How to Validate Numbers Using Luhn Algorithm in Python
- Validate Numbers Using the Luhn Algorithm in Python
- Use Functions to Validate Numbers Through the Luhn Algorithm
- Use Nested Loops to Validate Numbers Through the Luhn Algorithm
- Use Functional Programming to Validate Numbers Through the Luhn Algorithm
- Conclusion
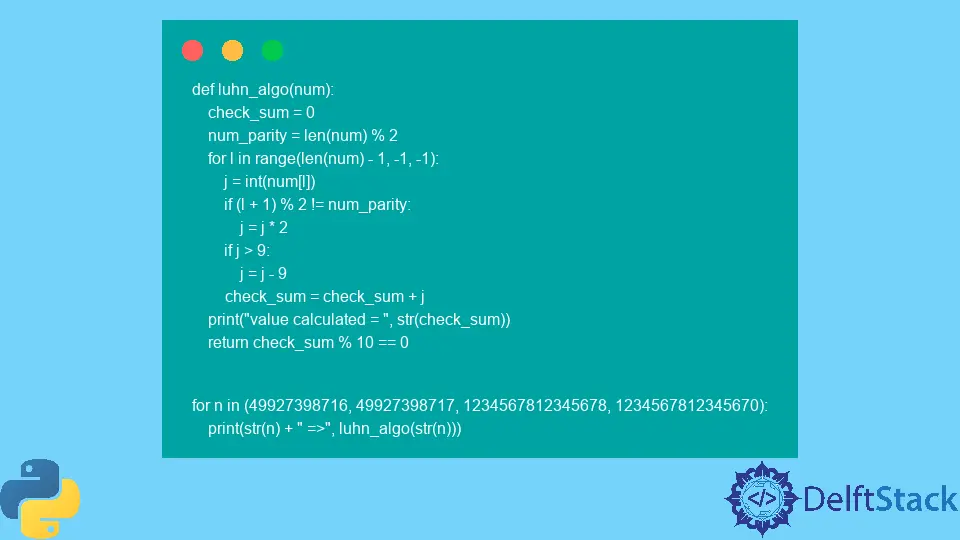
German computer scientist Hans Peter Luhn developed the Luhn algorithm formula in the 1960s. It is a checksum algorithm used by major international financial electronic funds transfer organizations like Visa and Master Card to speed up online payments and transactions.
This article explains writing the Luhn algorithm in Python and validates numbers according to the algorithm.
Validate Numbers Using the Luhn Algorithm in Python
The Luhn algorithm validator aids in examining and separating legitimate numbers from incorrect or misspelled inputs. To know more about it, check this link.
Let’s understand how to create a program that validates numbers using the Luhn algorithm in Python.
Use Functions to Validate Numbers Through the Luhn Algorithm
This program takes a number and validates it using the Luhn algorithm in Python. The program has three methods - separate_digits()
, luhn_algo()
, and check_if_valid
.
Step-by-step description:
-
The first line of code creates a method
luhn_algo
with a parameternum
. -
Inside the method, another nested method,
separate_digits()
, is created. This nested method separates the digits of the number passed to it and returns the separated numbers as a Python list.The method use a
for
loop to iterate the digits inside the list.def luhn_algo(num): def separate_digits(n): return [int(d) for d in str(n)]
-
A new variable,
digits
, uses theseparate_digits
method to convert the values insidenum
into a list of digits.digits = separate_digits(num)
-
Once the digits are stored in a Python list, the list needs to be reversed, and even and odd digits are needed to be separated. This is achieved by using the slice operator
::
.For example, in the syntax
odd_digits = digits[-1::-2]
, the-1::
reverses the list and takes the 1st index.The
::-2
picks up every second element starting from the 1st index. This creates a new list with just odd digits.Similarly, the list for even digits is created using the operator
-2::-2
.digits = separate_digits(num) odd_digits = digits[-1::-2] even_digits = digits[-2::-2]
-
The Luhn algorithm adds up the odd digits, while the even digits are added up after being multiplied by 2.
If the product of even digits is greater than 9, the sum of their digits is added. Lastly, all the odd and even digits are added up together.
A variable
checksum
is created to sum all the digits. The sum of odd digits is computed using the syntax:checksum += sum(odd_digits)
A
for
loop is created to iterate the list of even digits. This way, each digit gets multiplied by2
, and then the methodseparate_digits
separates the digits of the product, and then their sum is calculated.Lastly, it is added to the variable
checksum
.
```python
for d in even_digits:
checksum += sum(separate_digits(d * 2))
```
-
A number to get validated by the Luhn algorithm, its end product must be divisible by 10. The value of the result is returned at the end of the method.
return checksum % 10
-
To validate the result of the Luhn algorithm, a method
check_if_valid
is created with a parameternum
. The method checks if the result returned from the methodluhn_algo
is equal to zero by using the equity operators and returns the result.def check_if_valid(num): return luhn_algo(num) == 0
-
For printing the results, a number is passed to the method
check_if_valid
, and the results are printed.
