Nested Dictionary in Python
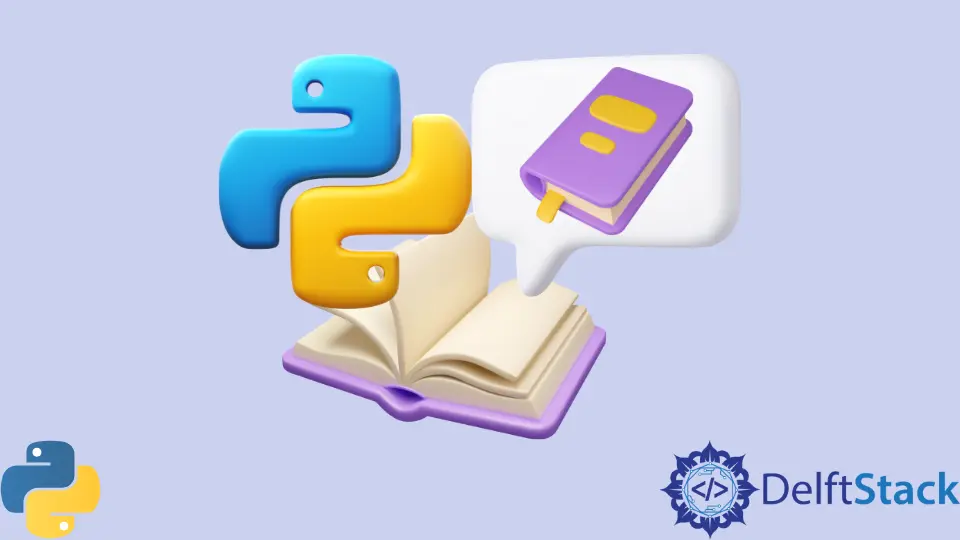
A Python nested dictionary is a dictionary within a dictionary, where the outer dictionary’s values are also dictionaries. The following code shows an elementary example.
d1 = {
0: {"Dept": "Mathematics", "Prof": "Dr Jack"},
1: {"Dept": "Physics", "Prof": "Dr Mark"},
}
print(d1)
Output:
{0: {'Dept': 'Mathematics', 'Prof': 'Dr Jack'}, 1: {'Dept': 'Physics', 'Prof': 'Dr Mark'}}
We can easily access the dictionary elements using the key of the nested dictionary, as demonstrated in the below example.
d1 = {
0: {"Dept": "Mathematics", "Prof": "Dr Jack"},
1: {"Dept": "Physics", "Prof": "Dr Mark"},
}
print(d1[0]["Dept"])
Output:
Mathematics
Here, 0
is the key of the outer dictionary, and 'Dept'
is the inner dictionary’s key.
We can also add elements as we do in a normal Python dictionary. Moreover, we can also add a whole dictionary as an element. For example:
d1 = {
0: {"Dept": "Mathematics", "Prof": "Dr Jack"},
1: {"Dept": "Physics", "Prof": "Dr Mark"},
}
d1[2] = {"Dept": "CS", "Prof": "Dr Jay"}
print(d1[2])
Output:
{"Dept": "CS", "Prof": "Dr Jay"}
Python has a defaultdict()
constructor in the collections
module to create dictionaries and return a default value when an unavailable key is accessed.
The defaultdict()
datatype is very useful when you want to return default values or initialize the dictionary as per your requirement. The following code shows how to create a simple dictionary with this method:
from collections import defaultdict
d1 = defaultdict(lambda: defaultdict(dict))
d1["key_outer_1"] = {"key_inner_1": "val1"}
d1["key_outer_2"] = {"key_inner_2": "val2"}
print(dict(d1))
Output:
{'key_outer_1': {'key_inner_1': 'val1'}, 'key_outer_2': {'key_inner_2': 'val2'}}
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn