How to Get the First Key in Python Dictionary
- Accessing Dictionary Keys
-
Method 1: Using
next()
-
Method 2: Using
.keys()
- Method 3: Using List Comprehensions
-
Method 4: Using
dictionary.items()
- Method 5: Using Dictionary Unpacking (Python 3.7+)
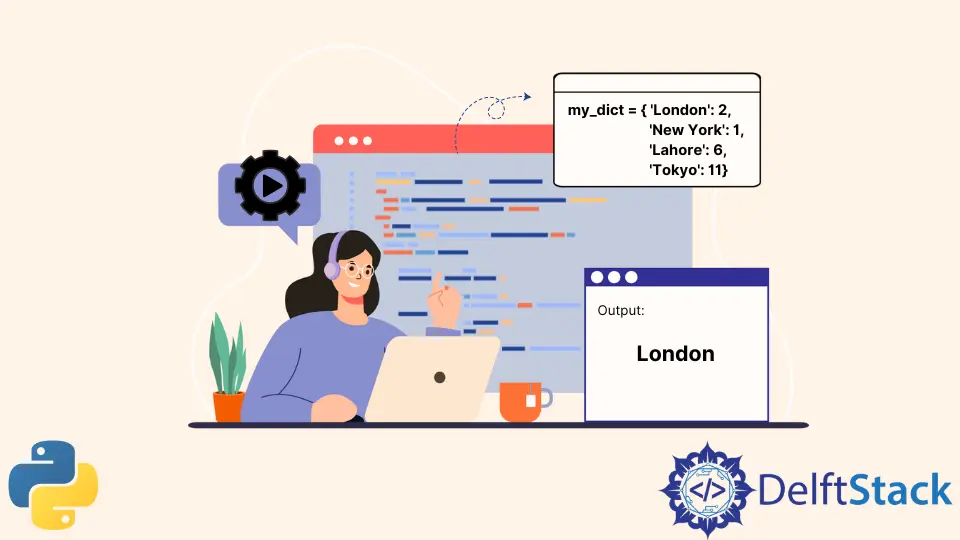
Python, a versatile and dynamic programming language, offers a wide range of data structures to help developers efficiently manage and manipulate data. Among these, dictionaries stand out as a key-value store that allows you to organize data in an unordered, associative manner. Dictionaries play a pivotal role in many Python applications, as they provide quick and efficient data retrieval based on keys.
In Python, dictionaries are known for their flexibility, enabling developers to work with a variety of data types and structures. However, there are times when you need to access the first key in a dictionary, either to perform specific operations or to extract critical information.
This article delves into the intricacies of obtaining the first key of a dictionary in Python. We will explore multiple methods and techniques that cater to different scenarios and versions of Python. Whether you’re a novice programmer or an experienced developer, understanding these methods will enhance your ability to work with dictionaries effectively.
Accessing Dictionary Keys
Accessing dictionary keys is a fundamental operation when working with dictionaries in Python. The key you want to access might be the first one, a specific key, or any key based on your requirements. In this section, we will explore various methods for accessing dictionary keys, including techniques using loops and built-in functions.
Basic Dictionary Key Access
In Python, you can access dictionary keys using square brackets []
. To retrieve the value associated with a particular key, simply provide that key inside the square brackets. Let’s take a look at an example:
# Creating a dictionary
my_dict = {"name": "Alice", "age": 30, "city": "New York"}
# Accessing a specific key
name = my_dict["name"]
print(name)
Using the keys()
Method
Python dictionaries come with a built-in keys()
method that returns a view object containing all the keys in the dictionary. You can convert this view object to a list to work with the keys directly. To access the first key, you can use indexing after converting to a list:
# Creating a dictionary
my_dict = {"name": "Alice", "age": 30, "city": "New York"}
# Converting keys to a list and accessing the first key
key_list = list(my_dict.keys())
first_key = key_list[0]
print(first_key)
Iterating Through Keys
Another common approach to accessing dictionary keys is by iterating through them using a loop, such as a for
loop. This technique allows you to perform actions on each key, including accessing the first key.
