How to Find Character in a String in Python
-
Using the
find()
Function to Find the Position of a Character in a String -
Using the
rfind()
Function to Find the Position of a Character in a String -
Using the
index()
Function to Find the Position of a Character in a String -
Using the
for
Loop to Find the Position of a Character in a String - Faq
- Conclusion
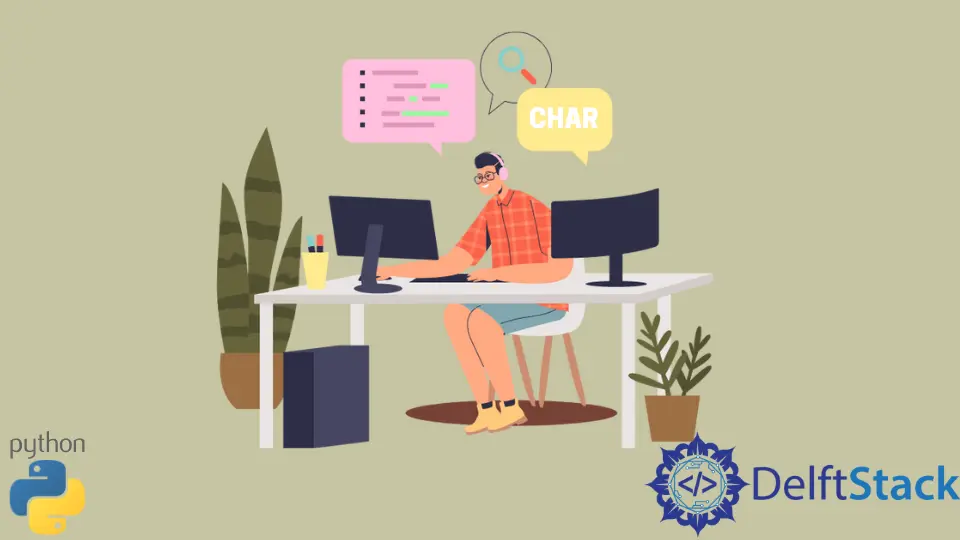
In Python, determining the position of characters within a string is crucial, especially when working with data science and string manipulation. The significance lies in employing string methods to efficiently locate the index position of a character in a given string.
The method takes the character to search for as its first parameter, the start parameter to specify where to start searching, and the third parameter to indicate the end position. The return value is the index position of the first match, considering the case sensitivity of characters.
This information is valuable for tasks such as printing characters, identifying the last character in a string, or finding the index of a substring in tuple format. The ability to pinpoint the position of characters within a string is fundamental for various applications and simplifies tasks involving string length and substring presence.
This tutorial delves into techniques for finding the position of a character in a Python string. The tutorial provides code examples and corresponding outputs, offering a comprehensive guide to character position identification in Python strings.
Using the find()
Function to Find the Position of a Character in a String
In Python, the find()
method is used to locate the position of a specified substring or character within a string.
The find()
method in Python is used to find the position of a specified substring or character within a string. Here is the general syntax:
string.find(substring, start, end)
The method takes three parameters: the string to search, the substring or character to find, and optional starting and ending indices for the search.
The starting index (optional) denotes the position from which the search begins, and if not provided, it starts from the beginning of the string. The ending index (optional) indicates where the search stops, and if not given, it goes up to the highest index in the string.
The method returns the position of the first occurrence of the specified character in the string, and if the character is not found, it returns -1
.
This function is useful for identifying where a character or substring occurs within strings, and it is particularly handy for tasks like character printing or checking the presence of a substring inside a given range of indices in a string.
In the following code, we will use this function to find the position of a character in a string.
Code Input:
s = "python is fun"
c = "n"
print(s.find(c))
The variable s
is assigned the string python is fun
, and the variable c
is assigned the character n
. The find()
function is then applied to the string s
with the argument c
, which means it searches for the first occurrence of the character n
within the string.
Code Output:
The output of print(s.find(c))
will be 5
. This is because the character n
first appears at index 5
in the string python is fun
when counting from the left, and the find()
function returns the index of the first occurrence. It’s important to note that the indexing is zero-based, so the first position of the character has an index of 0
, the second has an index of 1
, and so on.
Using the rfind()
Function to Find the Position of a Character in a String
In Python, the rfind()
method helps find the highest index of a specified substring or character within a string, searching from right to left. The function takes three parameters: the string to search, the substring or character to find, and optional starting and ending indices for the search.
string.rfind(substring, start, end)
The starting index (optional) indicates the position from where the search begins, defaulting to the end of the string if not provided. The ending index (optional) specifies where the search ends, defaulting to the beginning of the string if not given.
