How to Read Video in OpenCV
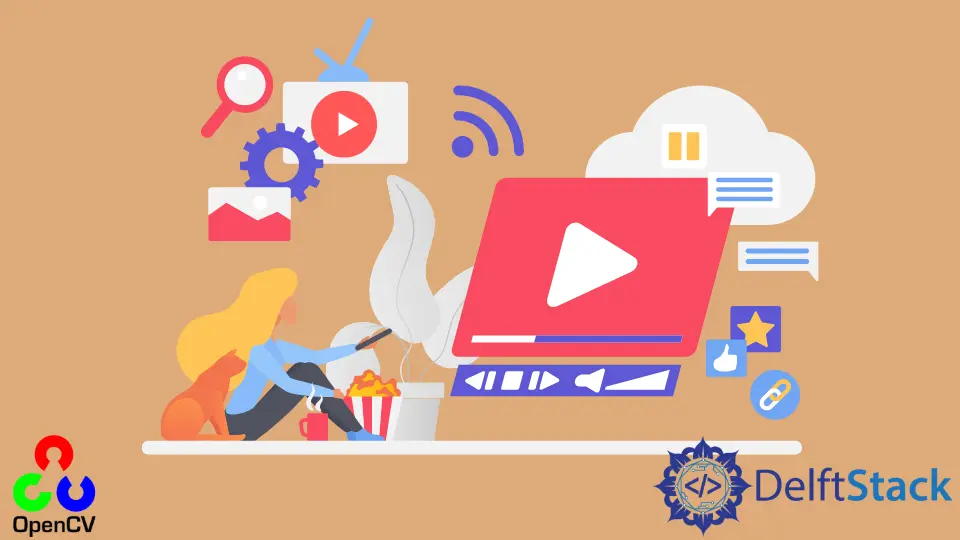
This tutorial will discuss reading a video using the VideoCapture()
function of OpenCV in Python.
Use the VideoCapture()
Function of OpenCV to Read a Video in Python
A video file contains multiple frames which we can read and show using OpenCV. We can use the VideoCapture()
function of OpenCV to read a video file.
We can use the cap.isOpened()
function to check if the capture is initialized or not. This function will return false if the capture is not initialized, and we can use the cap.open()
function to initialize the capture.
We can use the cap.read()
function to read the frames present in the video, and then we can process them and then show them using the imshow()
function of OpenCV. In this example, we will convert each frame to grayscale using the cvtColor()
function of OpenCV.
The cap.read()
function also returns a flag that will be true if the frame is received and ready to be processed, and we can use this flag to check the end of a video file and print something accordingly. This flag can also be used in a live stream video where we wait for the frame to arrive.
In this example, we will use the flag to check the end of the video, and if it is reached, we will print a message and break the loop. The waitKey()
function of OpenCV can add delay in the code in milliseconds, and we will use it to slow down the playing of the video.
After all the frames have been received and processed, we can use the cap.release()
function to release the capture. The destroyAllWindows()
function of OpenCV can destroy all open windows.
For example, let’s use a video, read its frames, and convert them to grayscale.
See the code below.
import numpy as np
import cv2
cap = cv2.VideoCapture("bon_fire_dog_2.mp4")
while cap.isOpened():
ret, frame = cap.read()
if not ret:
print("Can't receive frame (stream end?). Exiting ...")
break
gray = cv2.cvtColor(frame, cv2.COLOR_BGR2GRAY)
cv2.imshow("frame", gray)
cv2.waitKey(10)
if cv2.waitKey(1) == ord("q"):
break
cap.release()
cv2.destroyAllWindows()
Output:
We have also defined a key that will break the loop if pressed using the waitKey()
function. It is useful because we cannot terminate the video manually.
We can use the cap.set()
function to set many video capture properties. The first argument of the set()
function is the property we want to change, and the second is the property’s value.
For example, to set the width and height of the frames present in a video, we can use the cv2.CAP_PROP_FRAME_WIDTH
and cv2.CAP_PROP_FRAME_HEIGHT
properties. We can also set the frame rate, brightness, contrast, and other video properties.
Check this link for more details about the properties of the video capture. We can use the same properties inside the cap.get()
function to get their current values.
For example, we can use the cv2.CAP_PROP_FRAME_WIDTH
and cv2.CAP_PROP_FRAME_HEIGHT
properties to get the current width and height of the frames.