# Creating a dictionary
my_dict = {"name": "Alice", "age": 30, "city": "New York"}
# Iterating through keys and accessing the first key
for key in my_dict:
first_key = key
break # Exit the loop after the first iteration
print(first_key) # Output: 'name'
The Importance of Ordering
Starting from Python 3.7 and later versions, dictionaries maintain the order of key-value pairs by default. It means that as you iterate through a dictionary, you will encounter the keys in the sequence they were originally added.
However, in earlier Python versions (before 3.7), dictionaries were unordered, and you couldn’t rely on the order of keys. If you’re working with an older Python version and need to access the first key, you may need to use a different approach, such as converting keys to a list and indexing, as shown earlier.
Understanding how dictionaries handle ordering in your Python version is crucial when working with dictionary keys, especially when accessing the first key. In modern Python versions, you can confidently access the first key using techniques like the ones demonstrated here.
Method 1: Using next()
In Python, you can efficiently access the first key of a dictionary using the built-in next()
function. The next()
function allows you to iterate through elements of an iterable one at a time and retrieve the next item. When applied to a dictionary’s keys, it returns the first key without the need for loops or additional conversions.
How next()
Works
The next()
function takes two arguments: an iterable and an optional default value. It returns the next item from the iterable. If there are no more items in the iterable, it raises the StopIteration
exception. To prevent the exception, you can provide a default value that next()
will return when the iterable is exhausted.
Accessing the First Key
To access the first key of a dictionary using next()
, you can pass the dictionary’s keys view as the iterable to the next()
function. Here’s a step-by-step breakdown:
- Get the keys view of the dictionary using
my_dict.keys()
. - Pass the keys view to the
iter()
function to create an iterator. - Use
next()
to retrieve the first key from the iterator.
Let’s see this in action with an example:
# Creating a dictionary
my_dict = {"name": "Alice", "age": 30, "city": "New York"}
# Accessing the first key using next()
first_key = next(iter(my_dict.keys()))
# Printing the first key
print(first_key) # Output: 'name'
In this example, we first obtain the keys view of my_dict
using my_dict.keys()
. We then create an iterator from the keys view using iter()
. Finally, we use next()
to retrieve the first key from the iterator.
Method 2: Using .keys()
In Python, dictionaries provide a convenient method called .keys()
to access their keys. This method returns a view object that displays a list of all the keys in the dictionary. You can then convert this view object to a list and access the first key. Here’s how it works:
Understanding the .keys()
Method
The .keys()
method is a built-in function for dictionaries that returns a view object representing the dictionary’s keys. It provides a dynamic view of the keys, meaning it reflects any changes made to the dictionary in real time. This view object can be converted to a list to access all the keys as elements.
Accessing the First Key
To access the first key of a dictionary using the .keys()
method, follow these steps:
- Call
.keys()
on the dictionary to obtain a keys’ view. - Convert the keys view to a list.
- Access the first element of the list, which corresponds to the first key.
Here’s an example:
# Creating a dictionary
my_dict = {"name": "Bob", "age": 25, "city": "Paris"}
# Accessing the first key using .keys() and list conversion
keys_list = list(my_dict.keys())
first_key = keys_list[0]
# Printing the first key
print(first_key)
In this example, we first use .keys()
to obtain a keys’ view, and then we convert it to a list using list()
. Finally, we access the first element of the list to get the first key of my_dict
.
The .keys()
method is useful when you need to access not only the first key but also all the keys in a dictionary. This method provides an easy way to iterate over the dictionary’s keys, which can be valuable for tasks like data manipulation, filtering, or generating reports based on dictionary contents. It also allows you to maintain the order of keys, which is a feature introduced in Python 3.7 and later.
