How to Convert Dictionary to Tuples in Python
-
Method 1: Using the
items()
Method - Method 2: Using List Comprehension
-
Method 3: Using the
map()
Function - Conclusion
- FAQ
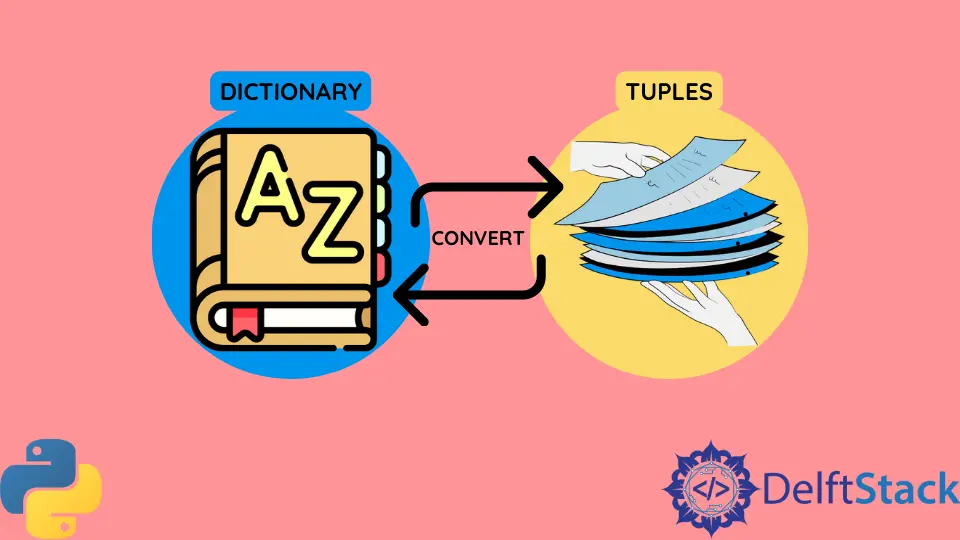
Converting a dictionary to a list of tuples in Python is a common task that can be achieved with ease. Whether you’re dealing with data manipulation, preparing data for analysis, or simply trying to understand how Python handles data structures, knowing how to perform this conversion is essential.
In this tutorial, we’ll explore various methods to convert a dictionary into tuples, highlighting the benefits and use cases for each approach. By the end of this guide, you’ll be equipped with the knowledge to efficiently convert dictionaries to tuples in your Python projects. Let’s dive in!
Method 1: Using the items()
Method
One of the simplest ways to convert a dictionary to a list of tuples is by using the items()
method. This built-in method returns a view object that displays a list of a dictionary’s key-value tuple pairs. It’s straightforward and efficient, making it a popular choice among Python developers.
Here’s how you can implement this method:
my_dict = {'a': 1, 'b': 2, 'c': 3}
tuple_list = list(my_dict.items())
print(tuple_list)
Output:
[('a', 1), ('b', 2), ('c', 3)]
Using the items()
method is not only easy but also very readable. When you call my_dict.items()
, it generates a view of the dictionary’s items, which are then easily converted into a list of tuples by wrapping it with the list()
function. This method maintains the order of the keys as they were inserted into the dictionary, which is particularly useful when the order matters in your application. Thus, if you need to convert a dictionary to tuples quickly, this is the go-to method.
Method 2: Using List Comprehension
Another efficient way to convert a dictionary to a list of tuples is by utilizing list comprehension. This method is particularly useful for those who prefer a more concise and Pythonic approach. It allows you to create a new list by iterating over the dictionary and generating tuples from its key-value pairs.
Here’s an example of how to do this:
my_dict = {'x': 10, 'y': 20, 'z': 30}
tuple_list = [(key, value) for key, value in my_dict.items()]
print(tuple_list)
Output:
[('x', 10), ('y', 20), ('z', 30)]
In this code, the list comprehension iterates through each key-value pair in the dictionary using my_dict.items()
. For each iteration, it creates a tuple containing the key and the corresponding value, which is then added to the new list. This method is not only efficient but also enhances code readability. If you have additional logic to apply while converting, list comprehension provides a flexible way to do so without compromising performance.
Method 3: Using the map()
Function
If you are looking for an alternative approach, the map()
function can also be utilized to convert a dictionary into a list of tuples. This built-in function applies a specified function to every item of an iterable, which can be quite handy in this context.
Here’s how you can employ the map()
function:
my_dict = {'name': 'Alice', 'age': 25, 'city': 'New York'}
tuple_list = list(map(lambda item: (item[0], item[1]), my_dict.items()))
print(tuple_list)
Output:
[('name', 'Alice'), ('age', 25), ('city', 'New York')]
In this example, the map()
function is used in conjunction with a lambda function that takes each item (which is a tuple of key-value pairs) and simply returns it unchanged. The result is then converted to a list. While this method may seem a bit more complex than the previous ones, it can be useful when you want to apply additional transformations to the items during the conversion process. This flexibility allows for more intricate data manipulation while still achieving the desired outcome of converting a dictionary to tuples.
Conclusion
Converting a dictionary to a list of tuples in Python is a straightforward process that can be accomplished using several methods. Whether you choose to use the items()
method, list comprehension, or the map()
function, each approach has its unique advantages. By understanding these methods, you can select the one that best fits your coding style and the specific requirements of your project. With this knowledge, you will be better equipped to handle data manipulation tasks in Python effectively.
FAQ
-
What is the purpose of converting a dictionary to tuples?
Converting a dictionary to tuples allows for easier data manipulation and can be useful in scenarios where immutable data structures are required. -
Can I convert a nested dictionary to tuples?
Yes, you can convert a nested dictionary to tuples, but you may need to implement a recursive function to handle the inner dictionaries. -
Are there performance differences between the methods?
Generally, the performance differences are minimal for small dictionaries, butitems()
is often the most efficient for straightforward conversions. -
Can I use these methods for large dictionaries?
Yes, these methods can be used for large dictionaries, but be mindful of memory usage and performance when dealing with very large datasets.
- Is there a way to convert only specific key-value pairs to tuples?
Yes, you can filter the items during the conversion process using conditional statements in list comprehensions or lambda functions.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn