How to Create Local HTTP Server in Python
- Create an HTTP Server From Scratch in Python
- What Is An HTTP Server
- Build a Basic HTTP Server With One Command in Python
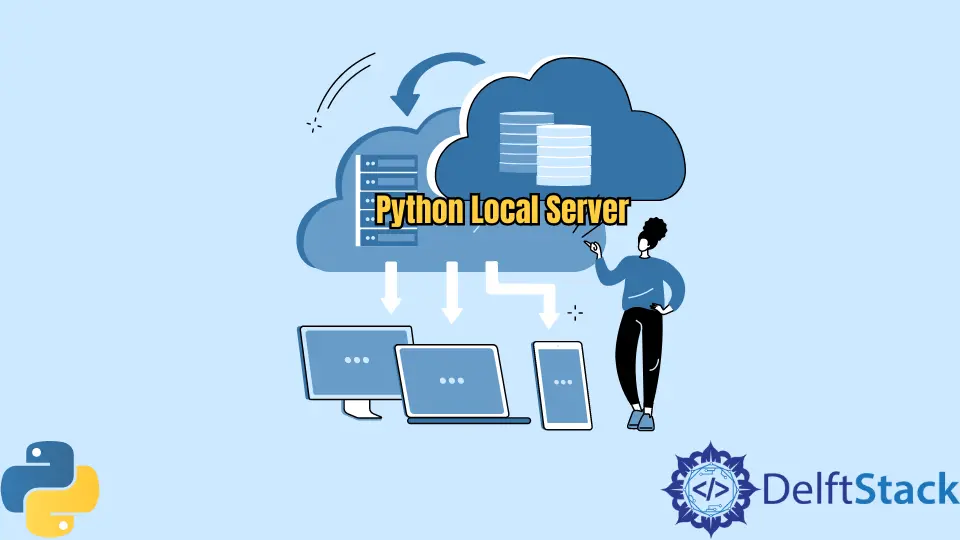
This tutorial will show us how to create an HTTP server in Python. And after going through this tutorial, it will be easy for us to set up an HTTP server with only a few lines of code.
Once we have created our HTTP server, we can start serving files from it. Let’s deep dive into Python HTTP server step-by-step guide for further assistance.
Create an HTTP Server From Scratch in Python
At some point in our career as software engineers, we will be required to work with web servers, regardless of our software engineer. Perhaps we may need to develop an API server for a backend service.
Maybe we would be merely setting up a web server for our website.
Python simplifies serving files from a directory using a primary HTTP server. This is by no means an innovative technique, but it is useful when we need it.
What Is An HTTP Server
An HTTP server is a computer program (or possibly a software component of another program) that acts as a server in a client-server architecture by implementing the server portion of the HTTP and/or HTTPS network protocols.
An HTTP server monitors incoming client requests (made by user agents such as browsers, web crawlers, and so on) and responds to each request by transmitting the requested web resource or returning an HTTP error message.
An HTTP server additionally contains bindings to manage protocol extensions to HTTP (such as WebDAV) or messages of other protocols encased in HTTP messages (such as SOAP) to support program-to-program interactions.
The complexity of an HTTP server implementation can range from a small and controllable component to a full-featured software implementation of HTTP and other protocols that can operate in the background and as one or more processes.
An HTTP server is found in every type of computer, including embedded systems and supercomputers, and is also necessary to operate web interfaces (web applications), among other things.
Let us clarify this point more precisely.
Consider opening our Chrome browser and typing www.gmail.com
into the address bar.
The Gmail
home page will be shown in the window of our web browser after running the link. However, what exactly happened behind the scenes?
Honestly, many things have happened, and we can devote some time to exploring the mysterious workings behind how this came to pass. However, we will discuss this topic with an example for clarity and conciseness.
When we input www.gmail.com
into our browser, our browser will generate a network message known as an HTTP request. This request will be sent to a machine at Gmail
operating a web server and will stay there until it is processed.
This web server will detect our request, process it, and then provide us the HTML for the gmail
homepage as a response.
In the end, our web browser displays this HTML to us on the screen of our system, which is what we see when we look at our computer.
This is how a request is sent and processed. Now that we know the behind-the-scene process, we can easily move to our main topic of creating an HTTP Server using Python.
Build a Basic HTTP Server With One Command in Python
Installation
We can install Python from here if we don’t already have it installed (probably using Windows).
As we have Python installed, we can go with the process of creating an HTTP Server. To begin, open the terminal and type the following command into the terminal.
Example code:
python -m http.server
When we run the command, we will get a message that notifies server started
and server stopped
, depending on our computers’ configuration.
And there we have it - our very own Python server! It’s a simple one, a web server on our machine’s default port of 8000
.
It solely performs this function. Changing the port is also possible by including the new port number at the end of the line, as seen here:
python -m http.server 8080
The above code changes the server port from default port 8000
to 8080
.
Now we need to go to either http://localhost:8000/
or http://127.0.0.1:8000/
to reach the server on our local network. From this point, we can see all the folders that make up our local storage and all the data.
We can also visit an HTML page; when we do so, our web browser will recreate the page for us automatically.
BaseHTTPRequestHandler
BaseHTTPRequestHandler
is a class used to manage the various requests sent to a server. It handles the Get
and Post
requests, not the actual HTTP request itself.
HTTPServer
This function, known as HTTPServer(server address, BASE HTTP REQUEST HANDLER(),)
, is used to save the server’s port number in addition to the name of the server.
The Approach in a Step-by-Step Manner
- We will construct a class responsible for managing the server’s requests.
- After we finish, we will develop a method in that class that will be utilized for
GET
requests. - Within the confines of that function, the HTML code that will be used to display it on the server will be drafted.
- At the very end, we will be utilizing the
HTTPServer()
method, which is responsible for the operation of our server.
Now, we will implement the steps described above using Python language.
# importing all the functions
# from http.server module
# * means all
from http.server import *
# creating a class for handling
# basic Get and Post Requests
class GFG(BaseHTTPRequestHandler):
# We will create a function
# for Get Request using the command below
def do_GET(self):
# Successful Response --> 200
self.send_response(200)
# Type of file that we are using for creating our
# web server.
self.send_header("content-type", "text/html")
self.end_headers()
# whatever we write in our function,
# we can see it at the web-server
self.wfile.write("<h1>AO-(AbidOrakzai)</h1>".encode())
# this object takes a report
# number and the server-Name
# for running the server
port = HTTPServer(("", 5555), GFG)
# We use this for running our
# server as long as we want, i.e., forever
port.serve_forever()
Output:
AO-(AbidOrakzai)
How Can We Start Our HTTP-Server
We can use the following terminal instructions to start our HTTP server.
Example code:
python our_file_name.py
And the last step is to access the server at port 5555
. We can use the browser http://localhost:5555/
or http://127.0.0.1:5555/
.
We hope that this article will be helpful for you in gaining a better knowledge of how an HTTP server can be created from scratch using Python.
My name is Abid Ullah, and I am a software engineer. I love writing articles on programming, and my favorite topics are Python, PHP, JavaScript, and Linux. I tend to provide solutions to people in programming problems through my articles. I believe that I can bring a lot to you with my skills, experience, and qualification in technical writing.
LinkedIn