How to Draw Rectangle in Pygame
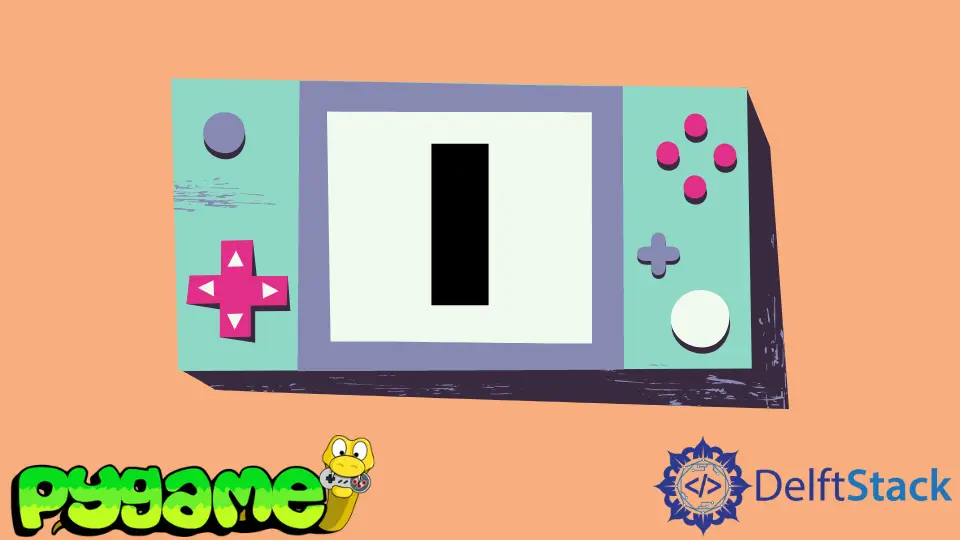
Pygame is a powerful library in Python that allows you to create engaging games and multimedia applications. Drawing shapes is one of the fundamental tasks in game development, and rectangles are often used for various purposes, such as creating game characters, obstacles, or backgrounds.
In this article, we will explore how to draw a rectangle in Pygame using the built-in function pygame.draw.rect()
. We will walk through the steps of setting up Pygame, defining a rectangle, and rendering it on the screen. Whether you’re a beginner or looking to refresh your skills, this guide will provide you with the essential knowledge to get started with drawing rectangles in Pygame.
Setting Up Pygame
Before we can dive into drawing rectangles, we need to set up our Pygame environment. This involves initializing Pygame, creating a window, and defining colors. Below is a simple example to help you get started.
import pygame
import sys
pygame.init()
screen = pygame.display.set_mode((800, 600))
pygame.display.set_caption("Draw Rectangle Example")
white = (255, 255, 255)
In this code snippet, we begin by importing the necessary modules: pygame
and sys
. After initializing Pygame with pygame.init()
, we create a window of size 800x600 pixels. The pygame.display.set_caption()
function sets the title of our window. Lastly, we define a color (white) using an RGB tuple, which we will use later to fill the background.
Output:
A Pygame window is created with the title "Draw Rectangle Example".
Drawing a Rectangle
Now that we have our Pygame window set up, let’s draw a rectangle on the screen. The pygame.draw.rect()
function allows us to specify the surface to draw on, the color of the rectangle, and the rectangle’s dimensions. Here’s how you can do it:
rect_color = (0, 128, 255)
rect_position = (100, 100, 200, 150)
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
screen.fill(white)
pygame.draw.rect(screen, rect_color, rect_position)
pygame.display.flip()
In this code, we define a rectangle color (blue) and its position and size using a tuple. The rect_position
tuple specifies the x and y coordinates of the rectangle’s top-left corner, followed by its width and height. Inside the game loop, we handle events to allow the window to close properly. The screen.fill(white)
function fills the screen with the white color, creating a clean background. Finally, pygame.draw.rect()
draws the rectangle on the screen, and pygame.display.flip()
updates the display.
Output:
A blue rectangle is drawn on a white background at position (100, 100) with a width of 200 and a height of 150.
Customizing the Rectangle
Now that you know how to draw a basic rectangle, let’s explore how to customize it further. You can change the rectangle’s size, position, and color dynamically based on user input or game logic. Here’s an example that allows you to move the rectangle with arrow keys.
rect_color = (0, 128, 255)
rect_position = [100, 100, 200, 150]
speed = 5
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
keys = pygame.key.get_pressed()
if keys[pygame.K_LEFT]:
rect_position[0] -= speed
if keys[pygame.K_RIGHT]:
rect_position[0] += speed
if keys[pygame.K_UP]:
rect_position[1] -= speed
if keys[pygame.K_DOWN]:
rect_position[1] += speed
screen.fill(white)
pygame.draw.rect(screen, rect_color, rect_position)
pygame.display.flip()
In this example, we use a list to store the rectangle’s position, allowing us to easily modify its x and y coordinates. The pygame.key.get_pressed()
function checks for key presses. If the left arrow key is pressed, we decrease the x-coordinate, moving the rectangle to the left. Similarly, we adjust the position for the right, up, and down arrow keys. This interactivity adds a fun element to your Pygame project.
Output:
The blue rectangle can now be moved around the screen using the arrow keys.
Conclusion
Drawing rectangles in Pygame is a straightforward task that can greatly enhance your game development projects. By utilizing the pygame.draw.rect()
function, you can create visually appealing elements and add interactivity to your games. Whether you’re creating simple shapes or complex graphics, mastering the basics of Pygame will set you on the path to becoming a proficient game developer. So, fire up your Pygame environment and start experimenting with rectangles today!
FAQ
-
What is Pygame?
Pygame is a Python library designed for writing video games. It provides functionalities for creating graphics, sounds, and handling user input. -
Can I draw other shapes in Pygame?
Yes, Pygame allows you to draw various shapes, including circles, lines, and polygons, using similar functions. -
How do I change the size of the rectangle?
You can modify the width and height values in the rectangle’s position tuple to change its size. -
Is Pygame suitable for beginners?
Absolutely! Pygame is beginner-friendly and offers a great way to learn game development concepts using Python. -
Can I use images instead of shapes in Pygame?
Yes, Pygame supports image loading and rendering, allowing you to use graphics instead of simple shapes.
Hi, my name is Maxim Maeder, I am a young programming enthusiast looking to have fun coding and teaching you some things about programming.
GitHub