How to Set Height and Width of Tkinter Entry Widget
-
width
Option inEntry
Widget to Set the Width -
width
andheight
Option inplace
Geometry Method to Setwidth
andheight
of TkinterEntry
Widget -
ipadx
andipady
Options inpack
andgrid
Method to Set the Width and Height of TkinterEntry
Widget
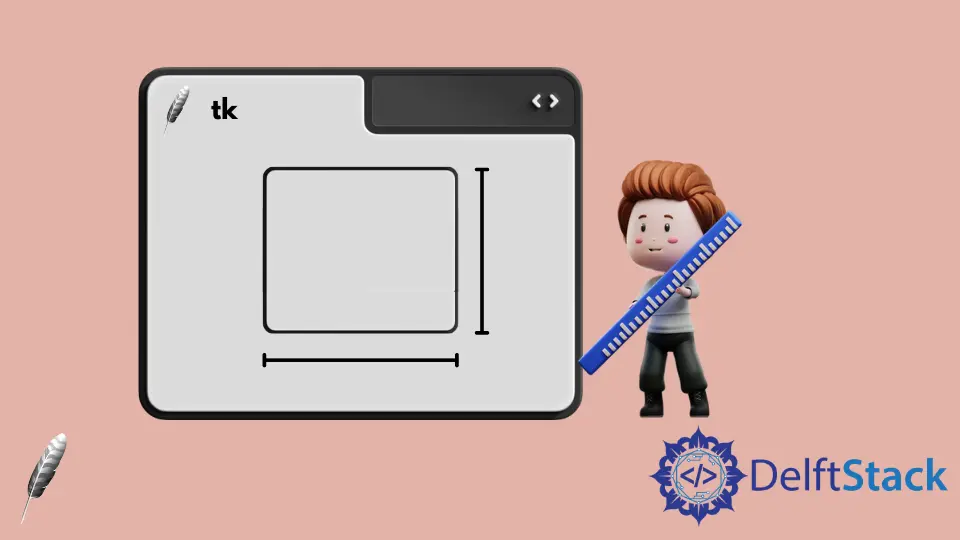
Tkinter Entry
widget is the widget to let the user enter or display a single line of text. Therefore it normally doesn’t need to set the height of the Entry
widget. But it has some methods to set the height and also the width of Tkinter Entry
widget.
width
option inEntry
widget to set the widthwidth
andheight
options inplace
method to set the width and height of TkinterEntry
widgetipadx
andipady
options inpack
andgrid
method to set the width and height of TkinterEntry
widget
width
Option in Entry
Widget to Set the Width
If only the width of Entry
widget needs to be specified, width
option in Entry
widget could be the simplest method.
import tkinter as tk
app = tk.Tk()
app.geometry("400x200")
entryExample = tk.Entry(app, width=10)
entryExample.pack(side=tk.LEFT, padx=10)
app.mainloop()
The unit of width
option in Tkinter Entry
widget is text units but not pixels. One text unit in the width is equal to the width of 0
in the system font. That’s why it could display 10 zero’s in the above image when the width
is set to be 10.
width
and height
Option in place
Geometry Method to Set width
and height
of Tkinter Entry
Widget
width
and height
in place
geometry method sets the width and height of the widget, in the unit of pixels.
import tkinter as tk
app = tk.Tk()
app.geometry("400x200")
entryExample = tk.Entry(app)
entryExample.place(x=10, y=10, width=200, height=100)
app.mainloop()
ipadx
and ipady
Options in pack
and grid
Method to Set the Width and Height of Tkinter Entry
Widget
ipadx
and ipady
sets the internal padding in the horizontal and vertical direction. It could set the width and height of Tkinter Entry
widget indirectly.
The geometry of Tkinter Entry
widget with grid
method is as below,
The actual width of the Tkinter Entry
is 2*ipadx + Default Entry width
, similarly the actual width of Entry
is 2*ipady + Default Entry height
.
import tkinter as tk
app = tk.Tk()
entryExample1 = tk.Entry(app)
entryExample2 = tk.Entry(app)
entryExample1.grid(row=0, column=0, padx=10, pady=10, ipady=30)
entryExample2.grid(row=1, column=0, padx=10, pady=10, ipadx=20, ipady=30)
app.geometry("200x200")
app.mainloop()
As you could see, because entryExample2
has ipadx
as 20
meanwhile ipadx
of entryExample1
is by default 0
, therefore, the width of entryExample2
is 40
pixels wider than entryExample1
.
We could similarly set the ipady
to change the width of Tkinter Entry
widget.
import tkinter as tk
app = tk.Tk()
entryExample1 = tk.Entry(app)
entryExample2 = tk.Entry(app)
entryExample1.grid(row=0, column=0, padx=10, pady=10, ipady=50)
entryExample2.grid(row=0, column=1, padx=10, pady=10, ipady=60)
app.geometry("300x200")
app.mainloop()
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook