How to Declare a Dictionary in Python
- Method 1: Using Curly Braces
-
Method 2: Using the
dict()
Constructor - Method 3: Using a List of Tuples
- Method 4: Dictionary Comprehension
- Conclusion
- FAQ
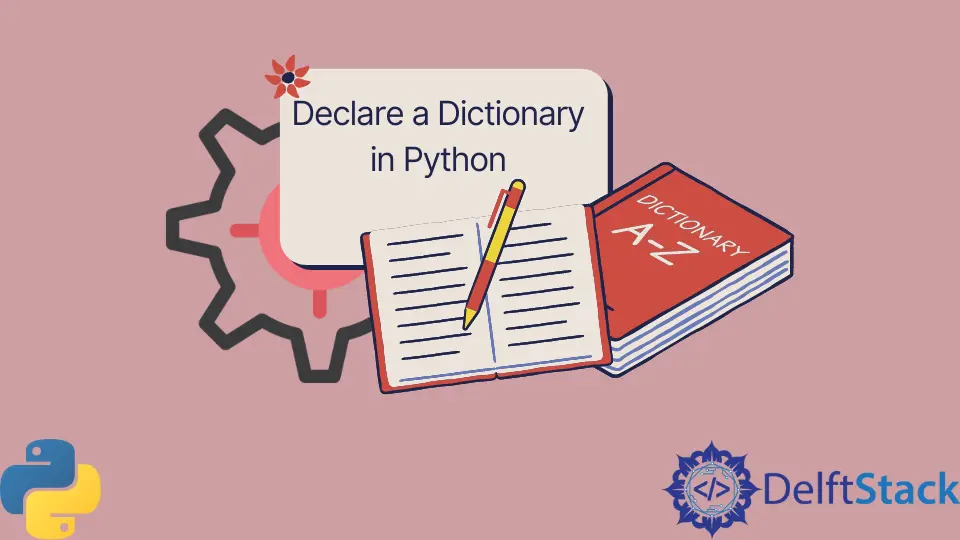
Dictionaries are one of the most versatile and powerful data types in Python. They allow you to store data in key-value pairs, making it easy to retrieve, update, and manipulate information. If you’re new to Python or looking to refine your skills, understanding how to declare a dictionary is essential.
In this tutorial, we’ll explore various methods to declare a dictionary in Python, providing clear examples to help you grasp the concept. Whether you’re working on a simple project or a complex application, mastering dictionaries will enhance your programming capabilities. Let’s dive in and discover how to effectively declare dictionaries in Python.
Method 1: Using Curly Braces
The most common way to declare a dictionary in Python is by using curly braces. This method is straightforward and intuitive. You simply create a pair of curly braces and include your key-value pairs, separated by colons. Here’s how it works:
my_dict = {
"name": "Alice",
"age": 30,
"city": "New York"
}
Output:
{'name': 'Alice', 'age': 30, 'city': 'New York'}
In this example, we created a dictionary named my_dict
that contains three key-value pairs. The keys are “name”, “age”, and “city”, while the corresponding values are “Alice”, 30, and “New York”. You can access the values by referring to their keys. For instance, my_dict["name"]
will return “Alice”. This method is not only simple but also allows for easy readability, making it a popular choice among Python developers.
Method 2: Using the dict()
Constructor
Another way to declare a dictionary in Python is by using the built-in dict()
constructor. This method is particularly useful when you want to create a dictionary from a sequence of key-value pairs or when you prefer a more functional style. Here’s how you can do it:
my_dict = dict(name="Bob", age=25, city="Los Angeles")
Output:
{'name': 'Bob', 'age': 25, 'city': 'Los Angeles'}
In this example, we utilized the dict()
constructor to create a dictionary named my_dict
. The keys are specified as keyword arguments, making the syntax clean and easy to understand. This method is especially handy when you want to create dictionaries dynamically or when working with functions that return key-value pairs. You can still access the values using their respective keys, just like with the curly braces method.
Method 3: Using a List of Tuples
If you have a list of tuples, you can also convert it into a dictionary using the dict()
constructor. This method is particularly useful when you have data in a different format and want to transform it into a dictionary. Here’s how to do it:
tuple_list = [("name", "Charlie"), ("age", 28), ("city", "Chicago")]
my_dict = dict(tuple_list)
Output:
{'name': 'Charlie', 'age': 28, 'city': 'Chicago'}
In this example, we first created a list of tuples named tuple_list
, where each tuple contains a key-value pair. By passing this list to the dict()
constructor, we transformed it into a dictionary named my_dict
. This method is particularly useful for converting data from CSV files or APIs, allowing for easy data manipulation and access. The resulting dictionary retains the same key-value structure, and you can access the values in the same way as before.
Method 4: Dictionary Comprehension
Dictionary comprehension is a concise way to create dictionaries in Python. It allows you to construct a dictionary in a single line of code, making it a powerful tool for more advanced users. This method is especially useful when you need to create dictionaries from existing data structures. Here’s an example:
my_dict = {x: x**2 for x in range(5)}
Output:
{0: 0, 1: 1, 2: 4, 3: 9, 4: 16}
In this example, we used dictionary comprehension to create a dictionary named my_dict
. The keys are integers from 0 to 4, and the values are the squares of these integers. This method is not only efficient but also enhances code readability. You can easily modify the expression to suit your needs, making it a versatile option for generating dictionaries dynamically.
Conclusion
In this tutorial, we’ve explored various methods to declare a dictionary in Python, including using curly braces, the dict()
constructor, a list of tuples, and dictionary comprehension. Each method has its own advantages, and understanding these can significantly enhance your programming skills. Whether you’re storing user data, processing information, or building complex applications, dictionaries are an essential tool in your Python toolkit. Now that you know how to declare dictionaries, you’re well on your way to becoming a more proficient Python programmer.
FAQ
-
What is a dictionary in Python?
A dictionary in Python is a mutable, unordered collection of key-value pairs, allowing for efficient data retrieval. -
Can dictionary keys be of any data type?
Yes, dictionary keys can be of any immutable data type, such as strings, numbers, or tuples.
-
How do I access a value in a dictionary?
You can access a value by using its key within square brackets, e.g.,my_dict["key"]
. -
Are dictionaries ordered in Python?
As of Python 3.7, dictionaries maintain the insertion order of items. -
How can I update a value in a dictionary?
You can update a value by assigning a new value to an existing key, e.g.,my_dict["key"] = new_value
.