How to Pad String With Zeros in Python
-
Use the
zfill()
Method to Pad a String With Zeros in Python -
Use the
rjust()
orljust()
String Method to Pad a String With Zeros in Python -
Use the
format()
to Pad a String With Zeros in Python - Use the F-Strings to Pad a String With Zeros in Python
- Using String Concatenation to Pad a String With Zeros in Python
- Conclusion
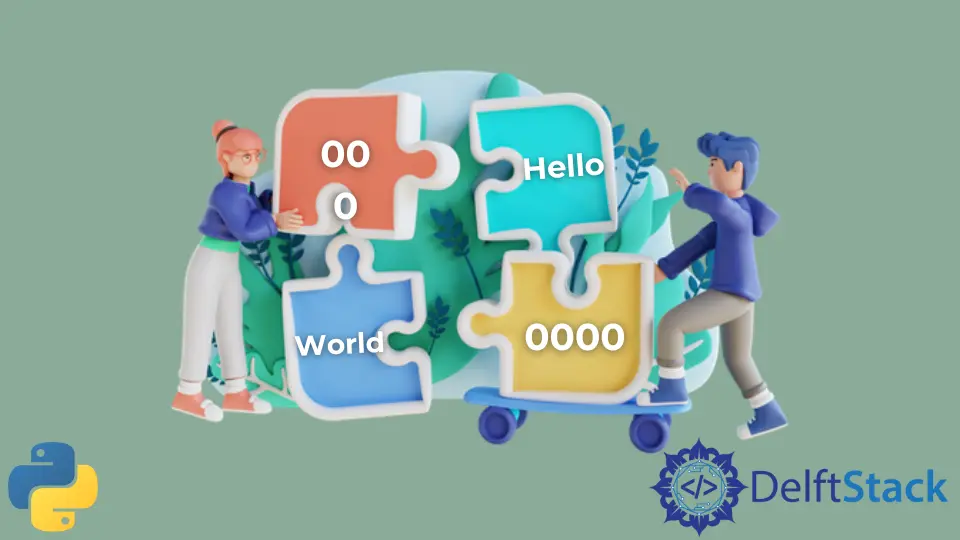
To make a string of the desired length, we add or pad a character at the beginning or the end of the string until it is of the required length. In this tutorial, we will discuss how to pad a string with zeros in Python.
Use the zfill()
Method to Pad a String With Zeros in Python
In Python, the zfill()
method accepts a numerical parameter specifying the desired length of the string and adds zeros to the left until the string reaches the desired length. If the number passed in as the parameter is smaller than the length of the original string, then there is no change.
Syntax:
str.zfill(width)
Parameter:
width
: The total width of the resulting string. The original string is padded with zeros to the left until the total width matches the specified value.
Code:
A = "007"
B = "1.000"
C = "4876"
print(A.zfill(5))
print(B.zfill(6))
print(C.zfill(3))
In the code, we have three strings, A
, B
, and C
, with values "007"
, "1.000"
, and "4876"
, respectively. The zfill()
method is applied to each string to pad zeros to the left until the specified width is met.
Output:
00007
01.000
4876
In the output, we can see that for A
(width 5), the output is 00007
. For B
(width 6), the output is 01.000
.
Lastly, for C
(width 3), since its length exceeds the specified width, the output remains 4876
.
Use the rjust()
or ljust()
String Method to Pad a String With Zeros in Python
The rjust()
or ljust()
method accepts a numerical parameter of the desired length of the string and a character as an optional parameter.
The rjust()
method adds the optional character to the left of the string until the string is of the desired length. Similarly, the ljust()
method adds the optional character to the right of the string until the string is of the desired length.
Basic Syntax - rjust()
& ljust()
:
# Using rjust() method
original_string.rjust(width, fillchar)
# Using ljust() method
original_string.ljust(width, fillchar)
In the syntax, the original_string
is the string we want to justify. The width
is the resulting justified string, including the original string and any additional padding.
