How to Rotate Images in Pygame
-
Method 1: Using the
pygame.transform.rotate
Function - Method 2: Rotating with Continuous Angle Changes
- Method 3: Rotating Around the Image’s Center
- Conclusion
- FAQ
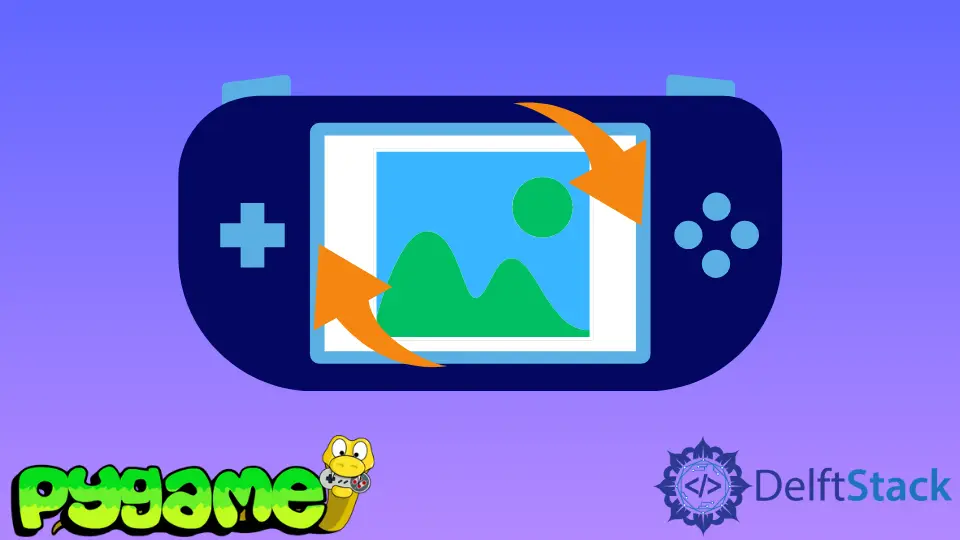
Pygame is a fantastic library for creating games and multimedia applications in Python. One common requirement when working with graphics is the ability to rotate images. Whether you’re designing a game character, a rotating background, or any other visual element, knowing how to rotate images can add a dynamic touch to your project.
In this tutorial, we’ll explore the step-by-step process of rotating images in Pygame. We’ll cover the essential methods and provide clear code examples to help you master this skill quickly. So, let’s dive in and learn how to rotate images in Pygame!
Method 1: Using the pygame.transform.rotate
Function
One of the simplest ways to rotate an image in Pygame is by using the built-in pygame.transform.rotate
function. This function takes an image and an angle as parameters and returns a new rotated surface. Here’s how you can implement it:
import pygame
import sys
pygame.init()
screen = pygame.display.set_mode((800, 600))
image = pygame.image.load('Lenna.png')
angle = 45 # Rotate by 45 degrees
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
rotated_image = pygame.transform.rotate(image, angle)
screen.fill((255, 255, 255))
screen.blit(rotated_image, (0, 0))
pygame.display.flip()
Output:
In this code, we first initialize Pygame and create a window. We load an image using pygame.image.load
and specify the rotation angle. Inside the game loop, we check for quit events and then rotate the image using pygame.transform.rotate
. The rotated image is then drawn on the screen using screen.blit
. The screen is filled with a white background before displaying the image to ensure clarity. This method is straightforward and effective for simple image rotation.
Method 2: Rotating with Continuous Angle Changes
If you want to create a dynamic effect where the image rotates continuously, you can modify the angle within the game loop. This approach can be particularly useful for animations or interactive elements in your game. Here’s an example:
import pygame
import sys
pygame.init()
screen = pygame.display.set_mode((800, 600))
image = pygame.image.load('your_image.png')
angle = 0
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
angle += 1 # Increment the angle for rotation
rotated_image = pygame.transform.rotate(image, angle)
screen.fill((255, 255, 255))
screen.blit(rotated_image, (400, 300))
pygame.display.flip()
Output:
In this example, we start with an angle of zero and increment it by one degree in each iteration of the loop. This causes the image to rotate continuously. The rest of the code remains similar to the previous method, where we handle events and update the display. The result is a smooth rotation effect that can enhance the visual appeal of your game or application.
Method 3: Rotating Around the Image’s Center
When rotating images, it’s often desirable to rotate around the image’s center rather than the top-left corner. To achieve this, you can adjust the position where the rotated image is blitted onto the screen. Here’s how to do it:
import pygame
import sys
pygame.init()
screen = pygame.display.set_mode((800, 600))
image = pygame.image.load('your_image.png')
angle = 0
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
angle += 1
rotated_image = pygame.transform.rotate(image, angle)
rect = rotated_image.get_rect(center=(400, 300)) # Center the image
screen.fill((255, 255, 255))
screen.blit(rotated_image, rect.topleft)
pygame.display.flip()
Output:
In this code, after rotating the image, we obtain a rectangle around the rotated image using get_rect()
. By setting the center of this rectangle to the desired position (in this case, (400, 300)), we ensure that the image rotates around its center. This technique is particularly useful for game elements like characters or objects that need to maintain their visual integrity during rotation.
Conclusion
Rotating images in Pygame is a straightforward process that can significantly enhance your game’s visuals. By using the pygame.transform.rotate
function, you can easily rotate images by any angle, create dynamic animations, and ensure that your images rotate around their centers. With these methods at your disposal, you can add a new layer of interactivity and excitement to your projects. So go ahead, experiment with these techniques, and let your creativity shine!
FAQ
-
How do I rotate an image by a specific angle in Pygame?
You can use thepygame.transform.rotate
function to rotate an image by a specified angle. -
Can I rotate an image continuously in Pygame?
Yes, you can increment the rotation angle in each iteration of the game loop to create a continuous rotation effect. -
How can I rotate an image around its center in Pygame?
To rotate an image around its center, useget_rect(center=(x, y))
after rotating the image to position it correctly. -
What types of images can I rotate in Pygame?
You can rotate any image format supported by Pygame, including PNG and JPEG. -
Is it possible to rotate multiple images at once in Pygame?
Yes, you can rotate multiple images by applying thepygame.transform.rotate
function to each image individually within your game loop.
Hi, my name is Maxim Maeder, I am a young programming enthusiast looking to have fun coding and teaching you some things about programming.
GitHub