How to Add Character to String in Python
-
Add a Character to a String in Python Using the
+
Operator -
Add a Character to a String in Python Using the
join()
Method -
Add a Character to a String in Python Using the
str.format()
Method - Add a Character to a String in Python Using String Interpolation (f-strings)
-
Add a Character to a String in Python Using the
%
Operator - Conclusion
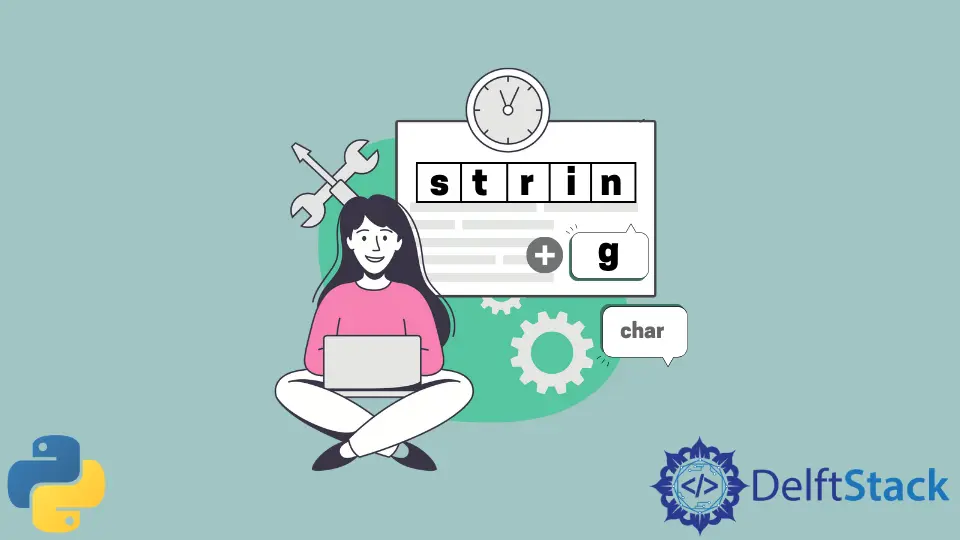
Strings are essential in Python programming, and you often need to manipulate them by adding characters or substrings.
In this article, we’ll explore various methods to add a character to a string in Python.
Add a Character to a String in Python Using the +
Operator
In Python, the +
operator is used for string concatenation. It allows you to join two or more strings together to create a new string.
When you use +
to combine strings, you effectively add the characters from one string to another.
To add a character to a string using the +
operator, follow these steps:
Start by defining the original string to which you want to add a character. This can be done by assigning a string to a variable.
original_string = "Hello, "
Next, create a variable that holds the character you want to add to the original string.
char_to_add = "W"
Then, use the +
operator to concatenate the original string and the character you want to add. This creates a new string with the character included.
new_string = original_string + char_to_add
In this example, the new_string
variable will contain "Hello, W"
.
You can then print or use the new_string
in your program as needed.
print(new_string)
Let’s look at a few practical examples of adding characters to strings using the +
operator.
Example 1: Greeting Message
greeting = "Hello, "
name = "Alice"
full_greeting = greeting + name
print(full_greeting)
Output:
Hello, Alice
In this example, greeting
is assigned the string "Hello, "
and name
is assigned the string "Alice"
. Next, full_greeting
is created by concatenating the greeting
and name
strings using the +
operator.
The print()
function is used to display the full_greeting
on the console. As a result, you get the output "Hello, Alice"
, which is a complete greeting message.
Example 2: Combining Words
word1 = "Python"
word2 = "is"
word3 = "awesome!"
sentence = word1 + " " + word2 + " " + word3
print(sentence)
Output:
Python is awesome!
In this example, three variables, word1
, word2
, and word3
, are assigned strings representing individual words. sentence
is constructed by concatenating these words with spaces in between using the +
operator.
Finally, the print()
function displays the sentence
, which results in the output Python is awesome!
.
Example 3: Adding Punctuation
sentence = "This is a simple sentence"
punctuation = "!"
complete_sentence = sentence + punctuation
print(complete_sentence)
Output:
This is a simple sentence!
Here, sentence
is assigned the string "This is a simple sentence"
, and punctuation
is assigned the string "!"
, representing an exclamation mark.