The fillchar
(optional) is the character used for padding. If not specified, it defaults to space.
Code:
A = "Hello"
B = "World"
print(A.rjust(7, "0"))
print(B.ljust(8, "0"))
In the code, strings A
and B
, with values "Hello"
and "World"
, undergo padding operations. For A
, the rjust()
method right-justifies the string, adding zeros to the left until the width is 7
.
Meanwhile, for B
, the ljust()
method left-justifies the string by adding zeros to the right until the width is 8
.
Output:
00Hello
World000
For A
, it results in 00Hello
after adding zeros to the left until the width is 7
. For B
, it outputs World000
by adding zeros to the right until the width is 8
.
Use the format()
to Pad a String With Zeros in Python
The format()
method in Python is a built-in string method that is used for string formatting. It allows us to create formatted strings by inserting values into placeholders within a string.
We can use it to add zeros to the left of the string until the string is of the desired length. The format()
method can only be used for Python 2 to Python 3.5.
Syntax:
formatted_string = "String with placeholders {}".format(value1, value2, ...)
In the syntax, the curly braces {}
within the string act as placeholders. The format()
method is called on the string, and it replaces the placeholders with the specified values.
Code:
A = "Hello"
B = "CR7"
print("{:0>8}".format(A))
print("{:0>5}".format(B))
In this code, strings A
and B
("Hello"
and "CR7"
) undergo formatting using the format()
method.
Output:
000Hello
00CR7
For A
, zeros are added to the left, resulting in 000Hello
to meet the width of 8
. Similarly, for B
, zeros are added to the left, resulting in 00CR7
achieving a width of 5
characters.
Use the F-Strings to Pad a String With Zeros in Python
The f-strings
is an addition in recent versions of Python and provides quick formatting of strings. We can use it to pad a string with zeros.
This is similar to the format()
method.
Code:
A = "Hello"
B = "CR7"
print(f"{A:0>8}")
print(f"{B:0>5}")
In this code, strings A
and B
("Hello"
and "CR7"
) are formatted using f-strings
. Zeros are added to the left to achieve widths of 8
for A
(f"{A:0>8}"
), and 5
for B
(f"{B:0>5}"
).
Output:
000Hello
00CR7
In the output, we can see that the zeros added to the left to achieve widths of 8
for A
, resulting in 000Hello
, and 5
for B
, yielding 00CR7
.
Using String Concatenation to Pad a String With Zeros in Python
String concatenation is a technique in Python where two or more strings are combined to create a new string. When it comes to padding a string with zeros, string concatenation involves adding a specific number of zeros to the left or right of an existing string to achieve a desired length.
Code:
A = "Hello"
B = "World"
desired_length = 8
zeros_to_add_A = desired_length - len(A)
zeros_to_add_B = desired_length - len(B)
padded_A = "0" * zeros_to_add_A + A
padded_B = "0" * zeros_to_add_B + B
print(f"{padded_A} {padded_B}")
In this code, we work with string variables, A
and B
, initialized with values "Hello"
and "World"
. We introduce a variable, desired_length
, set to 8
.
Next, we calculate the number of zeros to add for each string by subtracting their current lengths from desired_length
. Using this information, we employ string concatenation to pad A
and B
with zeros to the left.
Output:
000Hello 000World
In the output, A
is displayed as 000Hello
, and B
is shown as 000World
, each padded with zeros to meet the specified length of 8
characters.
Conclusion
In this tutorial, we covered diverse methods for zero-padding strings in Python.
The zfill()
method emerged as a straightforward solution for left-padding with zeros. Alternatives like rjust()
and ljust()
offered flexibility in justification.
The versatile format()
method provided a powerful way to insert values and pad strings with zeros. We also explored the concise f-strings
for the same purpose.
String concatenation, a more hands-on approach, was demonstrated for dynamic zero-padding. By mastering these methods, Python developers can confidently handle string padding for different applications, ensuring strings meet specified length requirements.