How to Sort Dictionary by Key in Python
-
Python Sort Dictionary by Key With the
dict.keys()
Method -
Python Sort Dictionary by Key With the
dict.items()
Method -
Python Sort Dictionary by Key With the
OrderedDict()
Method - Sort Dictionary in Reverse Order in Python
-
Python Sort Dictionary With Custom
key
Function Method
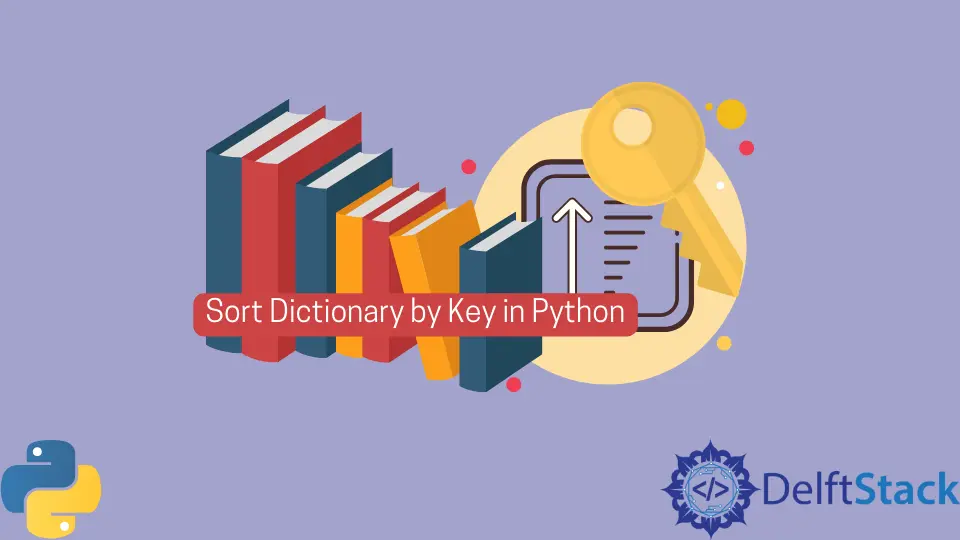
Python dictionary is the same as the hash table that keeps the entries by evaluating the hashes of keys, and the sequence of entries are impossible to anticipate.
This article will introduce how to sort dictionary by key in Python.
Python Sort Dictionary by Key With the dict.keys()
Method
Let’s use the below dictionary as an example.
dict = {"hello": 56, "at": 23, "test": 43, "this": 43}
The output of the dict.keys()
is
dict_keys(["hello", "at", "test", "this"])
We could make a new, ordered dictionary from this unordered list of keys.
sorted(dict.keys())
Output:
['at', 'hello', 'test', 'this']
We repeatedly apply the sorting on this ordered list by select each entry from the dictionary.
for key in sorted(dict.keys()):
print(key, " :: ", dict[key])
Output:
at :: 23
hello :: 56
test :: 43
this :: 43
Python Sort Dictionary by Key With the dict.items()
Method
We can also sort a dictionary by key in Python with the dict.items()
method.
It will generate a list that holds key-value pairs?
dict.items()
Output:
dict_items([("hello", 56), ("at", 23), ("test", 43), ("this", 43)])
We could make an arranged list by the following function. It will sort the entries of the dictionary according to the key values.
sorted(dict.keys())
Output:
['at', 'hello', 'test', 'this']
Now to produce the sort key-value pair from the dictionary, we use the following code.
for elem in sorted(dict.items()):
print(elem[0], " ::", elem[1])
Output:
at :: 23
hello :: 56
test :: 43
this :: 43
In terms of complexity, it is more powerful than the previous approach. Since we don’t need to check for key-value after sorting the iterable list, as in dict.key()
.
Python Sort Dictionary by Key With the OrderedDict()
Method
Alternatively, we can sort the dictionary items by key-value using the collections
module.
import collections
d = {2: 13, 1: 9, 4: 25, 3: 0}
result = collections.OrderedDict(sorted(d.items()))
print(result)
Output:
OrderedDict([(1, 9), (2, 13), (3, 0), (4, 25)])
Sort Dictionary in Reverse Order in Python
Previously, we sorted the dictionary items in ascending order. Now we discuss some ways to sort the dictionary items in descending order.
The syntax is:
sorted(iterable_sequence, reverse=True)
The following code sorted and reverse the dictionary items.
dict = {"hello": 56, "at": 23, "test": 43, "this": 43}
for elem in sorted(dict.items(), reverse=True):
print(elem[0], " ::", elem[1])
The parameter reverse=true
makes sure that the sorted dictionary is reversed.
Output:
this :: 43
test :: 43
hello :: 56
at :: 23
Python Sort Dictionary With Custom key
Function Method
This method will sort the dictionary items by using the length of the key
string.
sorted(iterable_sequence, key=Function)
The lambda
function that returns the string size is given to the key
parameter.
listofTuples = sorted(dict.items(), key=lambda x: len(x[0]))
for elem in listofTuples:
print(elem[0], " ::", elem[1])
Output:
at :: 23
test :: 43
this :: 43
hello :: 56