Folium Package in Python
- Install Folium Package in Python
- Create a Simple Map Using Folium Package in Python
- Add Markers to a Map Using Folium Package in Python
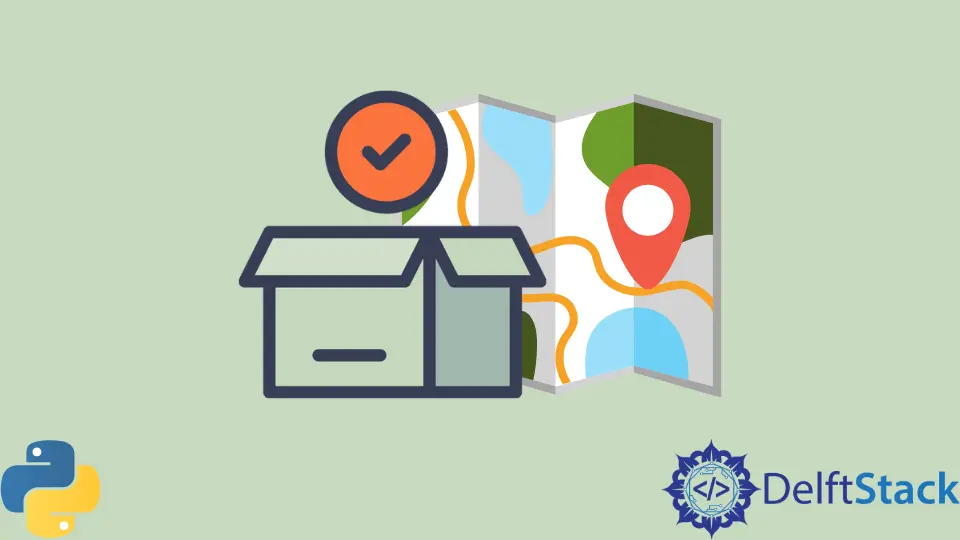
This tutorial will introduce the uses of the Python Folium library.
Folium is a library in Python that visualizes the already manipulated data in an interactive leaflet map. We can make different leaflet maps and visualize geospatial data through this library.
Install Folium Package in Python
Before we can utilize its functionalities, we should install Folium first using the following command.
#Python 3.x
pip install folium
Create a Simple Map Using Folium Package in Python
To show any location on the map, we will import the folium library first in our code. Then we will call the Map()
method of Folium and pass the location in terms of latitude
and longitude
.
We can obtain the latitude and longitude of any location from Google. The other argument that we have passed in the following code is zoom_start
, which initially specifies the map’s zoom level.
To display the map on the screen, we will write the map object name, my_map
in our case.
# Python 3.x
import folium
my_map = folium.Map(location=[24.860966, 66.990501], zoom_start=12)
my_map
Output:
Add Markers to a Map Using Folium Package in Python
To specify or highlight the exact location on the map, we can add a marker on that point, an icon or shape representing a location. Below are the marker types that we can use.
Add a Circular Marker on a Map Using Folium Package in Python
We will use the circleMarker()
method to add a circular marker on the map and pass the location, radius, and popup text as arguments.
# Python 3.x
import folium
my_map = folium.Map(location=[24.860966, 66.990501], zoom_start=12)
folium.CircleMarker(
location=[24.860966, 66.990501], radius=40, popup="My Location"
).add_to(my_map)
my_map
Output:
Add a Parachute Marker on a Map Using Folium Package in Python
A parachute marker is the standard way to represent a location on the map. We will use the Marker()
method to add a parachute marker and pass the location and popup text arguments.
# Python 3.x
import folium
my_map = folium.Map(location=[24.860966, 66.990501], zoom_start=15)
folium.Marker([24.860966, 66.990501], popup="My Place").add_to(my_map)
my_map
Output:
Add a Line Between Two Locations on a Map Using Folium Package in Python
If we want to add a line between two locations on the map, we will create two markers, each having their location and popup text specified. Then we will call the PolyLine()
method of Folium and pass both locations and line opacity or thickness.
Additionally, if you’re interested in further calculations related to locations, consider exploring how to calculate the distance between two GPS points with Python. You can find more details in this article about Calculating the Distance Between Two GPS Points.
We will see both the locations on a map and a line that connects them in the output. This is helpful when we want to see how far apart the two places are from each other.
# Python 3.x
import folium
my_map = folium.Map(location=[24.860966, 66.990501], zoom_start=12)
folium.Marker([24.860966, 66.990501], popup="Location 1").add_to(my_map)
folium.Marker([24.7077, 66.0501], popup="Location 2").add_to(my_map)
folium.PolyLine(
locations=[(24.860966, 66.990501), (24.7077, 66.0501)], line_opacity=0.5
).add_to(my_map)
my_map
Output:
I am Fariba Laiq from Pakistan. An android app developer, technical content writer, and coding instructor. Writing has always been one of my passions. I love to learn, implement and convey my knowledge to others.
LinkedIn