How to One Line FTP Server in Python
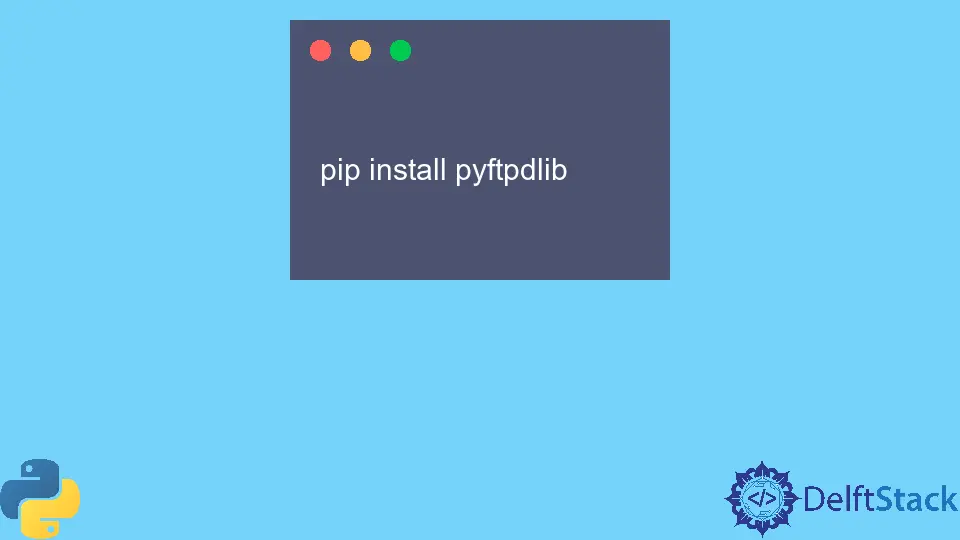
FTP is an abbreviation of File Transfer Protocol. It allows you to transfer files between client and server over a computer network.
Python library includes a pyftpdlib
module, allowing you to write efficient and scalable FTP servers with Python. This tutorial teaches you to create an FTP server using a one-liner in Python.
Use pyftpdlib
to Create One Line FTP Server in Python
First, you have to install the pyftpdlib
module.
pip install pyftpdlib
Output:
Successfully built pyftpdlib
Installing collected packages: pyftpdlib
Successfully installed pyftpdlib-1.5.6
Then you can execute the pyftpdlib
command in the terminal. For example, the following command starts an FTP server with the current directory.
python -m pyftpdlib
Output:
[I 2022-09-20 21:36:36] concurrency model: async
[I 2022-09-20 21:36:36] masquerade (NAT) address: None
[I 2022-09-20 21:36:36] passive ports: None
[I 2022-09-20 21:36:36] >>> starting FTP server on 0.0.0.0:2121, pid=3492 <<<
Next, verify whether the server is running.
curl ftp://127.0.0.1:2121
It will list all content of the current directory as follows.
Output:
-rw-rw-rw- 1 owner group 6817 May 20 06:55 -1.14-windows.xml
drwxrwxrwx 1 owner group 0 Nov 13 2021 .Icecream Screen Recorder
drwxrwxrwx 1 owner group 4096 Mar 28 02:51 .THypervBox
drwxrwxrwx 1 owner group 12288 Sep 18 14:46 .VirtualBox
drwxrwxrwx 1 owner group 4096 May 15 07:36 .android
The default mode is read-only. You can enable write access using the -w
option. The -d
flag allows you to use a different directory to share in the server.
The following example serves the directory C:\pc\test_folder
with write access for logged-in users.
python -m pyftpdlib -w -d C:\pc\test_folder
Output:
[I 2022-09-22 19:55:47] concurrency model: async
[I 2022-09-22 19:55:47] masquerade (NAT) address: None
[I 2022-09-22 19:55:47] passive ports: None
[I 2022-09-22 19:55:47] >>> starting FTP server on 0.0.0.0:2121, pid=25560 <<<
To check if it is running.
curl ftp://127.0.0.1:2121
Output:
drwxrwxrwx 1 owner group 0 Feb 23 2022 New folder
-rw-rw-rw- 1 owner group 0 Feb 23 2022 books.txt
-rw-rw-rw- 1 owner group 18 Jun 09 15:58 hello.txt
To disable anonymous login, you have to specify the username
and password
before starting the server.
The -u
option allows you to set the username
, and the -P
option helps set a password
to login into the FTP server.
python -m pyftpdlib -w -d C:\pc\test_folder -u username -P password
Output:
[I 2022-09-23 00:16:12] concurrency model: async
[I 2022-09-23 00:16:12] masquerade (NAT) address: None
[I 2022-09-23 00:16:12] passive ports: None
[I 2022-09-23 00:16:12] >>> starting FTP server on 0.0.0.0:2121, pid=22732 <<<
Execute this command to list the shared directory.
curl --user username:password ftp://127.0.0.1:2121/
Output:
drwxrwxrwx 1 owner group 0 Feb 23 2022 New folder
-rw-rw-rw- 1 owner group 0 Feb 23 2022 books.txt
-rw-rw-rw- 1 owner group 18 Jun 09 15:58 hello.txt
For more command options, run the help command.
python -m pyftpdlib --help