a specified character assigned to a unique character used by the user in human language that gives a unique character of its own. Unicode character has a widespread acceptance in the world of programming.
Unicode characters play a crucial role in data representation, allowing developers to work with a vast array of symbols and characters from various languages and domains. In Python, handling Unicode characters is straightforward, thanks to its built-in support for Unicode. In this enhanced guide, we will explore multiple methods to print Unicode characters, ensuring you have a variety of options at your disposal.
The specified code or unique character to every human value character is called a code point. Ranging from U+0000
to U+10FFFF
the code points are in the form of hexadecimal digits. Each hexadecimal digit has its own Unicode character.
In Python, Unicode characters are represented as a string type. These characters are printed using the print
command.
Before giving the hexadecimal value as an input value, the escape sequence \u
is used before every hexadecimal value.
Method 1: Using Unicode Escape Sequences
Python supports Unicode escape sequences, enabling you to include Unicode characters within strings effortlessly. Precede the Unicode code point with \u
for basic characters or \U
for extended characters.
Example:
unicode_character = "\u2612\u7687\u00C9\u00C9"
print(unicode_character)
Output:
☒皇ÉÉ
In this example, each \u
is followed by a Unicode code point in hexadecimal, representing a specific Unicode character.
Method 2: Using the chr()
Function
The chr()
function in Python is used to get the string representing a character whose Unicode code point is the integer parameter.
Example:
unicode_character = chr(0x2612) + chr(0x7687) + chr(0x00C9) + chr(0x00C9)
print(unicode_character)
Output:
☒皇ÉÉ
Here, chr()
converts each integer Unicode code point to its corresponding character.
Method 3: Using Unicode Names
Python also allows the use of Unicode names. The unicodedata
module provides a function named lookup()
that returns the character whose name is passed as a parameter.
Example:
import unicodedata
unicode_character = unicodedata.lookup('BALLOT BOX WITH X') + \
unicodedata.lookup('CJK UNIFIED IDEOGRAPH-7687') + \
unicodedata.lookup('LATIN CAPITAL LETTER E WITH ACUTE') + \
unicodedata.lookup('LATIN CAPITAL LETTER E WITH ACUTE')
print(unicode_character)
Output:
☒皇ÉÉ
Additional Tips
- Ensure your text editor or IDE supports Unicode characters to display them correctly.
- Always refer to the official Unicode character table to find the correct code points or names for the characters you wish to use.
Conclusion
Printing Unicode characters in Python is a straightforward task, thanks to the language’s robust support for Unicode. Whether you’re using escape sequences, the chr()
function, or Unicode names with the unicodedata
module, Python offers a variety of methods to suit your needs. Always ensure to test your code thoroughly to confirm the correct display of Unicode characters in your application’s output.
Lakshay Kapoor is a final year B.Tech Computer Science student at Amity University Noida. He is familiar with programming languages and their real-world applications (Python/R/C++). Deeply interested in the area of Data Sciences and Machine Learning.
LinkedIn