Then, complete_sentence
is formed by concatenating sentence
and punctuation
using the +
operator. The print()
function is used to display complete_sentence
, and the output is "This is a simple sentence!"
.
This demonstrates how you can easily add punctuation or special characters to the end of a sentence or string by using the +
operator.
Add a Character to a String in Python Using the join()
Method
Another efficient way to add a character to a string is by using the join()
method. The join()
method is a powerful tool in Python used for string manipulation.
It’s a method that operates on a string and takes an iterable as an argument, which typically consists of other strings or characters. The method joins these elements together into a single string, using the original string as a separator.
The basic syntax of the join()
method is as follows:
separator.join(iterable)
Here, separator
is the string on which you call the join()
method, and iterable
is a collection of elements (usually strings) that you want to concatenate.
To add a character to a string using the join()
method, start by defining the original string to which you want to add a character. This can be done by assigning a string to a variable.
original_string = "Hello"
Next, create a variable that holds the character you want to add to the original string.
char_to_add = ","
To use the join()
method, you need an iterable that contains the original string and the character you want to add. This iterable can be a list, tuple, or any other collection.
elements = [original_string, char_to_add]
Call the join()
method on the separator
string (in this case, char_to_add
) and pass the elements
iterable as its argument.
new_string = char_to_add.join(elements)
The new_string
variable will now contain the result of joining the elements using the char_to_add
as the separator.
You can then print or use the new_string
in your program as needed.
print(new_string)
Let’s explore some practical examples of adding characters to strings using the join()
method.
Example 1: Adding Commas to a List of Items
items = ["apple", "banana", "cherry"]
separator = ", "
result = separator.join(items)
print(result)
Output:
apple, banana, cherry
In this example, we have a list of items, and we want to add commas between them. The join()
method allows us to do this efficiently by specifying the comma and then joining the list elements.
Example 2: Building a Path
directory = "/home/user"
filename = "example.txt"
path = "/".join([directory, filename])
print(path)
Output:
/home/user/example.txt
Here, we have a directory and a filename, and we want to create a complete file path. By joining them with a forward slash using the join()
method, we create the desired path string.
Add a Character to a String in Python Using the str.format()
Method
The str.format()
method is a powerful way to create formatted strings in Python. It allows you to insert values, variables, or expressions into a string using placeholders enclosed in curly braces {}
.
These placeholders can be filled with data dynamically, making it a flexible method for string manipulation.
The basic syntax of the str.format()
method is as follows:
formatted_string = "Hello, {}!".format(variable)
In this example, the curly braces {}
serve as placeholders that will be replaced by the value of the variable
when the format()
method is called.
To add a character to a string using the str.format()
method, begin by defining the original string to which you want to add a character. This can be done by assigning a string to a variable.
original_string = "Hello"
Next, create a variable that holds the character you want to add to the original string.
char_to_add = ","
Construct the new string by using the str.format()
method on the original string. Inside the string, place a set of curly braces {}
where you want to insert the character, and then use the format()
method to specify what should replace those placeholders.
new_string = "{}{}".format(original_string, char_to_add)
The new_string
variable now contains the original string with the character added to it.
You can then print or use the new_string
in your program as needed.
print(new_string)
That’s it! You’ve successfully added a character to a string using the str.format()
method.
Let’s explore some practical examples of adding characters to strings using the str.format()
method:
Example 1: Greeting Message
greeting = "Hello, {}"
name = "Alice"
full_greeting = greeting.format(name)
print(full_greeting)
Output:
Hello, Alice
In this example, we have a greeting message with a placeholder, and we use the format()
method to insert the name into the placeholder.
Example 2: Adding Punctuation
sentence = "This is a simple sentence"
punctuation = "!"
complete_sentence = "{}{}".format(sentence, punctuation)
print(complete_sentence)
Output:
This is a simple sentence!
Here, we add an exclamation mark to the end of a sentence by using the format()
method to insert it at the appropriate position.
Example 3: Combining Words
word1 = "Python"
word2 = "is"
word3 = "awesome!"
sentence = "{} {} {}".format(word1, word2, word3)
print(sentence)
Output:
Python is awesome!
In this example, we concatenate individual words to form a complete sentence by filling in the placeholders with the words.
Add a Character to a String in Python Using String Interpolation (f-strings)
F-strings (formatted string literals) were introduced in Python 3.6. They provide a concise and readable way to embed expressions and variables within strings.
