How to Use the quit() Method in Pygame
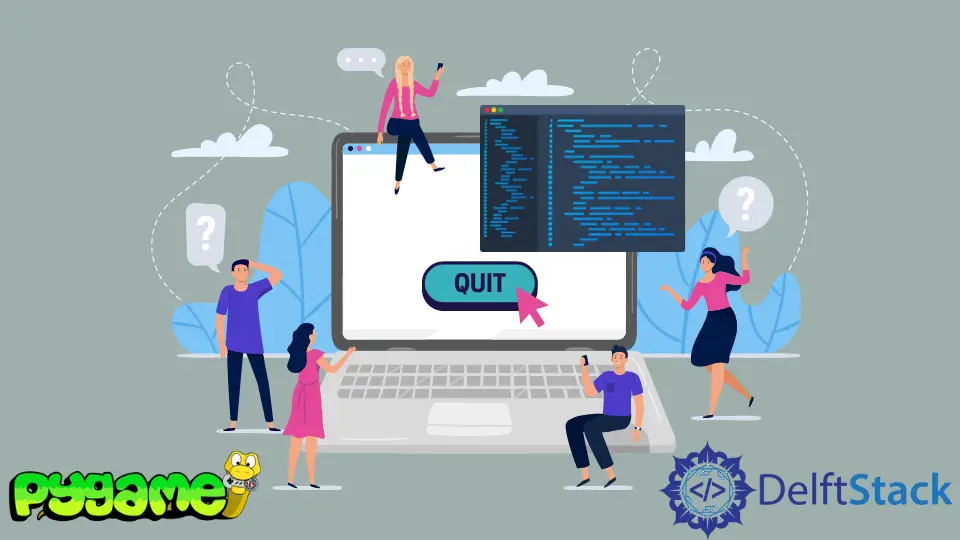
Pygame, a popular set of Python modules designed for game development, brings to the table a myriad of functionalities that simplify the creation of interactive applications.
One essential aspect of any Pygame program is the proper termination or shutdown process. Failing to close the Pygame modules gracefully can lead to resource leaks and unintended behavior.
In this article, we’ll delve into the significance of the pygame.quit()
method and explore how it ensures the orderly shutdown of a Pygame application.
Note: The code displayed here is not the complete code for a valid Pygame window. If you are interested in a bare-bones working framework, consult this article.
Use the pygame.quit()
Method
When we want to stop our game and then quit the window, we can use the pygame.quit()
method. This method will uninitialize all Pygame modules.
As the documentation states, this function will automatically be called at the end of the program, but it is safe (and advised) to call it multiple times. This method won’t stop the program, just Pygame, but we can let the program run itself out like any normal Python file.
Generally, the code structure will be like this:
import pygame
import sys
# Initialize Pygame
pygame.init()
# Your Pygame code goes here
# Quit Pygame
pygame.quit()
# Exit the program
sys.exit()
As we break down the code, it is important to always start with importing the module pygame
.
The pygame.init()
call initializes all Pygame modules. This step is crucial and must be done before using any Pygame functionalities. It sets up the necessary resources, such as display, sound, and input devices.
When it’s time to shut down the Pygame environment, the pygame.quit()
method comes into play. It uninitializes all Pygame modules, releasing resources and closing any open windows or connections. This step is crucial to prevent memory leaks and ensure a clean exit.
Following the Pygame shutdown, we call sys.exit()
to terminate the Python script. This ensures that the program exits properly, closing any open files or connections and returning control to the operating system.
Now, let’s apply some practical applications on the code:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
As you can see, this code will loop through all the events that occurred in this frame, and if the event is pygame.QUIT
, it will call the pygame.quit()
method. This event is called when the red x
at the top right of the window is pressed.
As you can notice, there is another function, sys.exit()
. This function will kill the current process; it is unnecessary, but it is often used to be sure.
Conclusion
In Pygame development, ensuring a graceful termination is as vital as the creative aspects of your game.
The pygame.quit()
method, coupled with appropriate resource cleanup, guarantees that your program exits without leaving a trail of lingering processes or memory leaks. By adopting this disciplined approach, you not only enhance the reliability of your Pygame applications but also contribute to a smoother user experience.
Hi, my name is Maxim Maeder, I am a young programming enthusiast looking to have fun coding and teaching you some things about programming.
GitHub