How to Get IP Address in Python
-
Use the
os
Module to Get the Local IP Address in Python -
Use the
socket.gethostname()
andsocket.gethostbyname()
Functions to Get the Local IP Address in Python -
Use the
socket.getsockname()
Function to Get the Local IP Address in Python -
Use the
netifaces
Module to Get the Local IP Address in Python - Conclusion
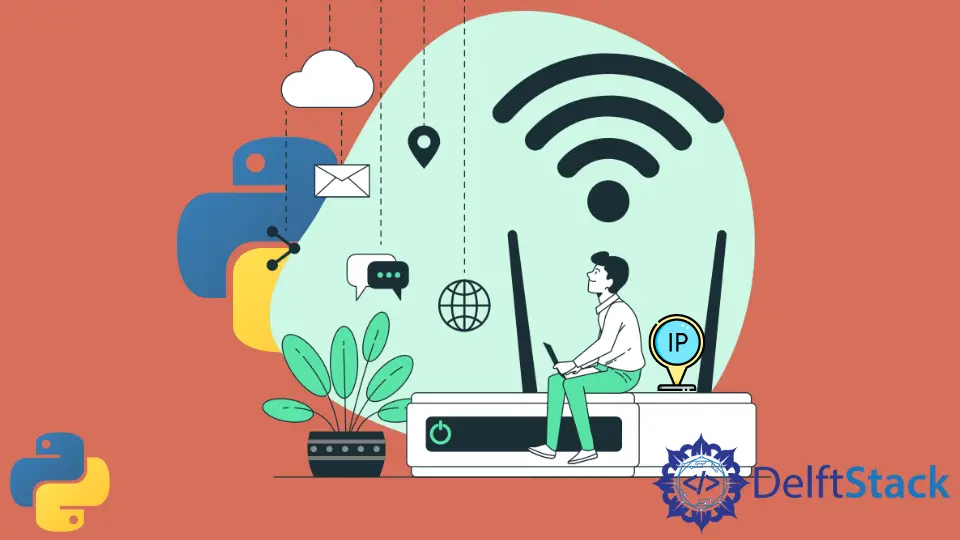
In today’s interconnected world, where networking forms the backbone of communication, understanding IP addresses is vital for any aspiring programmer. Whether we are building a web application, troubleshooting network issues, or implementing security measures, the ability to retrieve IP addresses programmatically using Python is an invaluable skill.
An IP address, short for Internet Protocol address, is a unique numerical label assigned to devices connected to a computer network, enabling communication and data exchange. IP addresses are a sequence of digits starting from 0.0.0.0 until 255.255.255.255, with each number in the address ranges of 0 to 255. It can uniquely identify a device over a network.
Imagine we are developing a security application that analyzes incoming network traffic. We can identify potential threats, block suspicious activity, and enhance system security by obtaining IP addresses.
Similarly, retrieving user IP addresses in a web application allows for personalized content and improved user experience by determining geographical location.
This tutorial explores methods and libraries for effortlessly retrieving IP addresses in Python.
Use the os
Module to Get the Local IP Address in Python
In Python, the os
module provides a platform-independent way to interact with the underlying operating system. While it does not have a direct function for retrieving the local IP address, we can use it with other techniques.
By executing system commands using the os.system()
function, we can invoke commands like ipconfig
(Windows) or ifconfig
(Unix-like systems) to obtain network interface information, including the local IP address. This approach allows us to leverage the existing functionality of the operating system and retrieve the local IP address within our Python scripts.
The following code snippet shows how we can get the IP address in Python using the os
module.
import os
print(os.system("ipconfig"))
Output:
The above code uses the os.system()
function to execute the ipconfig
command (on Windows) and displays the output in the console.
Use the socket.gethostname()
and socket.gethostbyname()
Functions to Get the Local IP Address in Python
We can use the socket
module in Python to create connections and send messages across the network. The socket.gethostname()
function in Python provides a simple yet effective way to retrieve the local IP address of a device.
This function returns the hostname of the current system under which the Python interpreter executes. We can obtain the corresponding IP address associated with the device by utilizing this hostname.
Syntax:
import socket
local_ip = socket.gethostname()
The socket.gethostname()
function requires no parameters. It directly retrieves the device’s hostname on which the Python script runs.
We can utilize the hostname returned by the socket.gethostname()
function to get the IP address from the socket.gethostbyname()
function. The socket.gethostbyname()
function in Python is used to retrieve the IP address corresponding to a given hostname.
It takes a hostname
as a parameter and returns the IP address associated with that hostname. This function performs a DNS lookup to resolve the hostname to its corresponding IP address.
Syntax:
import socket
ip_address = socket.gethostbyname(hostname)
Parameters:
hostname
: A string representing the hostname for which we want to obtain the IP address. It can be a domain name (e.g.,www.example.com
) or a local hostname (e.g.,localhost
).
