How to Convert String to Lowercase in Python 2 and 3
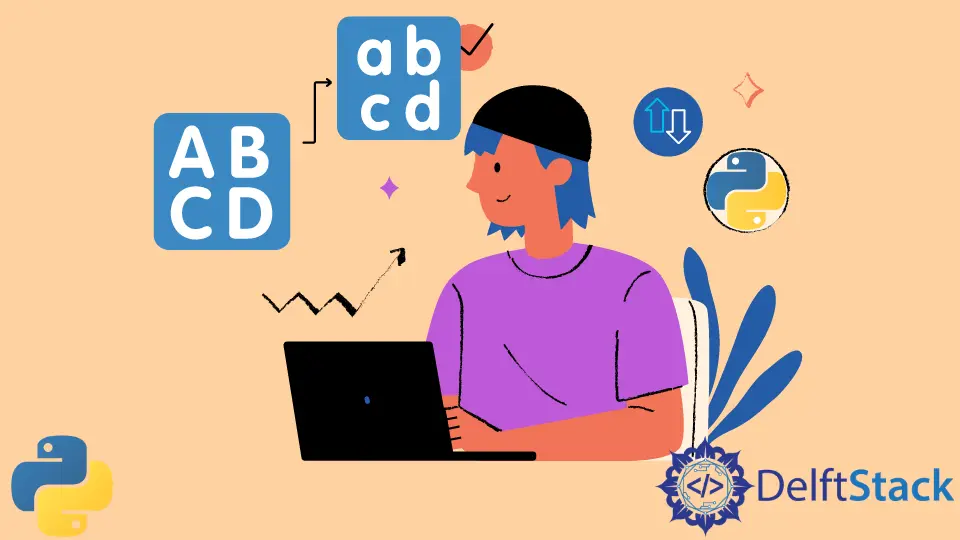
Convert String to Lowercase in Python 3
str
type since Python 3.0 contains by default Unicode
characters, meaning any string like "unicode example"
, 'unicode example 2'
is stored as Unicode
.
Therefore, you could use str.lower()
to convert any string to lowercase in Python 3.
exampleString = "CaseString"
exampleString.lower()
# Out: 'casestring'
exampleString = "СтрокаСлучая"
exampleString.lower()
# Out: 'строкаслучая'
str.casefold()
Caseless Conversion
str.lower()
converts the string to lowercase, but it doesn’t convert the string’s case distinctions.
For example, ß
in German is equal to double s
- ss
, and ß
itself is already lowercase, therefore, str.lower()
will not convert it.
Но str.casefold()
превратит ß
в ss
.
>>> 'Straße'.lower()
'straße'
>>> 'Straße'.casefold()
'strasse'
Convert String to Lowercase in Python 2.7
str
type in Python 2.7 is not stored as Unicode
, and Unicode
strings are instances of the unicode
type. We must distinguish whether the string is an ASCII
string or Unicode
string when converting the string to lowercase.
ASCII
Type
It is the same as the method used in Python 3. str.lower()
converts the string to lowercase.
exampleString = "CaseStringExample"
exampleString.lower()
# Out: 'casestringexample'
unicode
Type
If the string’s characters are Unicode
type, and the string is not explicitly represented in the Unicode
type, then the str.lower()
method doesn’t convert the string to lower case at all.
# python 2.x
exampleString = "СтрокаСлучая"
print exampleString.lower()
# Out: СтрокаСлучая
exampleString.lower() == exampleString
# Out: True
Python objects to non-ASCII bytes in a string with no-encoding given because the intended coding is implicit.
Using Unicode
literals but not str
# python 2.x
exampleUnicodeString = u"СтрокаСлучая"
exampleUnicode
# u'\u0421\u0442\u0440\u043e\u043a\u0430\u0421\u043b\u0443\u0447\u0430\u044f'
exampleUnicodeString.lower()
# u'\u0441\u0442\u0440\u043e\u043a\u0430\u0441\u043b\u0443\u0447\u0430\u044f'
print exampleUnicodeString.lower()
# Out: строкаслучая
You could see here that the string’s first character is converted from \u0421
to \u0441
.
Convert a str
to unicode
If the given string is in the form of str
, we must first convert it to Unicode
before lowercase conversion.
# python 2.x
exampleString = "СтрокаСлучая"
print exampleString.decode("utf-8").lower()
# Out: строкаслучая
Conclusion
lower()
method is the method to convert string to lower case both in Python 2 and 3, but with a noticeable difference.
The string in Python 3 is the unicode
string by default, but the string in Python 2 is not. If the string is not explicitly represented in the unicode
type, for example, not putting u
before the string, the unicode string will not be converted to lowercase at all.
str.casefold
converts the case distinctions to their caseless matches, but it is only available in Python 3. You could install py2casefold in Python 2.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook