How to Ping A Server using Python
-
Ping Server in Python Using the
subprocess.call()
Method -
Ping Server in Python Using the
os.system()
Method -
Ping Server in Python Using the
ping3.ping()
Function - Conclusion
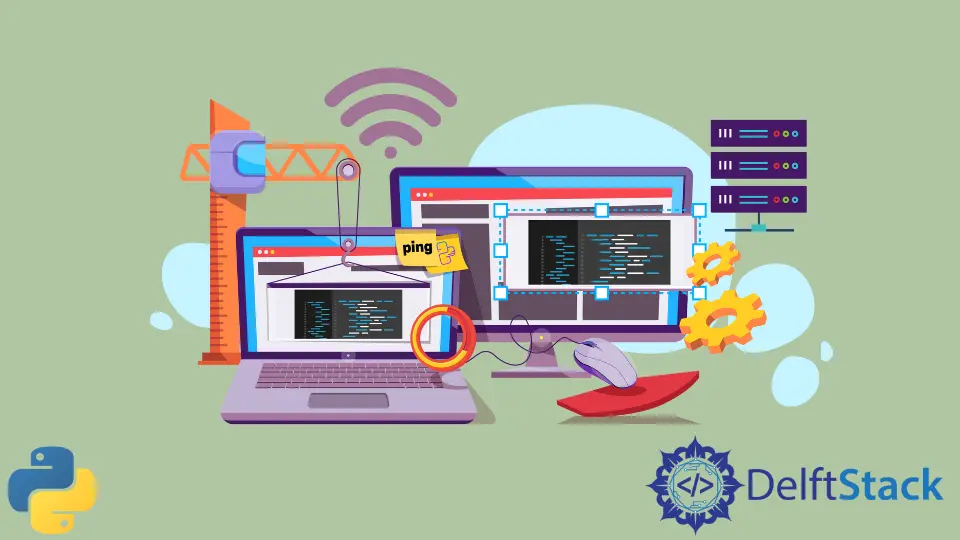
This tutorial will explain various methods to ping a server using Internet Control Message Protocol (ICMP) in Python. Pinging a server is a fundamental operation in network diagnostics, used to test the reachability of a host on an IP network and measure the round-trip time for messages sent from the originating host to a destination computer.
Ping Server in Python Using the subprocess.call()
Method
The subprocess.call(command)
method takes command
as input and executes it. It returns 0
if the command executes successfully.
The command to ping a server will be ping -c 1 host_address
for Unix and ping -n 1 host_address
for Windows, where 1
is the number of packets and host_address
is the server address we want to ping.
We can use the platform.system()
method first to check the OS of the machine and then run the command accordingly. The below example code demonstrates how to use the subprocess.call()
method to execute the command to ping a server in Python.
import platform
import subprocess
def myping(host):
# Choose parameter based on OS
parameter = "-n" if platform.system().lower() == "windows" else "-c"
# Constructing the ping command
command = ["ping", parameter, "1", host]
response = subprocess.call(command)
# Interpreting the response
return response == 0
# Testing the function
print(myping("www.google.com"))
In our myping
function, we first determine the operating system using platform.system()
and accordingly set the ping command parameter (-n
for Windows, -c
for others). This ensures cross-platform compatibility.
We then construct the ping command as a list, where the first element is the command itself (ping
), followed by the parameter specifying the number of echo requests (1
), and finally, the target host (host
).
The subprocess.call()
method executes this command and returns an exit status: 0
for a successful ping (indicating the host is reachable) and a non-zero value for failure.
We return True
if the response is 0
; otherwise, False
. This return value succinctly informs us whether the specified host is reachable or not.
Output:
Ping Server in Python Using the os.system()
Method
The os.system(command)
method takes the command
(a string) as input and executes it in a subshell. The method returns 0
if the command executes without any error.
We can use the os.system()
method in the following way to execute the ping server command:
import os
def myping(host):
# Executing the ping command
response = os.system("ping -c 1 " + host)
# Checking the response
return response == 0
# Testing the function with www.google.com
print(myping("www.google.com"))
In our myping
function, we utilize os.system()
to execute the ping command, constructing it by appending the host to "ping -c 1 "
. This command sends a single ICMP packet to the specified host.
The function’s return value is the exit status of the command executed: 0
indicates that the ping was successful, signifying the host is reachable. Any non-zero value, on the other hand, suggests a failure, either due to the host being unreachable or a timeout.
By returning response == 0
, we convert this exit status into a boolean value, True
for a successful ping and False
otherwise, offering a clear and concise indication of the host’s reachability.
Output:
Ping Server in Python Using the ping3.ping()
Function
The ping(addr)
function of the ping3
module takes the server address as input and returns the ping time as output if the server is available and returns False
if it is not available.
We can install the ping3
module with the root
privileges.
pip install ping3
We can pass the server address to the ping()
method to ping the server.
from ping3 import ping, verbose_ping
def check_ping(host):
response = ping(host)
return response if response else False
# Testing the function
print(f"Ping response from www.google.com: {check_ping('www.google.com')} ms")
# For more verbose output
verbose_ping("www.google.com")
In our check_ping
function, we leverage ping3.ping()
to send an ICMP packet to the provided host. This function returns the round-trip time (RTT) in seconds, or None
if the host is unreachable or the request times out.
We handle this by returning the RTT value directly when it’s available or False
when the response is None
, thus providing a clear indication of the host’s reachability or the lack thereof.
Additionally, we use ping3.verbose_ping
for detailed output, akin to the traditional ping command, which gives real-time feedback for each packet sent, enhancing the utility of our script in more interactive scenarios.
Output:
Conclusion
In conclusion, pinging servers in Python can be approached through various methods, each with its own set of advantages and use cases. The subprocess.call()
method offers a cross-platform solution, adapting to the underlying operating system.
The os.system()
method provides a more direct and simpler way to execute ping commands, though it is less flexible than the subprocess.call()
method. On the other hand, the ping3.ping()
function from the ping3
module presents a more Python-centric approach, simplifying the process by abstracting the underlying details and focusing on ease of use and readability.