Code:
def luhn_algo(num):
print("Number = ", num)
def separate_digits(n):
# Separates digits of num and stores them in a python list
return [int(d) for d in str(n)]
digits = separate_digits(num)
# Creates a new reversed list with just odd digits
odd_digits = digits[-1::-2]
# Creates another reversed list with even digits
even_digits = digits[-2::-2]
checksum = 0
checksum += sum(odd_digits) # Finds sum of odd digits
for d in even_digits:
checksum += sum(
separate_digits(d * 2)
) # Multiplies even digits with 2 and sums digits > 9
return checksum % 10
def check_if_valid(num):
return luhn_algo(num) == 0
result = check_if_valid(4532015112830366)
print("Correct:" + str(result))
result = check_if_valid(6011514433546201)
print("Correct:" + str(result))
result = check_if_valid(6771549495586802)
print("Correct:" + str(result))
Output:
Number = 4532015112830366
Correct:True
Number = 6011514433546201
Correct:True
Number = 6771549495586802
Correct:True
This way, a program can be easily created that validates numbers using the Luhn algorithm in Python.
Use Nested Loops to Validate Numbers Through the Luhn Algorithm
Another way to validate numbers through the Luhn algorithm in Python is by using nested loops. This program uses a single function to validate a number using the Luhn algorithm in Python.
Let’s understand how the code works.
-
The first line of code creates a method
luhn_algo
with a parameternum
. -
A variable
check_sum
is initialized with zero.def luhn_algo(num): check_sum = 0
-
A variable
num_parity
finds the length of the given number and checks its parity, whether it is even or odd.num_parity = len(num) % 2
-
A
for
loop is created that runs backward from its 0th position to its length. The value of thel
th index is copy initialized to a variablej
.for l in range(len(num) - 1, -1, -1): j = int(num[l])
-
The variable
num_parity
comes at this point. Ifnum_parity
is zero, this implies thatnum
is an even number, and vice versa for an odd number.As all the even digits needed to be multiplied by 2, the program checks the parity of its
l+1
th index. Suppose the parity is0
andl + 1 % 2
equals parity, it means that thel
th index is an odd digit.Similarly, if parity is
0
and it does not equall + 1 % 2
, it is an even digit. By using this logic, every even digit is multiplied by 2, and if the product is greater than 9, then 9 is divided from it.
The `check_sum` variable increments itself with the value of `j`. At the end of the loop iteration, the `check_sum` calculates the final sum of even and odd digits.
```python
if (l + 1) % 2 != num_parity:
j = j * 2
if j > 9:
j = j - 9
check_sum = check_sum + j
```
- As it is known that the final sum needs to be divisible by 10 to be validated using the Luhn algorithm in Python, the function returns
check_sum % 10 == 0
.
Code:
def luhn_algo(num):
check_sum = 0
num_parity = len(num) % 2
for l in range(len(num) - 1, -1, -1):
j = int(num[l])
if (l + 1) % 2 != num_parity:
j = j * 2
if j > 9:
j = j - 9
check_sum = check_sum + j
print("value calculated = ", str(check_sum))
return check_sum % 10 == 0
for n in (49927398716, 49927398717, 1234567812345678, 1234567812345670):
print(str(n) + " =>", luhn_algo(str(n)))
Output:
value calculated = 70
49927398716 => True
value calculated = 71
49927398717 => False
value calculated = 68
1234567812345678 => False
value calculated = 60
1234567812345670 => True
Use Functional Programming to Validate Numbers Through the Luhn Algorithm
The first two examples used a procedural method to validate numbers using the Luhn algorithm in Python. This example uses functional programming to validate numbers using the Luhn algorithm in Python.
Using the functional programming method saves the programmer time and effort. The below Python program validates a number in fewer lines of code.
-
A method
luhn_algo
is created with a parameternum
. -
In a new variable
rev
, the digits from the parameternum
are stored in a list. Afor
loop is used for this purpose that iterates for the number of digits there are innum
.The slicing operator
::-1
reverses the digits inside the list. -
The
return
statement computes the whole operation at once.The sum of odd digits is calculated using the syntax
(sum(rev[0::2])
. The even digits are run in afor
loop (for d in r[1::2]
).Each digit is multiplied by
2
, and the digits of the product are added using thedivmod()
function.The
divmod()
function takes in two parameters - numerator and denominator, and returns two values - quotient and remainder.The
divmod(d * 2, 10)
syntax takes ind*2
as the numerator and10
as the denominator. The resultant is added to get the sum of digits.Lastly, the function checks if the final sum is divisible by 10 and returns the result.
-
Using a
for
loop, four numbers are provided as input, and the result is printed.
Code:
def luhn_algo(num):
rev = [int(ch) for ch in str(num)][::-1]
return (sum(rev[0::2]) + sum(sum(divmod(d * 2, 10)) for d in rev[1::2])) % 10 == 0
for num2 in (49927398716, 49927398717, 1234567812345678, 1234567812345670):
print(num2, luhn_algo(num2))
Output:
49927398716 True
49927398717 False
1234567812345678 False
1234567812345670 True
Conclusion
This article provides three programs for the reader to understand how to validate numbers using the Luhn algorithm in Python.
It is suggested that the reader goes through the article, tries writing the code by themselves, and comes back for hints. This way, the reader can create programs that validate numbers using the Luhn algorithm.