F-strings are created by prefixing a string literal with the letter f
or F
and using curly braces {}
to enclose expressions to be evaluated within the string. These expressions are replaced with their values in the resulting string.
The basic syntax of an f-string is as follows:
f"String with {variable} interpolation"
In this example, the expression inside the curly braces is evaluated and replaced with the value of the variable
.
Start by defining the original string to which you want to add a character. This can be done by assigning a string to a variable.
original_string = "Hello"
Next, create a variable that holds the character you want to add to the original string.
char_to_add = ","
Construct the new string by creating an f-string with curly braces {}
where you want to insert the character. Inside the curly braces, you can place the variable or expression that represents the character you want to add.
new_string = f"{original_string}{char_to_add}"
The new_string
variable now contains the original string with the character added to it.
You can then print or use the new_string
in your program as needed.
print(new_string)
Let’s explore some practical examples of adding characters to strings using f-strings:
Example 1: Greeting Message
greeting = "Hello, "
name = "Alice"
full_greeting = f"{greeting}{name}"
print(full_greeting)
Output:
Hello, Alice
In this example, we have a greeting message and use an f-string to insert the name
variable into the string.
Example 2: Adding Punctuation
sentence = "This is a simple sentence"
punctuation = "!"
complete_sentence = f"{sentence}{punctuation}"
print(complete_sentence)
Output:
This is a simple sentence!
Here, we add an exclamation mark to the end of a sentence using an f-string.
Example 3: Combining Words
word1 = "Python"
word2 = "is"
word3 = "awesome!"
sentence = f"{word1} {word2} {word3}"
print(sentence)
Output:
Python is awesome!
In this example, we concatenate individual words to form a complete sentence using f-strings.
Add a Character to a String in Python Using the %
Operator
The %
operator is used for string formatting and interpolation in Python. It allows you to create formatted strings by specifying placeholders within a string and then substituting those placeholders with values.
The %
operator works with a format string and a tuple (or single value) containing the values to be inserted into the string.
The basic syntax for using the %
operator is as follows:
formatted_string = "String with %s interpolation" % value
In this example, the %s
placeholder represents the location where the value
will be inserted.
To add a character to a string using the %
operator, first, define the original string to which you want to add a character. This can be done by assigning a string to a variable.
original_string = "Hello"
Next, create a variable that holds the character you want to add to the original string.
char_to_add = ","
Construct the new string by using the %
operator within the original string. Place a %s
placeholder where you want to insert the character and use the %
operator to specify the value to be substituted.
new_string = "%s%s" % (original_string, char_to_add)
The new_string
variable now contains the original string with the character added to it.
You can then print or use the new_string
in your program as needed.
print(new_string)
Let’s explore some practical examples of adding characters to strings using the %
operator:
Example 1: Greeting Message
greeting = "Hello, %s"
name = "Alice"
full_greeting = greeting % name
print(full_greeting)
Output:
Hello, Alice
In this example, we have a greeting message with a placeholder, and we use the %
operator to insert the name
into the placeholder.
Example 2: Adding Punctuation
sentence = "This is a simple sentence"
punctuation = "!"
complete_sentence = "%s%s" % (sentence, punctuation)
print(complete_sentence)
Output:
This is a simple sentence!
Here, we add an exclamation mark to the end of a sentence by using the %
operator to insert it at the appropriate position.
Example 3: Combining Words
word1 = "Python"
word2 = "is"
word3 = "awesome!"
sentence = "%s %s %s" % (word1, word2, word3)
print(sentence)
Output:
Python is awesome!
In this example, we concatenate individual words to form a complete sentence by using the %
operator to insert them into the placeholders.
While the %
operator for string formatting was widely used in the past, newer methods like f-strings or the str.format()
method provide more readability, flexibility, and functionality. The %
operator can be error-prone and lacks the versatility of newer string formatting options.
It’s considered an older and less preferred method for string manipulation in modern Python code.
Conclusion
Python offers multiple approaches to adding characters to strings, providing flexibility based on the task and personal preference. Whether through basic concatenation, string methods, or modern f-string interpolation, the ability to add characters to strings plays a vital role in text manipulation in Python.
The choice of method depends on factors like readability, performance, and the specific requirements of your task.