Python Crc32
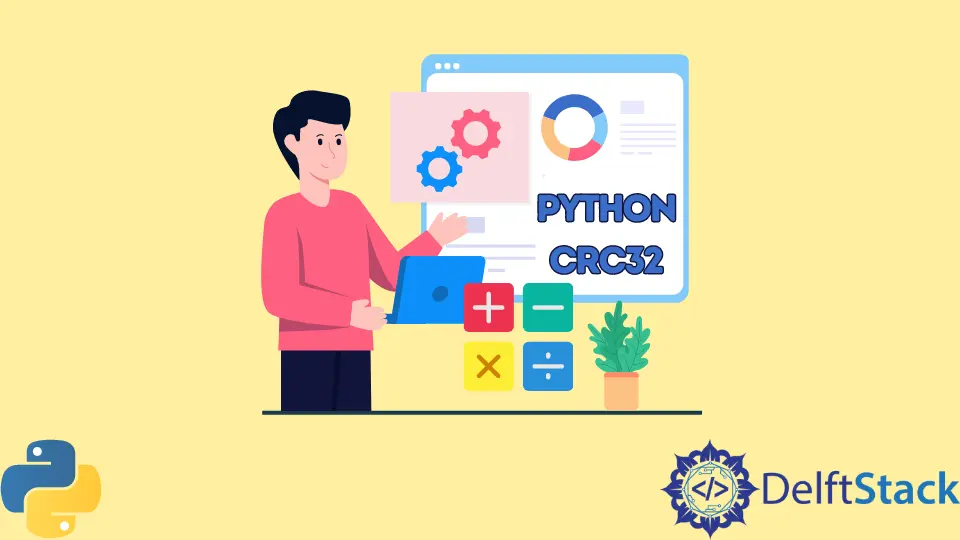
This tutorial will discuss computing the crc32 of data using the binascii
or zlib
library in Python.
Python CRC32
The CRC32 is the checksum of data, also known as cyclic redundancy check, which is used to check errors present in a digital transmission of data. In digital transmission, the data at the output might change with respect to data at the input because of noise or interference, and to check if the data at the output of the transmission is changed, we can use the CRC32 to find the difference in the checksum of data at the input and output.
If the difference between the input and output checksum of data is zero, there is no error in the transmission, and the data at the output is the same as the data at the transmission’s input. The checksum of data represents the number of bits present and is attached to the file before it is sent.
We already know the checksum of input data, we only have to find the checksum of the output data, and their difference will tell us about the error present in the data. We can use the crc32()
function of binascii
or zlib()
library to find the CRC32 or checksum of data.
We have to pass the data in bytes inside the crc32()
function and the function will return the 32-bit unsigned integer of that data. If the input data is not in byte data type, we must convert it to byte before passing it inside the crc32()
function.
If the input value passed inside the crc32()
function is zero, the output will also be zero. For example, let’s use the binascii
and zlib
libraries to find the CRC32 checksum of a string.
See the code below.
import binascii
import zlib
crc1 = binascii.crc32(b"abcd")
crc2 = zlib.crc32(b"abcd")
print(crc1)
print(crc2)
Output:
3984772369
3984772369
In the above code, we used the character b
inside the crc32()
function to convert the string into bytes. The algorithm used to find the checksum is not a cryptographic algorithm and is not quite accurate, so it cannot be used for hashing purposes.
The above code is tested in Python 3 and will return 32-bit unsigned integer, but if we run the same code in Python 2, the result will be a 32-bit signed integer, and both the result will not be the same. To convert the result of Python 2 to unsigned, we have to take the bitwise AND
of the output with 0xffffffff
using the &
character.
In case you’re interested in how to convert bytes into integers in Python, check out this guide on How to Convert Bytes to Int in Python 2.7 and 3.x.
The binascii
library can convert data from binary to ASCII, like binary to hexadecimal. It can convert the above byte value to hexadecimal using its b2a_hex()
function.
The zlib
library can compress and decompress data.