How to Convert NumPy Array to List in Python
-
Use the
tolist()
Method to Convert a NumPy Array to a List -
Use the
for
Loop to Convert a NumPy Array to a List in Python
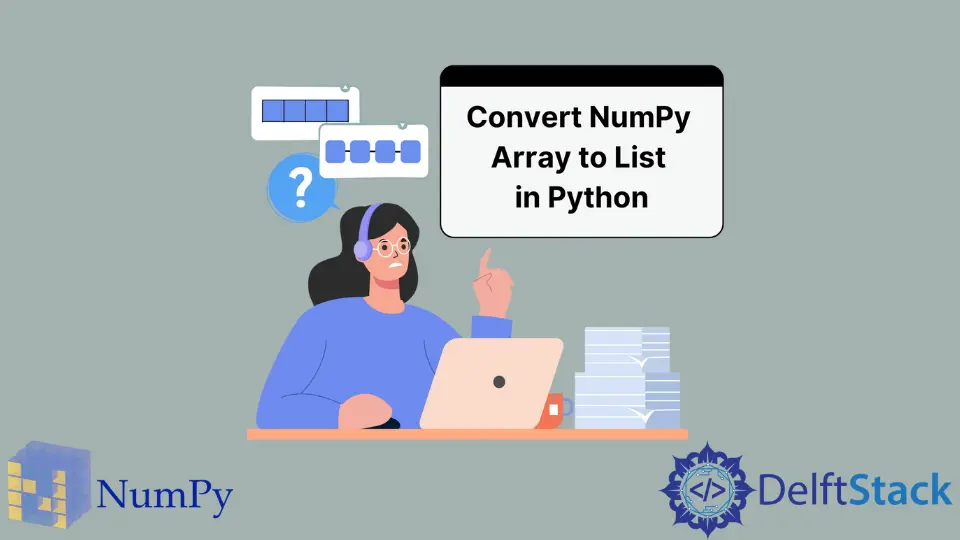
Lists and arrays are two of the most fundamental and frequently used collection objects in Python.
Both of them are mutable, used to store a collection of elements under a common name, and every element has a specific position that can be used to access it.
However, there are a few notable differences. Lists are already inbuilt in Python, whereas for arrays, we need to import arrays
or NumPy
module and need to declare arrays before using them. Arrays also store data more efficiently in memory and are highly used for mathematical operations.
Converting NumPy arrays to Python lists can be useful when working with standard Python operations. If you’re looking to extract specific parts of an array, check out [Getting a Subarray of an Array in Python]({{relref ‘/HowTo/Python/python subarray.en.md’}}).
In this tutorial, we will convert a numpy array to a list.
Use the tolist()
Method to Convert a NumPy Array to a List
The tolist()
Method of the NumPy array can convert a numpy array to a list.
For example,
import numpy as np
oned = np.array([[1, 2, 3]])
twod = np.array([[1, 2, 3], [4, 5, 6]])
print(oned.tolist())
print(twod.tolist())
Output:
[[1, 2, 3]]
[[1, 2, 3], [4, 5, 6]]
Note that this method treats the whole array as one element. That is why when we use it with a 2-D array it returns a list of lists.
To avoid this, we can use the tolist()
with the flatten()
or the ravel()
method, which can convert an N-D array to a linear 1-D array. Both these methods perform the same functionality. The difference is that the ravel()
method returns a reference view of the array and affects the original array, whereas the flatten()
method works on a copy of the array. Due to this, the ravel()
function is considered faster and occupies less memory.
The following code shows the use of these functions.
import numpy as np
oned = np.array([1, 2, 3])
twod = np.array([[1, 2, 3], [4, 5, 6]])
print(oned.flatten().tolist())
print(twod.flatten().tolist())
print(oned.ravel().tolist())
print(twod.ravel().tolist())
Output:
[1, 2, 3]
[1, 2, 3, 4, 5, 6]
[1, 2, 3]
[1, 2, 3, 4, 5, 6]
Use the for
Loop to Convert a NumPy Array to a List in Python
This is just a basic method for someone who is new to programming or wants to customize the final list. We iterate through an array and append each element individually to an empty list. The following code implements this.
import numpy as np
arr = np.array([1, 2, 3])
lst = []
for x in arr:
lst.append(x)
print(lst)
Output:
[1, 2, 3]
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn