Pickle Load in Python
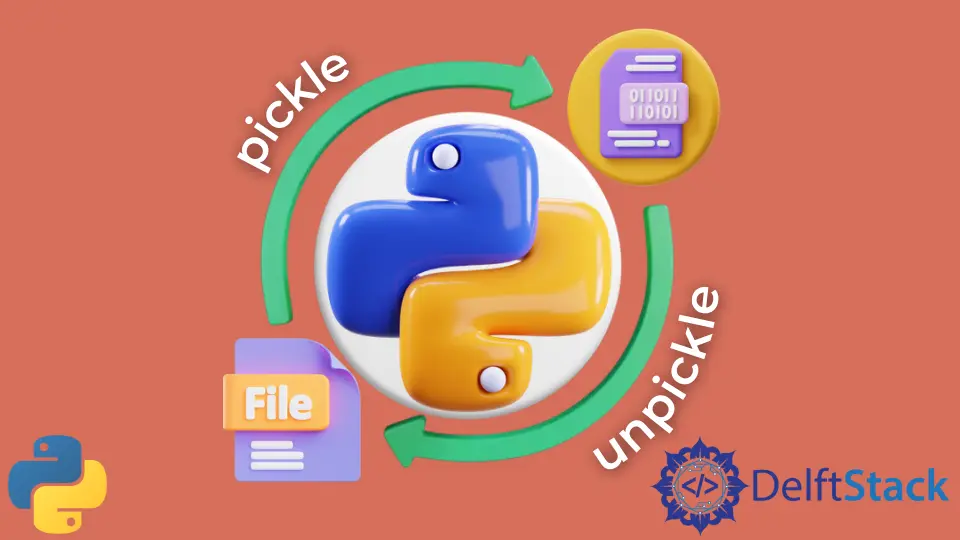
We will introduce the pickle
library in Python and how to use it in our Python applications.
Pickle in Python
As data scientists, we use data sets in data frames, dictionaries, and any other form of data type. While working on multiple data sets, we might need to save them in files for later use or to make it easy to share in the form of files.
Python provides a library pickle
that helps us in achieving this. Pickle
serializes the objects so they can be saved in a file and loaded in a program whenever we want.
We use pickle
when we want to serialize and de-serialize Python objects. Serialization is the process of converting an object to a byte stream that we can easily store on disk or share over a network.
With the help of pickle
, we can easily de-serialize the byte stream back to the Python object whenever we want.
Advantages of Pickle in Python
Pickle
is useful in applications if we want persistency in our data.- If we want to save the program’s state data into a disk to use afterward, we can use
pickle
. - We can also use
pickle
to send our data over TCP or socket connection or store the data in our database. - If you are a data scientist or working with machine learning algorithms where we want to save the data for predictions without rewriting or training the model repeatedly, we can use
pickle
.
Disadvantages of Pickle in Python
- Pickle’s protocol is specific to the Python language, so it cannot be used for cross-language applications.
- If we are trying to unpickle a file pickled in a different version of Python, it can cause problems.
- Unpickling data from untrusted sources can also cause problems.
- If there is some virus or malicious code inside the files that we are trying to unpickle, it can be executed and result in some problems.
Pickling Files in Python
Booleans, integers, floats, complex numbers, normal and Unicode strings, tuples, lists, sets, and dictionary data types can be picked up using Python’s pickle
library.
Let’s start with an example in which we will pickle a file. First, we will import the pickle
library as shown below.
# python
import pickle
Now let’s create a dictionary, save it to a file, and then load again.
# python
cats_dict = {"Tom": 5, "Oggy": 7, "Persia": 3, "Luna": 2}
To pickle this cat dictionary, we first need to specify the file’s name in which we have stored the dictionary. We can use the open()
function to open the file for writing.
The open()
function takes two arguments, the first will be the file name without extension, and the second will be the code for what we want to do with the file.
If we want to write the file in binary code, we will use wb
as the second argument. As shown below, w
stands for writing, and b
stands for binary mode.
# python
filename = "cats"
file = open(filename, "wb")
Once we have opened the file for writing, we can use pickle.dump()
, which takes in two arguments. The first will be the dictionary’s name, and the second argument will be the variable in which we have opened the file, as shown below.
# python
pickle.dump(cats_dict, file)
Now we will close the file.
# python
file.close()
This code will create a new file named cats
in the same directory containing the pickled data.
Output:
Pickle Load in Python
Now let’s unpickle the file that we just pickled using the method load()
. The load()
function comes in handy when we encounter an object that we have pickled in Python version 2, and now we are running Python 3.
It can be difficult and a hassle to unpickle. We can unpickle the file by running it in Python version 2, or we can do it using the encoding='latin1'
in the load()
function as shown below.
# python
filename = "cats"
unpickleFile = open(filename, "rb")
new_dict = pickle.load(unpickleFile, encoding="latin1")
If you have objects that contain NumPy
arrays, this method will not work. As shown below, we have to change the encoding from latin1
to bytes
.
# python
filename = "cats"
unpickleFile = open(filename, "rb")
new_dict = pickle.load(unpickleFile, encoding="bytes")
Output:
As you can see from the above example, we easily unpickled the file and got the correct results.
Summary
In this tutorial, we learned about the pickle
library; we also learned the advantages and disadvantages of pickle and when and when not to use the pickle. We learned how to pickle the file and unpickle the file using the load()
function.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn