How to Read a Pickle File Using Python
-
Read a Pickle File Using the
pickle
Module in Python -
Read a Pickle File Using the
pandas
Module in Python
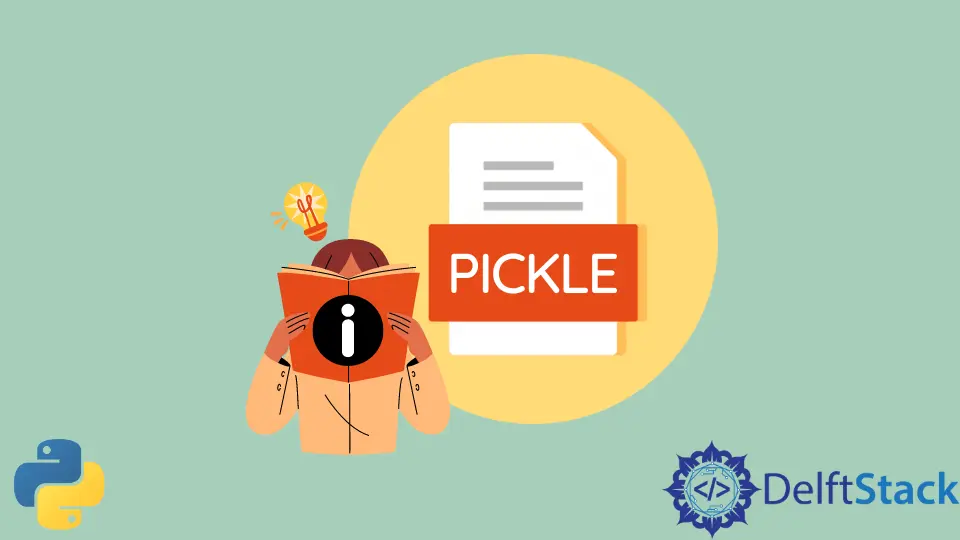
In Python, pickling refers to converting a Python object (lists, dictionaries, etc.) into a binary stream, and unpickling refers to converting a binary stream of data into a Python object.
The converted binary stream of data contains all the information to reconstruct the original object. Unfortunately, pickle files are generally considered unsafe.
Pickle files are used to save a program’s state (values of variables, objects, and their states, etc.), store Python objects to databases in the form of serialized binary strings, send data over TCP or Transmission Control Protocol, etc.
While training machine learning models, pickle files are used to store model weights, and sometimes, the loaded training data or the formatted training data is stored back to the disk in the form of pickle files.
In this article, we will get to understand how to read these pickle files using Python. We will discuss two such ways.
Read a Pickle File Using the pickle
Module in Python
Python has an in-built module, pickle
, that contains utilities for serializing and de-serializing data using Python. This data can be stored in pickle files.
We can use the pickle
module to read a pickle file using Python. Refer to the following Python code for the same.
objects = []
file_name = "/path/to/the/pickle/file"
with (open(file_name, "rb")) as f:
while True:
try:
objects.append(pickle.load(f))
except EOFError:
break
In the above code, the objects
variable will hold all the data of the pickle file.
The code loops over the file to read it until an EOFError
exception is found. The same is that the data is stored in objects inside a pickle file.
The load()
function from the pickle
module will only read a single object. After reading an object, the file pointer points to the beginning of the next object in the pickle file.
Refer to the documentation linked here to learn more.
Read a Pickle File Using the pandas
Module in Python
We can use the pandas
library to read a pickle file in Python.
The pandas
module has a read_pickle()
method that can be used to read a pickle file.
This method accepts a filepath_or_buffer
argument: the file path, the URL, or the buffer from where the pickle file will be loaded. This function will return an unpickled object of the file.
Now let us see how to use this method practically. Refer to the following Python code for the same.
import pandas as pd
file_name = "/path/to/the/pickle/file"
objects = pd.read_pickle(file_name)
To learn more about the
read_pickle()
method, refer to the official documentation here.