The advantage of socket.gethostbyname()
over os.system()
is that it’s a direct Python function that retrieves IP addresses programmatically, providing a more efficient and reliable method.
On the other hand, os.system()
relies on executing system commands, making it less flexible and potentially platform-dependent.
The following code snippet shows how we can get the IP address in Python using the socket.gethostbyname()
function.
import socket
print(socket.gethostbyname(socket.gethostname()))
Output:
The above code retrieves the local IP address of the device using Python’s socket
module. It first calls the socket.gethostname()
function, which returns the hostname of the current system.
Then, the socket.gethostbyname()
function is called, passing the obtained hostname as the parameter. This function performs a DNS lookup and returns the IP address associated with the hostname.
Finally, the obtained IP address is printed to the console using the print()
function.
Use the socket.getsockname()
Function to Get the Local IP Address in Python
In Python, the socket.getsockname()
function provides a powerful method to retrieve the local IP address of a device. Unlike the previously discussed socket.gethostbyname()
method, which retrieves the IP address associated with a hostname, getsockname()
focuses on obtaining the IP address of a specific socket.
Syntax:
import socket
sock = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
sock.connect((host, port))
local_ip = sock.getsockname()[0]
Input Parameters:
-
host
: A string representing the hostname or IP address of the target server. -
port
: An integer indicating the port number of the target server.
The socket.getsockname()
function is called on a connected socket sock
after establishing a connection using the socket.connect()
method. It returns a tuple containing the local IP address and port number associated with the socket.
To access only the IP address, [0]
is used to retrieve the first element of the tuple.
Compared to socket.gethostbyname()
, which retrieves the IP address based on a hostname, getsockname()
allows for more direct and precise retrieval of the local IP address associated with a socket. This is particularly useful in situations involving multiple network interfaces or IP addresses, such as in multi-homed systems or when dealing with specific network configurations.
See the code below.
import socket
s = socket.socket(socket.AF_INET, socket.SOCK_DGRAM)
s.connect(("8.8.8.8", 80))
print(s.getsockname()[0])
Output:
The above code retrieves the local IP address of the device by establishing a temporary connection to the remote server with IP address 8.8.8.8 on port 80 using a UDP socket. It creates a socket object s
with the appropriate address family socket.AF_INET
and socket type socket.SOCK_DGRAM
.
Then, it uses the connect()
method to connect to the specified remote server. Finally, it retrieves and prints the local IP address associated with the socket using getsockname()[0]
.
The above method works on all interfaces. It also works with all public, private, and external IPs. This method is efficient on Linux, Windows, and OSX.
Use the netifaces
Module to Get the Local IP Address in Python
The netifaces
module provides information about the network interfaces and their status.
In Python, the netifaces
module provides a comprehensive solution for retrieving the IP addresses associated with each network interface on a device. This module offers functions like interfaces()
, ifaddresses()
, and constants like AF_INET
to facilitate the process.
The interfaces()
function returns a list of the available network interfaces on the device. It allows us to iterate over each interface and gather their IP addresses.
The ifaddresses()
function takes an interface name as a parameter and returns a dictionary containing the address information associated with that interface. By specifying the AF_INET
constant, we can retrieve the IPv4 addresses.
This function allows us to extract the desired IP addresses from the interface information.
As shown below, we can use it to get the local machine’s IP address.
from netifaces import interfaces, ifaddresses, AF_INET
for ifaceName in interfaces():
addresses = [
i["addr"]
for i in ifaddresses(ifaceName).setdefault(AF_INET, [{"addr": "No IP addr"}])
]
print(" ".join(addresses))
Output:
The above code utilizes the netifaces
library in Python to retrieve the IP addresses associated with each network interface on the device. It begins by importing the necessary modules: interfaces
, ifaddresses
, and AF_INET
from the netifaces
library.
The code then uses a for
loop to iterate over each network interface present on the device. For each interface, it calls ifaddresses(ifaceName)
to retrieve the address information associated with that interface.
The AF_INET
parameter specifies that we are interested in IPv4 addresses. Using list comprehension and the setdefault()
method, it extracts the IPv4 addresses from the address information.
If no IP address is available for the interface, it defaults to 'No IP addr'
. Finally, the retrieved IP addresses are printed to the console using print(' '.join(addresses))
.
Conclusion
We have explored four methods in Python for retrieving local IP addresses, each with advantages and disadvantages.
Using the os.system()
method allows leveraging system commands like ipconfig
or ifconfig
to obtain network interface information, but it relies on executing system commands and may be platform-dependent.
The socket.gethostbyname()
method provides a simple way to retrieve the IP address associated with a hostname but is limited to hostname-based resolution.
The socket.getsockname()
method allows direct retrieval of the local IP address associated with a socket, but it requires a socket connection and is primarily suited for socket-related tasks.
The netifaces
module offers a comprehensive solution for accessing IP addresses of all network interfaces but requires an additional library dependency and may have a steeper learning curve.