How to Get Length of a Python Array
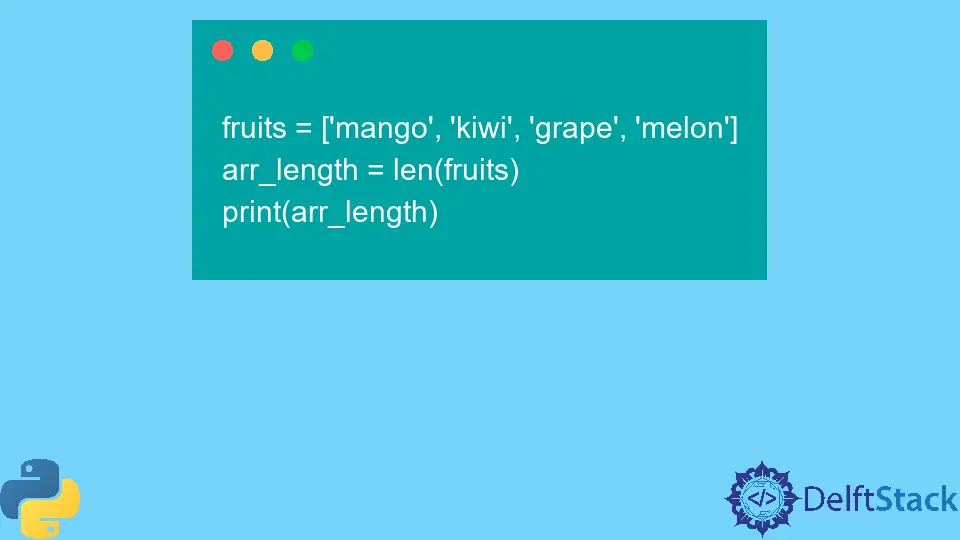
Finding the length of an array in Python is a fundamental skill for any developer. Whether you’re working with lists, tuples, or other iterable objects, knowing how to determine their size is essential for effective programming.
In this article, we will explore two primary methods to find the length of a Python array: using the built-in len()
function and the __len__()
method. Each method has its use cases and advantages, making it important to understand both. By the end of this article, you will be equipped with the knowledge to efficiently find the length of various Python objects in your projects.
Using the len()
Function
The len()
function is the most straightforward way to get the length of an array in Python. It is a built-in function that takes an iterable as an argument and returns the total number of items in that iterable. This method is not only simple but also highly efficient.
Here’s how you can use the len()
function:
my_list = [1, 2, 3, 4, 5]
length_of_list = len(my_list)
print(length_of_list)
Output:
5
In this example, we create a list named my_list
containing five integers. By passing this list to the len()
function, we get the length of the list, which is stored in the variable length_of_list
. Finally, we print the value, which shows that there are five elements in the list.
The len()
function works with various data types, including strings, tuples, and dictionaries, making it a versatile tool for developers. It is worth noting that the performance of len()
is O(1), meaning it executes in constant time regardless of the size of the iterable. This efficiency makes it an ideal choice for performance-critical applications.
Using the __len__()
Method
Another way to determine the length of an array in Python is by using the __len__()
method. This method is defined within the class of the object you are working with. While it is less commonly used than the len()
function, it can be particularly useful when you are working with custom classes or need to override the default behavior of an object.
Here’s an example of how to use the __len__()
method:
class CustomList:
def __init__(self, elements):
self.elements = elements
def __len__(self):
return len(self.elements)
my_custom_list = CustomList([1, 2, 3, 4, 5])
length_of_custom_list = my_custom_list.__len__()
print(length_of_custom_list)
Output:
5
In this example, we define a class called CustomList
, which has an __init__
method to initialize its elements. The __len__()
method is overridden to return the length of the elements
attribute. When we create an instance of CustomList
and call the __len__()
method, it returns the length of the contained list. This approach provides flexibility for customizing how length is calculated for user-defined objects.
While using __len__()
directly is less common, it can be particularly useful for creating classes that behave like built-in types. This method can enhance the readability of your code by allowing you to use the len()
function seamlessly with your custom objects.
Conclusion
In summary, knowing how to get the length of a Python array is a fundamental skill for any programmer. Whether you choose to use the built-in len()
function or the __len__()
method, both approaches offer their unique advantages. The len()
function is straightforward and efficient, while the __len__()
method provides flexibility for custom classes. By mastering these techniques, you will be better prepared to handle various data structures in your Python projects.
FAQ
-
What is the difference between len() and len()?
len() is a built-in function that works with all iterable objects, while len() is a method defined in a class to customize how length is calculated for that specific object. -
Can I use len() on a string?
Yes, len() can be used on strings to get the number of characters in the string. -
Is there a performance difference between len() and len()?
Generally, there is no significant performance difference since len() internally calls the len() method of the object. -
Can I override len() in my custom class?
Yes, you can override len() in your custom class to define how the length of your object is calculated. -
What happens if I call len() on an empty list?
len() will return 0 if called on an empty list, indicating that there are no elements present.