How to Enumerate Dictionary in Python
- Using a Simple For Loop to Enumerate Python Dictionary
-
Using
items()
Method to Enumerate Python Dictionary -
Using
keys()
Method to Enumerate Python Dictionary -
Using
values()
Method to Enumerate Python Dictionary -
Using
enumerate()
withitems()
to Enumerate Python Dictionary - Conclusion
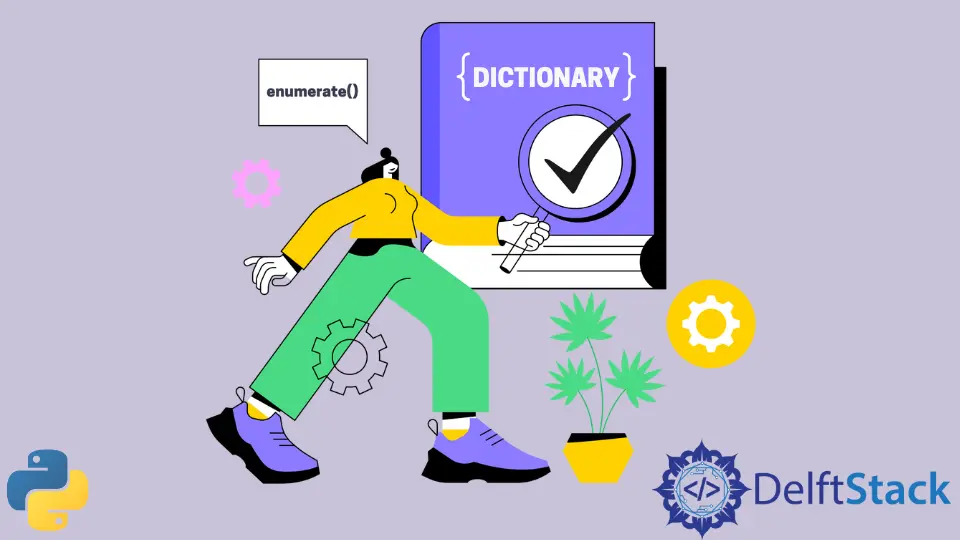
Python dictionaries serve as indispensable data structures, allowing developers to organize and manipulate key-value pairs efficiently. However, navigating the contents of a dictionary becomes more nuanced when the need arises to enumerate through its elements. Enumeration provides a systematic way to iterate over keys and values, offering improved code readability and flexibility.
In this article, we’ll explore various methods of enumerating dictionaries in Python, unraveling the intricacies of each approach.
Using a Simple For Loop to Enumerate Python Dictionary
Let’s kick off with the most straightforward method—using a simple for
loop. This approach involves iterating through the keys of the dictionary and accessing the corresponding values. Consider the following example:
# Define a dictionary representing the population of cities
city_population = {
"New York": 8398748,
"Los Angeles": 3990456,
"Chicago": 2705994,
"Houston": 2325502,
}
# Using a simple for loop
for city in city_population:
print(city, city_population[city])
Output:
New York 8398748
Los Angeles 3990456
Chicago 2705994
Houston 2325502
Explanation: In this snippet, the for
loop iterates through the keys of the city_population
dictionary. Inside the loop, we print each city name along with its population by accessing the value using the key. While this method is straightforward, it may be less concise than more advanced techniques.
Using items()
Method to Enumerate Python Dictionary
Now, let’s delve into a more Pythonic approach using the items()
method, which allows simultaneous iteration over keys and values.
# Using items() method
for city, population in city_population.items():
print(city, population)
Output:
New York 8398748
Los Angeles 3990456
Chicago 2705994
Houston 2325502
Explanation: Here, the items()
method returns a view of the dictionary’s key-value pairs. The loop then unpacks these pairs into city
and population
variables, resulting in a cleaner and more readable code structure.
Syntax of the items()
Method
Syntax:
dictionary.items()
This method returns a view object that displays a list of a dictionary’s key-value tuple pairs. Each item in this view is a tuple, where the first element is the key and the second element is the value.
Using keys()
Method to Enumerate Python Dictionary
For situations where you specifically need to iterate over keys, the keys()
method can be employed.
# Using keys() method
for city in city_population.keys():
print(city, city_population[city])
Output:
New York 8398748
Los Angeles 3990456
Chicago 2705994
Houston 2325502
Explanation: In this snippet, the keys()
method provides an iterable containing the dictionary keys. The loop then iterates through these keys, and, similar to the simple for loop, we access the corresponding values.
Using values()
Method to Enumerate Python Dictionary
Conversely, if you only need to work with the values, the values()
method is the go-to choice.
# Using values() method
for population in city_population.values():
print(population)
Output:
8398748
3990456
2705994
2325502
Explanation: Here, the values()
method returns an iterable containing the dictionary values. The loop then iterates through these values, providing a concise way to focus solely on the data.
Using enumerate()
with items()
to Enumerate Python Dictionary
To add an index to our enumeration, we can leverage the enumerate()
function with the items()
method.
# Using enumerate() with items()
for index, (city, population) in enumerate(city_population.items(), start=1):
print(index, city, population)
Output:
1 New York 8398748
2 Los Angeles 3990456
3 Chicago 2705994
4 Houston 2325502
Explanation: In this example, enumerate()
adds an index (starting from 1) to the iteration, enhancing the organization of the data. The loop then unpacks the key-value pairs, providing access to the index, city name, and population in each iteration.
Syntax of the enumerate()
Function
enumerate(iterable, start=0)
- Parameters :
iterable
: The sequence to be enumerated.start
: The starting index of the counter. By default, it is 0.
enumerate()
is a built-in function that adds a counter to an iterable (like a list or a tuple) and returns it in a form of enumerating object. This object can then be used directly in for loops.
Invoking enumerate()
with a Specific Start Value
For scenarios where a custom starting index is desired, the enumerate()
function allows specifying a start value.
# Invoking enumerate() with a specific start value
for index, (city, population) in enumerate(city_population.items(), start=10):
print(index, city, population)
Output:
10 New York 8398748
11 Los Angeles 3990456
12 Chicago 2705994
13 Houston 2325502
Explanation: Here, we set the start
parameter of enumerate()
to 10. This alters the starting index of our enumeration while retaining the elegant unpacking of key-value pairs.
Conclusion
In conclusion, enumerating dictionaries in Python is a versatile and powerful concept, offering various methods tailored to different use cases. Whether you need a simple iteration, simultaneous access to keys and values, or a custom index, Python provides elegant solutions to enhance your code readability and efficiency. By mastering these techniques, you can navigate and manipulate dictionary data with finesse, contributing to the clarity and maintainability of your Python programs.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn