Pygame clock.tick()
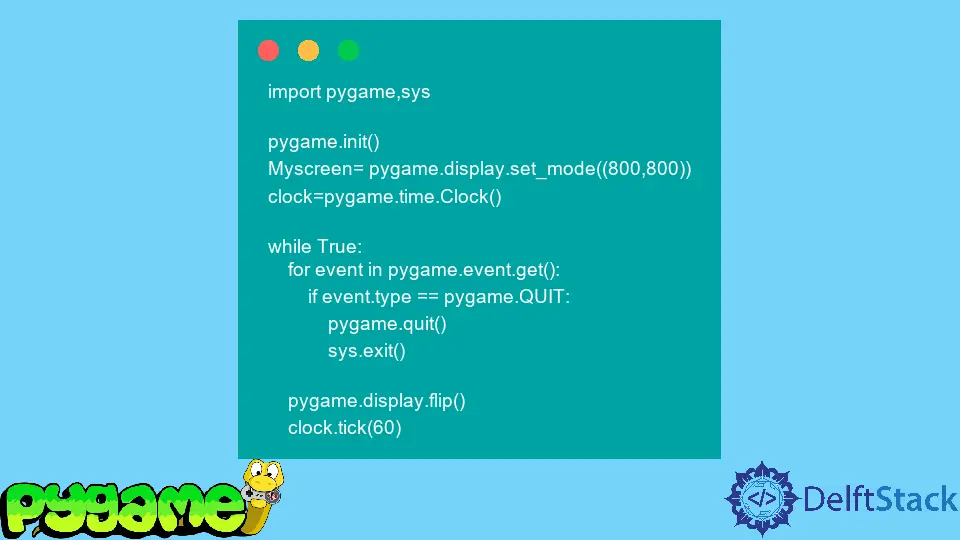
We will learn in this article about the clock.tick()
method and how it works in the pygame module. We also go to learn what is the difference between ticks and fps.
Pygame Use clock.tick()
and FPS in Game Loop
clock.tick()
vs FPS
We’re going to look at the function called clock.tick()
. When this function call this returns the number of ticks that your processor does.
If we put 150 in tick()
, this will complete 150 ticks in 0.15 seconds which will be our processor execution time.
If we move one pixel every time the loop executes, it would be faster on computers with a faster processor and slower on computers with a slower processor. This is important to know because whenever you use a clock tick, it calculates one tick equals one millisecond.
FPS stands for framerate per second, and FPS varies on GPU power. For example, if you’re playing a game with 30fps, it shows 30 frames per second, but the human eye can not see the changes.
If the game lacks during streaming, it’s because your GPU processing speed is slower.
Workflow of the clock.tick()
Method
Before we get into the actual code, let’s talk about the logic of timers because there is no inbuilt function in Pygame that waits a few seconds and then executes some code, but we had to create our timer because there is no such function.
Once we initiate pygame
, a timer starts to run and counts up in milliseconds, and we can measure how much time has passed. We need to measure a single point as often as we can and turn it into a timer.
Once we call a static point, we have to continuously measure a point, and our interrupt point will be the last or current time. The difference between our current time and our static point is the length of our timer.
If we want a two-second timer, we need a line like current-time - static-time>2000
and execute whatever code we want. Because we only measure the time on every single frame, we do not measure it every millisecond; if we have 60 frames per second, we only measure the time every 16 milliseconds.
Let’s implement all the logic in the Python script.
import pygame
import sys
pygame.init()
Myscreen = pygame.display.set_mode((800, 800))
clock = pygame.time.Clock()
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
pygame.display.flip()
clock.tick(60)
We start with importing the pygame
module, then we initiate pygame then create the main display surface object that displays a window with 800 by 800 pixels. In the next line, we create a clock using pygame.time.Clock()
to accurately measure at what time.
Then we come to our game loop and check for input if the players press the escape button. This pygame.display.flip()
draws the frame, and clock.tick(60)
measures the time accurately so that we have 60 frames per second.
To implement a timer, we create a variable called MyCurrent_Time
, which will be 0, and this variable will store the time on every frame. This variable will be used in this while loop calls pygame.time.get_ticks()
and gives us the millisecond.
Now we will create another variable called button_pressTime
and initialize it with 0, and we will check if a button was pressed key down. So we take this variable and assign it the same function pygame.time.get_ticks()
.
After pressing the down key, we want to fill our screen with white color. We will use the fill()
method and pass it RGB values in a tuple, and after two seconds, our window screen fills with black color.
Full Code Snippet:
import pygame
import sys
pygame.init()
Myscreen = pygame.display.set_mode((800, 800))
clock = pygame.time.Clock()
MyCurrent_Time = 0
button_pressTime = 0
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
if event.type == pygame.KEYDOWN:
button_pressTime = pygame.time.get_ticks()
Myscreen.fill((255, 255, 255))
MyCurrent_Time = pygame.time.get_ticks()
if MyCurrent_Time - button_pressTime > 2000:
Myscreen.fill((0, 0, 0))
print(f"current time {MyCurrent_Time} button pressed time {button_pressTime}")
pygame.display.flip()
clock.tick(60)
In the output, we can see our current time increases in every tick and static time remains 0 until we do not press the down key.
Output:
Hello! I am Salman Bin Mehmood(Baum), a software developer and I help organizations, address complex problems. My expertise lies within back-end, data science and machine learning. I am a lifelong learner, currently working on metaverse, and enrolled in a course building an AI application with python. I love solving problems and developing bug-free software for people. I write content related to python and hot Technologies.
LinkedIn