Method 3: Using List Comprehensions
List comprehensions are a concise and elegant way to process elements in a sequence, and they can be applied to dictionaries as well. Using the list comprehension, you can easily access the keys of a dictionary and extract the first key. Here’s how you can do it:
Understanding List Comprehensions
List comprehensions provide a concise and readable way to create lists by applying an expression to each item in an iterable (such as a dictionary’s keys). They consist of square brackets enclosing an expression followed by a for
loop that iterates over the iterable. List comprehensions are efficient and can simplify your code.
Accessing the First Key
To access the first key of a dictionary using list comprehension, follow these steps:
- Create a list comprehension that iterates over the dictionary’s keys.
- Extract the first key within the list comprehension.
Here’s an example:
# Creating a dictionary
my_dict = {"name": "Alice", "age": 30, "city": "New York"}
# Accessing the first key using list comprehension
first_key = [key for key in my_dict.keys()][0]
# Printing the first key
print(first_key)
In this example, we use a list comprehension to iterate over the keys of my_dict
. By enclosing [key for key in my_dict.keys()]
within square brackets, we create a list containing all the keys. Finally, we access the first element of the list using [0]
to obtain the first key.
Method 4: Using dictionary.items()
When working with dictionaries in Python, the dictionary.items()
method offers a versatile approach to access keys and their corresponding values. This method returns a view object that represents (key, value) pairs from the dictionary. While it may not directly provide the first key, we can efficiently extract it using list comprehension.
Example:
To access the first key of this dictionary using the dictionary.items()
method, you can use the following code:
# Complete Example
user_info = {"name": "Alice", "age": 30, "city": "New York"}
# Extracting the First Key
first_key = next(iter(user_info.items()))[0]
# Printing the Result
print("First Key:", first_key)
Explanation:
In this code, we perform the following steps:
- We define a dictionary
user_info
containing user details with keys like ’name,’ ‘age,’ and ‘city.’ - To access the first key of this dictionary using the
dictionary.items()
method, we start by creating an iterator from the view object returned byuser_info.items()
. We useiter()
to convert the view into an iterator. - Next, we use the
next()
function to retrieve the first (key, value) tuple from the iterator. This tuple represents the first (key, value) pair in the dictionary. - Finally, we use
[0]
to extract the first element of the tuple, which is the first key. We store it in the variablefirst_key
.
This method showcases the flexibility and readability of Python when working with dictionaries, making it a valuable tool for dictionary manipulation. You can use similar techniques to access specific keys and their corresponding values from dictionaries efficiently.
Method 5: Using Dictionary Unpacking (Python 3.7+)
With the introduction of Python 3.7, a new feature called “dictionary unpacking” became available. This feature allows you to conveniently access the first key of a dictionary without the need for loops or list conversions. Here’s how you can use dictionary unpacking to achieve this:
Understanding Dictionary Unpacking
Dictionary unpacking is a Python feature that simplifies the process of working with dictionaries. It allows you to extract keys directly, making your code more concise and readable. This feature is available in Python 3.7 and later versions.
Accessing the First Key
To access the first key of a dictionary using dictionary unpacking, you can use the below example code.
# Creating a dictionary
my_dict = {"name": "Bob", "age": 25, "city": "San Francisco"}
# Accessing the first key using dictionary unpacking
first_key, *_ = my_dict
# Printing the first key
print(f"First Key: {first_key}")
IHere, we use dictionary unpacking to access the first key of the my_dict
dictionary. Let’s break down this line step by step:
first_key, *_
: This is a destructuring assignment using the*
operator. It allows us to assign the first key of the dictionary to the variablefirst_key
. The*
operator is used to capture the remaining keys (if any) and assign them to the variable_
(underscore), which is a convention in Python to indicate that the variable is not going to be used further.my_dict
: This is the dictionary we want to unpack.
After executing this line, first_key
will contain the first key of the dictionary, which, in this case, is ’name’.
Benefits of Dictionary Unpacking
Using dictionary unpacking simplifies the process of accessing keys in dictionaries, making your code more concise and Pythonic. It eliminates the need for explicit loops or list conversions, improving code readability. Additionally, dictionary unpacking is backward compatible, meaning it can be used alongside older dictionary access methods, providing versatility in your code.