How to Plot an Array in Python
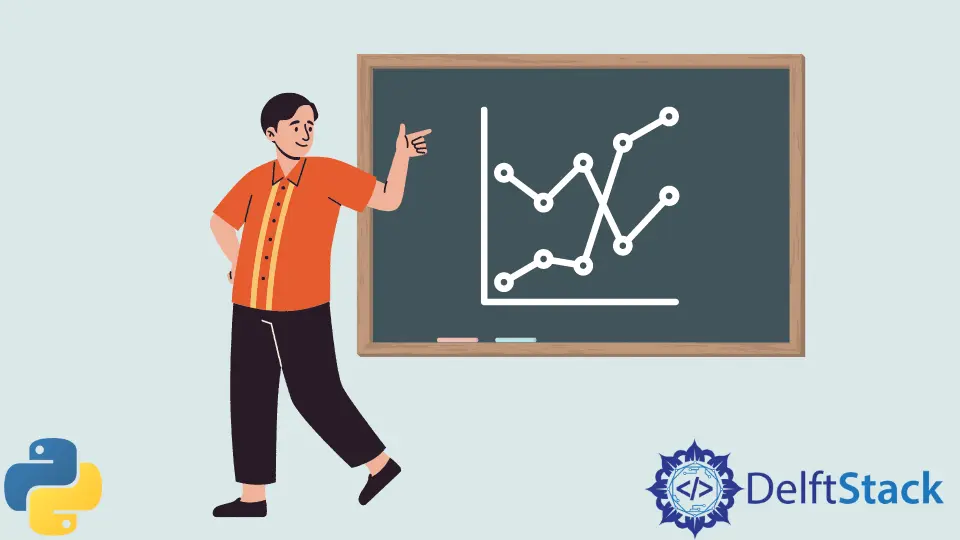
Visualizing data improves the general understanding of the data and helps with memory because humans tend to remember the visuals more than the texts. Visualization of data is crucial because we have a lot of data available to us, and we need a well-structured format to understand it. This activity is a part of the daily routine of every data scientist and machine learning enthusiast. They have to visualize data to understand it better and construct good and efficient machine learning models.
Often, data is represented in the form of arrays, and we end up in situations where we have to plot it. This article talks about how we can plot such data using Python.
Before we proceed with the code, we have to understand one thing. Arrays can be n-dimensional
, which means we can have arrays inside arrays (2-D arrays
), or arrays inside arrays that further have arrays inside them (3D arrays) so on. And as the number of dimensions increases, the complexity of plotting the arrays increases. In such cases, the arrays have to be converted into another format suitable for plotting or plotting some other kind of graph that makes visualization better. This article will talk about plotting 1D, and 2D arrays.
We will use Matplotlib
, a comprehensive python-based library for visualization purposes, and NumPy
to plot arrays.
You can read more about them here (Matplotlib
) and here (NumPy
).
Plot 1-D Arrays in Python
To plot a 1-dimensional array, refer to the following code.
import numpy as np
import matplotlib.pyplot as plt
x = np.array(range(0, 10))
y = np.array([100, 23, 44, 12, 55, 85, 69, 33, 75, 2])
plt.title("Plotting 1-D array")
plt.xlabel("X axis")
plt.ylabel("Y axis")
plt.plot(x, y, color="red", marker="o", label="Array elements")
plt.legend()
plt.show()
The variable y
holds the data that has to be plotted, and the variable x
holds the indexes. Here, for plotting purposes too, 0-based indexing has been considered. Note that one can also change the indexing back to 1-based indexing by add this x = np.array(range(1, 11))
. The plot
function first takes the data for the x-axis
and then for the y-axis
. The color
argument sets the color of the line plot, the marker
argument sets the symbol to be used for marking the points over the line graph, and the label
argument sets the label to be shown for this particular line. Note that, without plt.legend()
, no information about the label will be shown over the graph.
The output of the code above will look like this.
Plot 2-D Arrays in Python
To plot a 2-dimensional array, refer to the following code.
import numpy as np
import matplotlib.pyplot as plt
from matplotlib.pyplot import figure
figure(figsize=(8, 6))
x = np.array(range(0, 10))
y = np.array(
[
[32, 69, 92, 81, 27, 97, 64, 98, 26, 22],
[85, 76, 63, 44, 94, 52, 71, 66, 2, 62],
[45, 80, 46, 24, 10, 74, 10, 33, 12, 60],
[73, 94, 21, 6, 93, 74, 36, 47, 16, 99],
[96, 84, 66, 12, 43, 58, 17, 16, 28, 6],
[83, 4, 56, 94, 97, 36, 94, 41, 74, 14],
[90, 13, 11, 71, 79, 49, 9, 69, 73, 61],
[94, 52, 28, 18, 14, 15, 91, 95, 16, 42],
[73, 35, 40, 25, 46, 25, 33, 1, 99, 30],
[61, 7, 89, 33, 94, 64, 1, 60, 41, 81],
]
)
plt.title("Plotting a2-D array")
plt.xlabel("X axis")
plt.ylabel("Y axis")
for i, array in enumerate(y):
plt.plot(
x,
array,
color=np.random.rand(
3,
),
marker="o",
label=f"Array #{i}",
)
plt.legend(loc="center left", bbox_to_anchor=(1, 0.5))
plt.show()
The variable y
holds the 2-D
array. We iterate over each array of the 2-D
array, plot it with some random color and a unique label. Once the plotting is done, we reposition the legend box and show the plot.
The output of the code above will look like this.