How to Draw a Circle in Pygame
- Setting Up Your Pygame Environment
- Drawing a Basic Circle
- Customizing Circle Properties
- Animating the Circle
- Conclusion
- FAQ
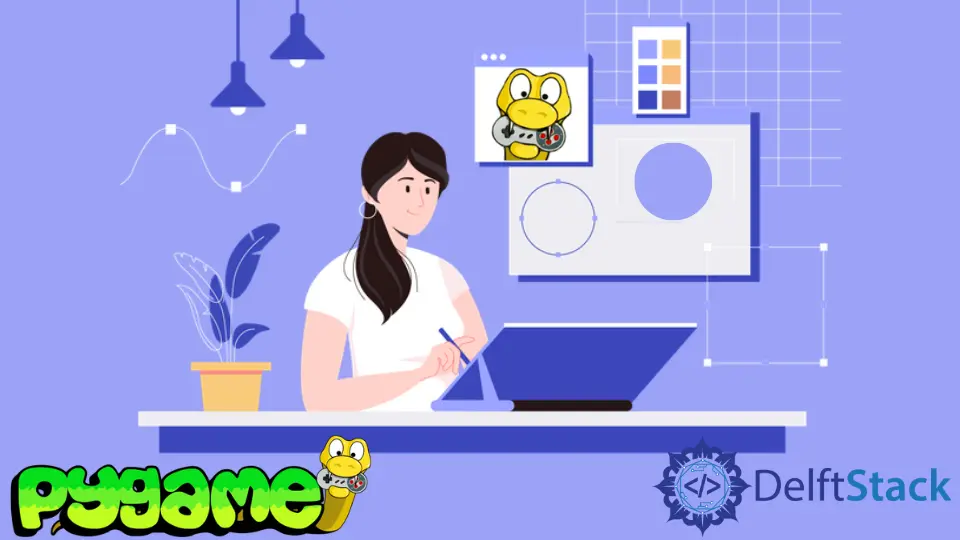
Pygame is a fantastic library for creating games and multimedia applications in Python. One of the fundamental graphical elements you’ll often need to draw is a circle. Whether you’re designing a simple game or a complex simulation, mastering how to draw a circle in Pygame is essential.
In this tutorial, we’ll walk you through the process step-by-step, covering the necessary code snippets and explanations to help you understand how it all works. By the end, you’ll have the knowledge to create beautiful circular shapes in your Pygame projects. So, let’s dive into the world of Pygame and start drawing circles!
Setting Up Your Pygame Environment
Before we begin drawing circles, make sure you have Pygame installed. If you haven’t installed it yet, you can do so using pip. Open your terminal or command prompt and run the following command:
pip install pygame
Once you have Pygame installed, you can start coding. Begin by importing the Pygame library and initializing it. Here’s a simple setup for your Pygame window:
import pygame
import sys
pygame.init()
screen = pygame.display.set_mode((800, 600))
pygame.display.set_caption("Draw a Circle in Pygame")
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
screen.fill((255, 255, 255))
pygame.display.flip()
In this code, we import the necessary libraries, set up the display window, and create an infinite loop to keep the window open until the user closes it. The screen is filled with a white background.
Output:
This setup is essential as it provides the foundation for drawing graphics, including circles.
Drawing a Basic Circle
Now that we have our Pygame environment set up, let’s draw a simple circle. To draw a circle, you’ll use the pygame.draw.circle()
function, which requires a few parameters: the surface to draw on, the color of the circle, the center position, and the radius.
Here’s a code snippet to draw a basic circle:
import pygame
import sys
pygame.init()
screen = pygame.display.set_mode((800, 600))
pygame.display.set_caption("Draw a Circle in Pygame")
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
screen.fill((255, 255, 255))
pygame.draw.circle(screen, (0, 0, 255), (400, 300), 100)
pygame.display.flip()
In this example, we’re drawing a blue circle with a center at coordinates (400, 300) and a radius of 100 pixels. The pygame.draw.circle()
function is called after filling the screen with white, ensuring that the circle is visible.
Output:
This method is straightforward and allows you to create circles quickly. You can easily modify the color, position, and size of the circle by changing the parameters.
Customizing Circle Properties
While drawing a basic circle is great, you might want to customize its properties further. Let’s explore how to change the color and add a border to the circle. The pygame.draw.circle()
function also accepts an additional parameter for the width of the circle’s border. If you set this parameter to 0, the circle will be filled; otherwise, it will be an outline.
Here’s how to customize the circle:
import pygame
import sys
pygame.init()
screen = pygame.display.set_mode((800, 600))
pygame.display.set_caption("Draw a Customized Circle in Pygame")
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
screen.fill((255, 255, 255))
pygame.draw.circle(screen, (255, 0, 0), (400, 300), 100, 5)
pygame.display.flip()
In this snippet, we’re drawing a red circle with a border width of 5 pixels. The circle is not filled, allowing the white background to show through the border.
This customization allows for more creative designs. You can experiment with different colors and border widths to achieve the desired look for your game or application.
Animating the Circle
To make your project more dynamic, you might want to animate the circle. Animation can bring life to your graphics and make your game more engaging. One way to animate a circle is by changing its position over time. Below is an example of how to make a circle move horizontally across the screen.
import pygame
import sys
pygame.init()
screen = pygame.display.set_mode((800, 600))
pygame.display.set_caption("Animate a Circle in Pygame")
x_pos = 0
y_pos = 300
speed = 5
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
screen.fill((255, 255, 255))
pygame.draw.circle(screen, (0, 255, 0), (x_pos, y_pos), 50)
x_pos += speed
if x_pos > 800:
x_pos = 0
pygame.display.flip()
In this code, we start with the circle positioned at the left edge of the window. Every frame, we increase the x_pos
variable to move the circle to the right. When the circle moves off the screen, it resets to the left side.
Output:
Animating shapes like this can be a great way to enhance the user experience in your games. You can expand on this concept by adding more shapes or varying the speed and direction of movement.
Conclusion
Drawing circles in Pygame is a fundamental skill that opens the door to more complex graphics and animations in your projects. From basic circles to customized designs and animations, the techniques shared in this tutorial will help you create visually appealing elements in your games. Remember to experiment with colors, sizes, and movements to fully explore the possibilities that Pygame offers. Happy coding!
FAQ
-
How do I change the color of the circle in Pygame?
You can change the color of the circle by modifying the color parameter in thepygame.draw.circle()
function. Use RGB values for the desired color. -
Can I draw multiple circles in Pygame?
Yes, you can draw multiple circles by calling thepygame.draw.circle()
function multiple times with different parameters for each circle. -
How can I make the circle interactive?
You can make the circle interactive by detecting mouse events. Usepygame.mouse.get_pos()
to get the mouse position and check if it overlaps with the circle’s coordinates. -
Is Pygame suitable for beginners?
Yes, Pygame is beginner-friendly and provides an excellent introduction to game development and graphics programming in Python. -
Can I use images instead of shapes in Pygame?
Absolutely! Pygame allows you to load and display images, giving you more flexibility in your graphics design.
Hi, my name is Maxim Maeder, I am a young programming enthusiast looking to have fun coding and teaching you some things about programming.
GitHub