How to Calculate the Distance Between Two GPS Points in Python
- Calculate the Distance Between Two GPS Points Using the Haversine Formula in Python
-
Calculate the Distance Between Two GPS Points Using the
mpu
Library in Python -
Calculate the Distance Between Two GPS Points Using the
geopy
Library in Python - Conclusion
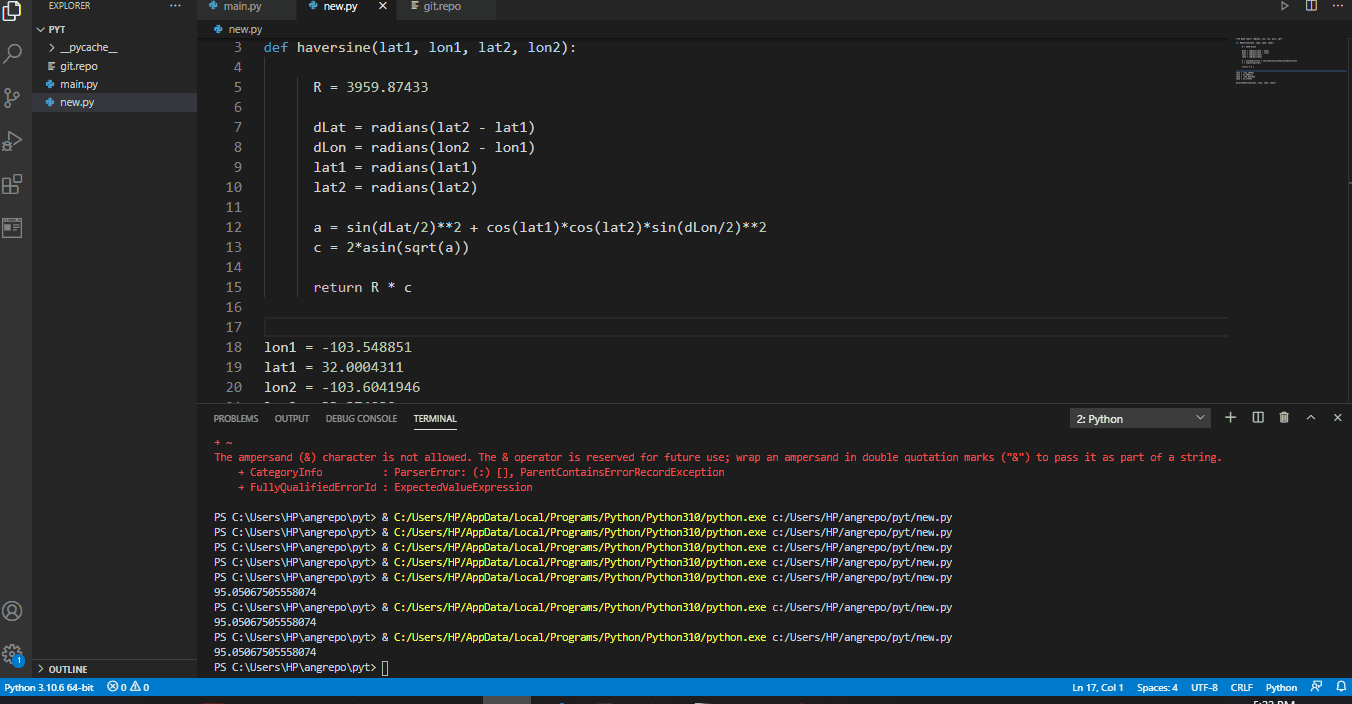
Calculating the distance between two GPS points is a geographical and mathematical exercise that we can operate inside the Python framework. Let us now look at how we can perform this operation with Python.
Calculate the Distance Between Two GPS Points Using the Haversine Formula in Python
The haversine formula is a simplified way to calculate the distance between two GPS points with Python, but its calculation is based on the assumption that the Earth is a perfect sphere. However, the Earth isn’t a perfect sphere.
This means that the result we’ll obtain would not be perfectly accurate; there’s a slight margin of error due to the assumption. The haversine formula calculates the distance between two GPS points by calculating the distance between two pairs of longitude and latitude.
Create a Python and input these codes inside. Name the file new.py
as seen below:
Code Snippet- new.py
:
from math import radians, cos, sin, asin, sqrt
def haversine(lat1, lon1, lat2, lon2):
R = 3959.87433
dLat = radians(lat2 - lat1)
dLon = radians(lon2 - lon1)
lat1 = radians(lat1)
lat2 = radians(lat2)
a = sin(dLat / 2) ** 2 + cos(lat1) * cos(lat2) * sin(dLon / 2) ** 2
c = 2 * asin(sqrt(a))
return R * c
lon1 = -103.548851
lat1 = 32.0004311
lon2 = -103.6041946
lat2 = 33.374939
print(haversine(lat1, lon1, lat2, lon2))
Output:
When we click on Run, we should see this result inside the terminal.
Calculate the Distance Between Two GPS Points Using the mpu
Library in Python
The mpu
is derived from Martins Python Utilities, and it is a Python library that can be used to perform various functions without needing any other dependencies.
First, we install mpu
by typing the following:
pip install mpu
Then, we create a new Python file, name it new.py
, and type in the codes below:
Code Snippet- new.py
:
import mpu
lat1 = 32.0004311
lon1 = -103.548851
lat2 = 33.374939
lon2 = -103.6041946
dist = mpu.haversine_distance((lat1, lon1), (lat2, lon2))
print(dist)
Wait a few moments, and you should view the distance calculated inside the terminal.
Output:
Calculate the Distance Between Two GPS Points Using the geopy
Library in Python
geopy
for Python is a library specifically designed for calculating distances between countries, states, cities, etc. As we will observe in the following example, we can calculate the distances using kilometers, miles, etc.
Inside the terminal, type the following to install the geopy
library:
pip install geopy
Create a new file, name it new.py
, and type in these codes.
from geopy.distance import geodesic
origin = (30.172705, 31.526725)
dist = (30.288281, 31.732326)
print(geodesic(origin, dist).kilometers)
Output:
If you want the result displayed in miles, change kilometers
in the last bit of code to miles
and run the code.
Conclusion
Calculating the distance between two specific points on Earth couldn’t be any easier. Even though the haversine formula can be off by about 0.5%, the result is still close and usable for various projects.
Fisayo is a tech expert and enthusiast who loves to solve problems, seek new challenges and aim to spread the knowledge of what she has learned across the globe.
LinkedIn