How to Copy a 2D Array in Python
-
Create a Copy of 2D Arrays Using the NumPy
copy()
Function -
Create a Copy of a 2D Array Using the
copy.deepcopy()
Function - Create a Shallow Copy of a 2D Array in Python
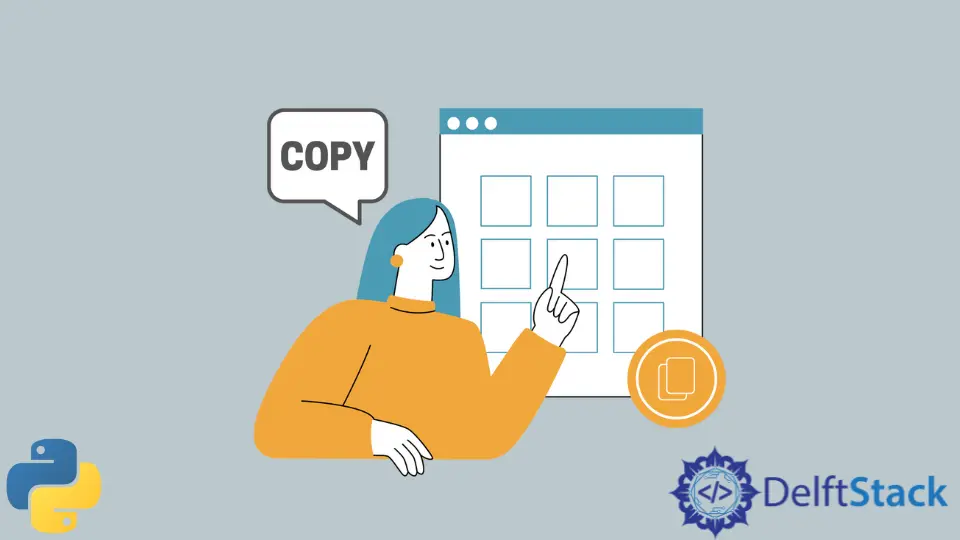
A two-dimensional array is an array of arrays representing data in rows and columns.
Elements in two-dimensional arrays can be accessed using two indices. The first index refers to the subarrays within the array, and the second index refers to the individual elements.
Two-dimensional arrays follow the following syntax.
array_name = [[d1, d2, d3, d4, ..., dn], [c1, c2, c3, c4, ...., cn]]
The first subarray represents the row, while the second subarray represents the table’s column.
Making copies of objects is an important aspect of working and modifying arrays.
This allows us to freely iterate and modify objects such as arrays without encountering errors. Creating copies also allows us to retain the original objects and instead perform operations on the copies.
Python offers a range of factory functions that can be used to create a copy of an array or any other mutable object in Python. These mutable objects include dictionaries, sets, and lists.
Create a Copy of 2D Arrays Using the NumPy copy()
Function
NumPy offers the copy()
function. The copy()
function can be implemented as shown below.
import numpy as np
x = np.array([[23, 34, 45], [24, 45, 78]])
y = x.copy()
print(y)
# making changes to the copy of array
y[0][2] = 65
print(y)
# printing original 2d array
print(x)
Output:
[[23 34 45]
[24 45 78]]
[[23 34 65]
[24 45 78]]
[[23 34 45]
[24 45 78]]
In the example code, we created a copy of the original array and changed the copy. However, we have retained the original copy of the array, printed in the last line.
Create a Copy of a 2D Array Using the copy.deepcopy()
Function
Using the deep copy function, we can create a new compound object by recursively adding the objects from the original object into the new object.
This means that changes made to the new object, which is an array, will not affect the original array. We will use the deepcopy()
function available in the copy module to implement this method.
The copy module provides functions for copying elements from Python objects such as lists and arrays. The deepcopy()
function can be used to create a copy of the 2D array, as shown in the example below.
import copy
x = [[24, 23, 25], [32, 43, 54]]
y = copy.deepcopy(x)
# a copy of the original array
print(y)
# making changes to the copy of the array
y[0][2] = 100
print(y)
# original array
print(x)
Output:
[[24, 23, 25], [32, 43, 54]]
[[24, 23, 100], [32, 43, 54]]
[[24, 23, 25], [32, 43, 54]]
Create a Shallow Copy of a 2D Array in Python
Similarly, the shallow copy method also creates a compound object representing the original object. Unlike deep copy, which inserts copies of the original objects, shallow copying only references objects in the original object.
Therefore shallow copying does not recurse but only allows us to copy the reference of one object into a different object. This means that changes in one object will not affect the other object.
As shown below, shallow copying can be implemented using the view()
function.
import numpy as np
x = np.array([[24, 23, 25], [32, 43, 54]])
y = x.view()
# a copy of the original array
# making changes to the copy of the array
y[0][2] = 100
print(y)
# original array
print(x)
Output:
[[ 24 23 100]
[ 32 43 54]]
[[ 24 23 100]
[ 32 43 54]]
In this case, changing the value of one array changes the values in the original array. However, the two arrays still reference different objects.
Using the id()
function, we can verify that the two arrays reference different objects. This is a built-in function that returns the identity of objects and can be implemented as shown below.
import numpy as np
x = np.array([[24, 23, 25], [32, 43, 54]])
print(id(x))
y = x.view()
print(id(y))
# a copy of the original array
# making changes to the copy of the array
y[0][2] = 100
print(y)
# original array
print(x)
Output:
139636169252912
139636169253008
[[ 24 23 100]
[ 32 43 54]]
[[ 24 23 100]
[ 32 43 54]]
Alternatively, we can also create a shallow copy of a 2D array using the copy()
function.
The copy module provides the copy()
function that provides different functions for copying elements in lists, arrays, and other objects. As shown below, the copy
function can be implemented to create a shallow copy.
import numpy as np
import copy
x = np.array([[24, 23, 25], [32, 43, 54]])
y = copy.copy(x)
# a copy of the original array
# making changes to the copy of the array
y[0][2] = 100
print(y)
# original array
print(x)
Output:
[[ 24 23 100]
[ 32 43 54]]
[[24 23 25]
[32 43 54]]
Isaac Tony is a professional software developer and technical writer fascinated by Tech and productivity. He helps large technical organizations communicate their message clearly through writing.
LinkedIn