The method returns the position of the last occurrence of the specified character in the string, and if not found, it returns -1
. This function is useful for tasks like character printing or checking where a substring occurs within strings, especially when searching from the end.
Code Input:
s = "python is fun"
c = "n"
print(s.rfind(c))
The variable s
is assigned the string python is fun
, and the variable c
is assigned the character n
.
The rfind()
function is then applied to the string s
with the argument c
. The rfind()
function searches for the last occurrence of the character n
within the string, starting the search from the end (right to left).
Code Output:
The output of print(s.rfind(c))
will be 12
. This is because the last occurrence of the character n
in the string python is fun
is at index 12
when counting from the left. The indexing is zero-based, so the first character has an index of 0
, the second has an index of 1
, and so on.
Using the index()
Function to Find the Position of a Character in a String
In Python, the index()
method is employed to locate the position of a specified substring or character within a string. The syntax involves three parameters: the string to search, the substring or character to find, and optional starting and ending indices for the search.
string.index(substring, start, end)
The starting index (optional) determines the position from which the search begins, defaulting to the beginning of the string if not provided. The end index (optional) specifies where the search ends, defaulting to the end of the string if not given.
This method works by returning the index of the first occurrence of the specified character in the string. It is important to note that the method is case-sensitive, and if the substring is not found, it raises a ValueError
exception.
The difference between index()
and find()
lies in how they handle a missing substring: index()
raises an exception, while find()
returns -1
. This distinction is crucial for error handling based on the outcome of the most recent call.
The function returns the index of the first occurrence of the specified item, and the search starts from the beginning of the string.
Code Input:
s = "python is fun"
c = "n"
print(s.index(c))
The variable s
is assigned the string python is fun
, and the variable c
is assigned the character n
.
The index()
function is then applied to the string s
with the argument c
. The index()
function searches for the first occurrence of the character n
within the string, starting the search from the left.
Code Output:
The output of print(s.index(c))
will be 5
. This is because the first occurrence of the character n
in the string python is fun
is at index 5
when counting from the left, with indexing starting from 0
.
The only difference between the index()
and find()
functions is that the index()
function returns ValueError
when the required character is missing from the string.
Using the for
Loop to Find the Position of a Character in a String
In Python, a for
loop is used for iterating over a sequence (such as a string, list, or tuple). It iterates through each element in the sequence, executing a block of code for each iteration.
By using a for
loop, we can note every occurrence of the character in a string. We can iterate through the string and compare each character individually.
Code Input:
s = "python is fun"
c = "n"
lst = []
for pos, char in enumerate(s):
if char == c:
lst.append(pos)
print(lst)
The code initializes an empty list lst
and iterates over each character in the string s
using a for
loop with the enumerate()
function, which provides both the position (pos
) and the character (char
) in each iteration.
If the current character (char
) matches the specified character (c
), the position (pos
) is appended to the list lst
. The final output, when printed, will be the list of positions where the character n
is found in the string python is fun
.
Code Output:
For this specific example, the output will be [5, 13]
, indicating that n
appears at positions 5
and 13
in the string.
We use the enumerate()
function because it makes the iteration easier and assigns a counter variable with every character of the string.
We can also implement this method using the list comprehension method, which is considered faster and cleaner.
Code Input:
s = "python is fun"
c = "n"
print([pos for pos, char in enumerate(s) if char == c])
The code uses a list comprehension with enumerate()
to iterate over all the characters in the string s
.
For each character, char
, and its corresponding position, pos
, it checks if the character is equal to the specified character c
. If there’s a match, it creates a list of positions where the character n
is found in the string.
Code Output:
The output, when printed, will be the list of positions where n
occurs in the string python is fun
. For this specific example, the output will be [5, 13]
.
Faq
Q1: How Do I Find a Character in a String in Python?
A1: To find the index of a character in a string in Python, you can use methods like find()
, rfind()
, or index()
. They return the index of the first occurrence of the character in the string, or -1
if not found.
Q2: What Is Index Position in Python?
A2: In Python, index position refers to the numerical position of an element in a sequence, such as a string or list. Indexing starts from 0
, with each subsequent position incremented by 1
.
Conclusion
In summary, the tutorial explores different methods to find the position of a character in a Python string.
It covers the find()
function for the first occurrence, rfind()
for the last occurrence, and index()
, which raises an error if not found. The tutorial also introduces a for
loop with enumerate()
and a concise list comprehension as efficient alternatives.
For additional insights into counting occurrences of a character in a string in Python, you may refer to a related tutorial available here.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn