How to Remove the Last Character From String in Python
- Remove the Last Character From String in Python With the Slicing Method
- Python String Last 3 Characters Removal With Negative Indexing Method
- Python String Last 3 Characters Removal With the Slicing Method and Positive Indexing
-
Python String Last 3 Characters Removal With the
for
Loop Method - Python String Last 3 Characters Removal With the Regex Method
- Python String Last Character Removal With the Regex Method
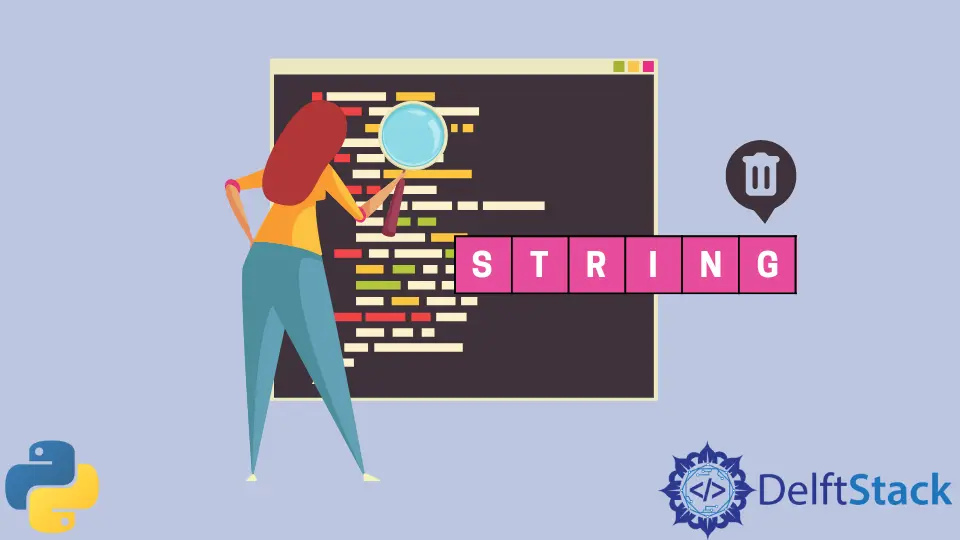
A Python String is a combination of characters enclosed in double quotes or single quotes. Python provides multiple functions to manipulate the string.
This article will introduce different methods to remove the last character and specific characters at the end of the string.
Remove the Last Character From String in Python With the Slicing Method
Let us take the below code as an example:
my_str = "python string"
final_str = my_str[:-1]
print(final_str)
Python string index starts from 0. Python also has negative indexing and uses -1
to refer to the last element. The slicing operator accesses the last element of the string and removes it.
The output of the slicing method is:
python strin
Python String Last 3 Characters Removal With Negative Indexing Method
We could use negative indexing in Python. The last character starts from index -1 and reaches the first character in the order -2, -3, -4, and so on.
The code is:
my_str = "python string"
final_str = my_str[:-3]
print(final_str)
Output:
python str
Python String Last 3 Characters Removal With the Slicing Method and Positive Indexing
The len()
function determines the length of the string. This method will choose the elements from the start position 0 to the last position N and subtract the last 3 characters i.e.(N-3).
The default of the first argument of the slicing method is 0.
The code is:
my_str = "python string"
size = len(my_str)
final_str = my_str[: size - 3]
print(final_str)
Output:
python str
Python String Last 3 Characters Removal With the for
Loop Method
We apply a loop on all the string characters, starting from the first index 0 to the last index (N-1), and remove the 3 characters at the end of the string.
The code is:
my_str = "python string"
n = 3
final_str = ""
for i in range(len(my_str) - n):
final_str = final_str + my_str[i]
print(final_str)
Output:
python str
Python String Last 3 Characters Removal With the Regex Method
This method is used in Python to compare two groups. We use a built-in library called re
that checks a specific pattern in a string.
The code is:
import re
def rmve_2nd_grp(b):
return b.group(1)
my_str = "Python String"
result = re.sub("(.*)(.{3}$)", rmve_2nd_grp, my_str)
print(result)
In the code, the sub()
method compares the defined pattern and passed that similar object to the method rmve_2nd_grp()
. The matched object has two groups, but rmve_2nd_grp()
compares characters from group1 and gives back the string.
Output:
Python Str
Python String Last Character Removal With the Regex Method
If you need to remove the last character, use the below code:
import re
def rmve_2nd_grp(b):
return b.group(1)
my_str = "Python String"
result = re.sub("(.*)(.{1}$)", rmve_2nd_grp, my_str)
print(result)
Output:
Python Strin